Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial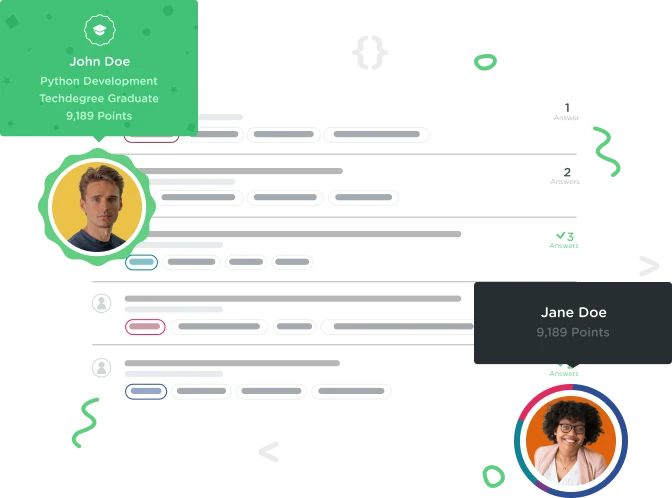
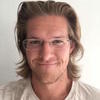
Josh Knapp
Full Stack JavaScript Techdegree Student 5,665 PointsTrying Error Handling, getting "SIGABRT" error
I'm doing a little project to help myself understand error handling a bit better. In my app there are three input fields to collect the users name. First is for the first name, second field is for the middle name and so on,
When the user presses enter, I want it to show the concatenated fullname that was entered.
I am trying error handling to make sure that the first and last name fields are populated.
When the "Enter" button is pushed, I get a "SIGABRT" error even if there is nothing in the input fields. The top of the error says "unrecognized selector sent to instance"
Here is a chunk of my view controller...
@IBAction func EnterButton() throws {
FeedbackImage.image = #imageLiteral(resourceName: "cross-out-mark")
guard FirstName.text != nil else {
FeedbackImage.image = #imageLiteral(resourceName: "cross-out-mark")
throw Errors.InvalidData("There is no first name")
}
guard LastName.text != nil else {
FeedbackImage.image = #imageLiteral(resourceName: "cross-out-mark")
throw Errors.InvalidData("There is no last name")
}
let firstName = FirstName.text!
let middleName = MiddleName.text!
let lastName = LastName.text!
let fullName = "\(firstName) \(middleName) \(lastName)"
FullName.text = fullName
}
At the top of the view controller class I created an enum for the error...
enum Errors: Error {
case InvalidData(String)
}
Can someone help me figure out what's going on??
3 Answers

jc laurent
6,351 PointsHi, Have you linked your textfield to the viewcontrollerswift?

jc laurent
6,351 Pointshave you checked in the connection inspector if you have a double linked to the textfield?
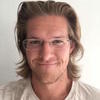
Josh Knapp
Full Stack JavaScript Techdegree Student 5,665 PointsI'm assuming you mean two links to the text field instead of a Double type. No, none of the three text fields have two links, only one to the view controller.

jc laurent
6,351 Pointsyou've been in the connection inspector haven't you?
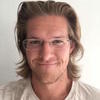
Josh Knapp
Full Stack JavaScript Techdegree Student 5,665 PointsYes, under referencing outlets, each of the elements has a connection to the view controller
Josh Knapp
Full Stack JavaScript Techdegree Student 5,665 PointsJosh Knapp
Full Stack JavaScript Techdegree Student 5,665 PointsEach of the three text fields are connected to the view controller yes