Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial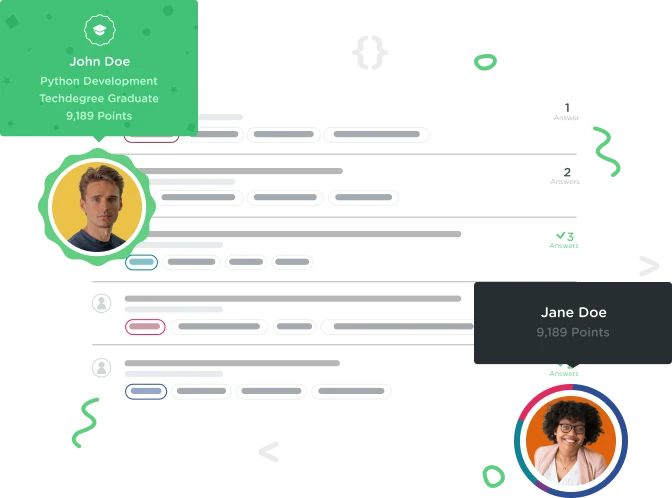
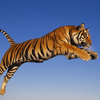
Diego Murray
2,515 PointsTry/Catch Exception Handling
If I have code that looks like this:
package com.example.java;
public class Main {
public static void main(String[] args) {
String welcome = "Welcome!";
char[] chars = welcome.toCharArray();
try {
char lastChar = chars[chars.length];
System.out.println("Last character: " + lastChar);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why would a programmer change (Exception e) to ArrayIdexOutOfBoundsExceptions? Why specify instead of having a catch all type situation?
Thanks in advance. Diego
2 Answers
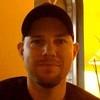
Jeremy Hill
29,567 PointsMany times programmers will add multiple catch exceptions: if you were to put one generalized exception in your code to catch everything then you wouldn't know what to tell the user/programmer when it catches an error; all you could say is "something went wrong" instead of "try using a number instead of a letter", or "couldn't read from file", etc. When you add multiple catch statements with varying messages, it will specify why the exception occurred. I hope this helps.
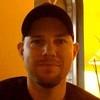
Jeremy Hill
29,567 PointsYou're welcome :)
Diego Murray
2,515 PointsDiego Murray
2,515 PointsThanks Jeremy!
Simon Coates
28,694 PointsSimon Coates
28,694 PointsGiven experience, exceptions are often pretty predictable and not always the product of faulty code. This makes recovery a more realistic option.
exceptions associated with random code defects are a bad thing. Needing to catch an expected exception is just how programming works. You don't need to code to absolutely avoid exceptions (i used to try). Sometimes, exceptions will be thrown and recovery (handling them) is okay. You can also create your own custom exceptions, rethrow exceptions or choose to handle them, or force a calling method to handle them. Exceptions and exception handling are technique.