Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial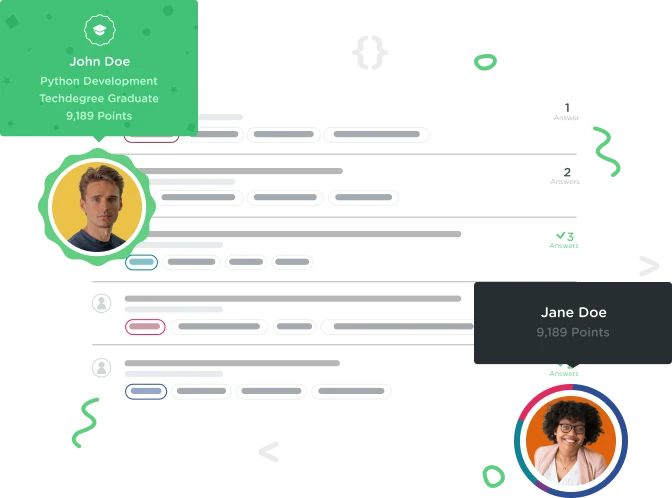
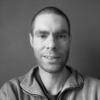
Tom Checkley
25,165 Pointstrying to add autoprefixer, not working, any advice?
I've tried to add autoprefixer (with compileSass as a dependency so it converts the sass to css and then adds prefixes) to the project we've worked on through this course. To no avail. When I tried to run gulp in terminal I get the following error
Toms-MacBook-Pro:skeletor tomcheckley$ gulp
[16:16:21] Using gulpfile /Applications/MAMP/htdocs/skeletor/gulpfile.js
[16:16:21] Starting 'clean'...
[16:16:21] Finished 'clean' after 5.43 ms
[16:16:21] Starting 'default'...
[16:16:21] Starting 'concatScripts'...
[16:16:21] Starting 'compileSass'...
[16:16:21] Finished 'default' after 14 ms
events.js:85
throw er; // Unhandled 'error' event
^
Error: ENOENT, open '/Applications/MAMP/htdocs/skeletor/js/app.js'
at Error (native)
Here is my gulpfile.js
"use strict";
var gulp = require('gulp'),
concat = require('gulp-concat'),
uglify = require('gulp-uglify'),
rename = require('gulp-rename'),
sass = require('gulp-sass'),
maps = require('gulp-sourcemaps'),
del = require('del'),
autoprefixer = require('gulp-autoprefixer');
gulp.task('concatScripts', function(){
return gulp.src([
'js/*.js'])
.pipe(maps.init())
.pipe(concat('app.js'))
.pipe(maps.write('./'))
.pipe(gulp.dest('js'));
});
gulp.task('minifyScripts', ['concatScripts'], function(){
return gulp.src('js/app.js')
.pipe(uglify())
.pipe(rename('app.min.js'))
.pipe(gulp.dest('js'));
});
gulp.task('compileSass', function(){
return gulp.src('scss/build.scss')
.pipe(maps.init())
.pipe(sass())
.pipe(maps.write('./'))
.pipe(gulp.dest('css'));
});
gulp.task('autoPrefixer', ['compileSass'], function(){
return gulp.src('css/build.css')
.pipe(maps.init())
.pipe(autoprefixer())
.pipe(maps.write('./'))
.pipe(gulp.dest('css'));
});
gulp.task('watchFiles', function(){
gulp.watch('scss/**/*.scss', ['autoPrefixer']);
gulp.watch('js/app.js', ['concatScripts']);
});
gulp.task('clean', function(){
del(['dist', 'css/build.css*', 'js/app*.js*']);
});
gulp.task("build", ['minifyScripts', 'autoPrefixer'], function(){
return gulp.src(["css/build.css",
"js/app.min.js",
'index.html',
'img/**',
'fonts/**'],
{base: './'})
.pipe(gulp.dest('dist'));
});
gulp.task('serve', ['watchFiles']);
gulp.task("default", ['clean'], function(){
gulp.start('build');
});
Thanks for any help
2 Answers
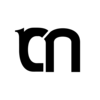
Colin Marshall
32,861 PointsYou don't need an extra task for the autoprefixer. You can just run it in your compileSass
task like this:
gulp.task('compileSass', function(){
return gulp.src('scss/build.scss')
.pipe(maps.init())
.pipe(sass())
.pipe(autoprefixer())
.pipe(maps.write('./'))
.pipe(gulp.dest('css'));
});
Bonus: Add error handling to the sass pipe so that your watch
tasks don't break on typos.
gulp.task('compileSass', function(){
return gulp.src('scss/build.scss')
.pipe(maps.init())
.pipe(sass().on('error', sass.logError))
.pipe(autoprefixer())
.pipe(maps.write('./'))
.pipe(gulp.dest('css'));
});
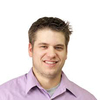
Kevin Korte
28,149 PointsI think what is happening is you're deleting app.js before you concat it.
What I'm reading for your error message is that clean runs first, and in that, you are deleting your app.js file. Than when it gets to running concatScripts after, it's looking for your app.js file, but it's not there.
I believe ENOENT means "No such file or directory", and which after it's listing your app.js file. Try changing the order your tasks run, so clean runs last, not first.
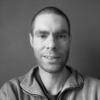
Tom Checkley
25,165 Pointsthanks Kevin, though I think from going through this course, that that is what is meant to happen, it removes the old app.js to create the new one from the concatenated files then goes through uglify. Colin's answer seems to have worked and the app.js file is being made as it should, thanks for the suggestion though
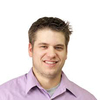
Kevin Korte
28,149 PointsSounds good, was taking a shot in the dark. I tend to use Gulp more as a personal preference. Glad Colin figured it out for you! I'll have to go through this course specifically, I think I'll learn a fair bit.
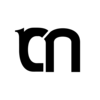
Colin Marshall
32,861 PointsThat is strange that you were getting that error and getting rid of the autoprefixer task fixed it. Maybe it was in the middle of building app.js when autoprefixer caused everything to stop so that's why an app.js error showed?
Tom Checkley
25,165 PointsTom Checkley
25,165 PointsAmazing, thanks, works like a charm