Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial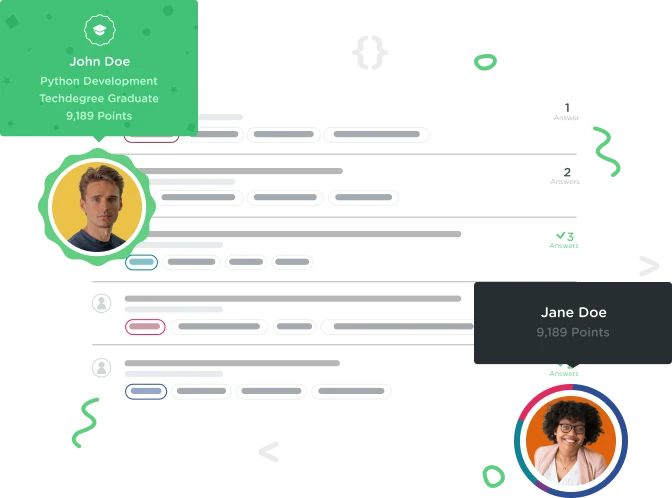
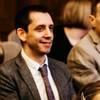
Leon Segal
14,754 Pointstry catch block not working
When I try to test if the try catch block is working, only a warning is thrown up but it doesn't stop the code running:
Output
- Connection Succeeded
Warning: PDO::query(): SQLSTATE[HY000]: General error: 1 no such table: Mediaaa in /var/www/html/integrating_php_with_databases_s1v3/inc/connection.php on line 14
- Retrieved Results
Code
<?php
// Database connection:
try {
$db = new PDO("sqlite:".__DIR__."/database.db");
$db -> setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_WARNING);
echo "- Connection Succeeded<br>";
} catch (Exception $e) {
echo "Unable to connect: ".$e->getMessage();
exit;
} // try
// Data retrieval:
try {
$results = $db -> query('SELECT * FROM Mediaaa');
echo "- Retrieved Results<br>";
} catch (Exception $e) {
echo "Foobar";
exit;
} // try
After a quick google search, I discovered this is normal for php(!).
Question
How can I test my try catch block in that case then? Do I have to write an error handler, or can I manipulate the code so that only an exception is thrown?
3 Answers
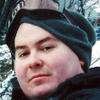
Jason Cook
11,403 PointsThere are two things you'll need to change, to resolve this issue.
- Change the ERRMODE_WARNING attribute to ERRMODE_EXCEPTION
- Add a line of code afterwards (such as my example below) that will throw an exception.
Below, you'll find a code block with a modified version that will solve your problem.
Modified Code Below
<?php
// Database connection:
try {
$db = new PDO("sqlite:".__DIR__."/database.db");
$db->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
$db->prepare('SELECT id FROM non_existing_table'); //This statement will fail, throwing exception
echo "- Connection Succeeded<br>";
} catch (Exception $e) {
echo "Unable to connect: ".$e->getMessage();
exit;
} // try
After executing this code, you should see the following output on your screen:
Unable to connect: SQLSTATE[HY000]: General error: 1 no such table: non_existing_object
I hope this helps! Happy coding :-)
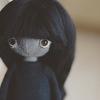
Asma Al-Marrikhi
45,525 Pointsif you use "treehouse workspaces" delete it and launch it again from last video ... it will work .. i had this problem .. and it is work for me :)
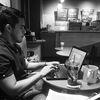
Jose Vaca Cipres
10,724 PointsWarning: PDO::query(): SQLSTATE[HY000]: General error: 1 no such table: Mediaaa in /var/www/html/integrating_php_with_databases_s1v3/inc/connection.php on line 14
This means that you don't have a table called 'Mediaaa' in your database. So, PHP can't a query correctly.
Pirvan Marian
14,609 PointsPirvan Marian
14,609 PointsChange this line of code
$db ->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_WARNING);
to
$db ->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
You have set the error mode of the PDO object to send warnings if something goes wrong, than throwing exceptions.
You can find a lot more information reading the PHP manual, http://php.net/manual/en/pdo.setattribute.php
From what we can see, we have three options for setting the error mode:
PDO::ERRMODE_SILENT: Just set error codes.
PDO::ERRMODE_WARNING: Raise E_WARNING.
PDO::ERRMODE_EXCEPTION: Throw exceptions. ( this one is used for throwing exceptions )