Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial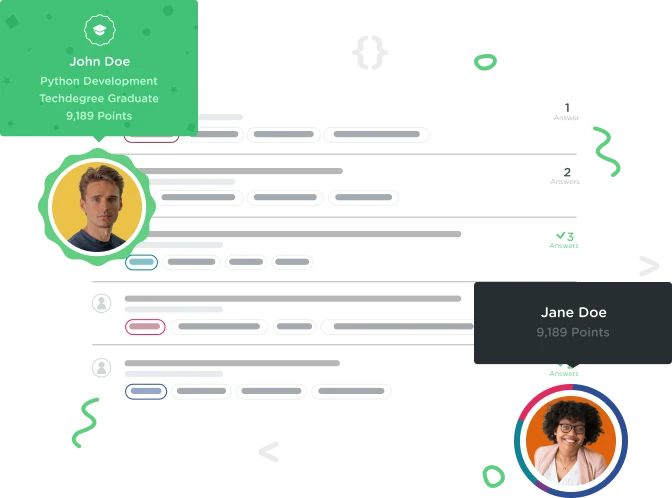
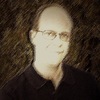
Jason Anders
Treehouse Moderator 145,860 PointsTry/Except/Else OR just Try/Except?
Craig Dennis uses a Try/Except/Else to catch the ValueError, but I did it with just the Try/Except.
Is there any benefit or real difference beside semantics to either method?
Note: I also added handling for a non integer value being passed in, just to get rid of the 'ugly' error message when that was tested.
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
print("There are {} tickets remaining!!".format(tickets_remaining))
user_name = input("Hi! Welcome... What's your name? ")
try:
number_of_tickets = input("Hi, {}. How many tickets did you want to purchase? ".format(user_name))
# Raise ValueError for non integer input
if not number_of_tickets.isdigit():
raise ValueError("That is not a number between 1 and {}".format(tickets_remaining))
number_of_tickets = int(number_of_tickets)
# Raise a ValueError if too many tickets are requested.
if number_of_tickets > tickets_remaining:
raise ValueError("There are only {} tickets remaining. Please enter a new amount.".format(tickets_remaining))
ticket_cost = number_of_tickets * 10
print("Your total ticket cost is going to be ${}".format(ticket_cost))
should_proceed = input("Is this correct? Would you like to proceed? (y/n) ")
if should_proceed.lower() == "y":
# TODO: Gather credit card info and process
print("SOLD")
tickets_remaining -= number_of_tickets
else:
print("Thanks anyways, {}!".format(user_name))
except ValueError as err:
print("Sorry, there seems there was a problem. {}.".format(err))
print("Sorry. :( All tickets are sold!")
Thanks in advance for any feedback.
Jason :)
3 Answers
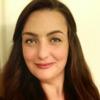
Jennifer Nordell
Treehouse TeacherHi there, Jason Anders ! Now, you'll likely still want the big dogs to chime in on this, but from what I gather, you only really need the else
clause if you also have a finally
. The else
clause states what should happen if an error wasn't raised, but the finally
clause causes the code in it to run regardless of whether an error was raised or not. But it seems to be mostly a matter of making your code more readable. But if you have code that needs to run regardless of an error, then you need the finally
. If you have code that needs to run only if there wasn't an error, you don't have to have the else
, but if you need both then the else
must come before the finally
.
You might take a look at this short article and section 8.6 of this Python documentation. Hope this helps!
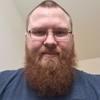
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsLike Jennifer Nordell said, the else is not required and you could just go with the try/except, but I personally prefer to use the else because it's more clear to me what's happening
try:
# try this unless something exceptional happens
except:
# if something exceptional happens then do this
else:
# if everything is okay then do this
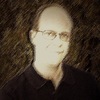
Jason Anders
Treehouse Moderator 145,860 PointsThanks Henrik!
It does read much more clear with all three like that and having the except
closer to where the exception would occur.
:)
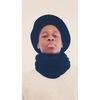
Adriano Junior
5,065 PointsThanks, Jason Anders for handling the non-integer value being passed in! I really struggled with this in a previous exercise.
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsThanks Jennifer! :)