Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial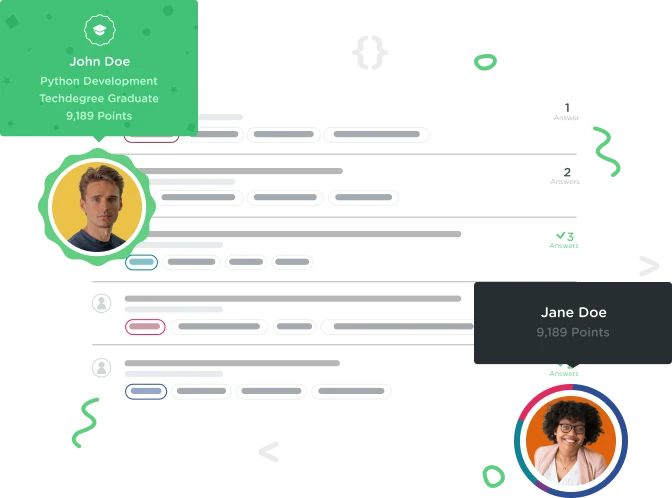
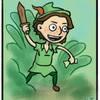
Rebecca Jensen
11,580 PointsTrying to add up digits from a number, that's from an array (JS)
I'm trying to: -access an array of numbers -take each number in the array, and add up the digits (e.g. 123 becomes 6)
This is what I have so far:
var ccNumbers = ['1200', '2111', '3111', '9111'];
function addDigits(number){
var sum = 0; //store the sum
var sumArray = [];//store several sums to print
for ( i = 0; i < ccNumbers.length; i++ ) { //loop through ccNumbers array
var ccNmbr = ccNumbers[i]; //pick out one array item and store it in ccNmbr
for ( i = 0; i < ccNmbr.length; i++ ) { //loop through each digit in ccNmbr
sum += parseInt(ccNmbr.charAt(i), 10); //add each digit to the sum
sumArray.push(sum); //add sum to an array
return sumArray;
}
}
}
addDigits();
You can also find an interactive version here: https://repl.it/I0e5/16
When I run this code, I get [ 1 ] , and that's it. What I WANT to be printing is [ 3, 5, 6, 12 ]
Any thoughts on where I'm going wrong?
2 Answers
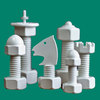
Steven Parker
231,533 PointsYou're returning too soon.
Right now, the return happens immediately after the first item is added to sumArray. But if you move the return statement to after the outer loop is done (make it be the last thing in the function) then you should get the complete result array.
You'll also need to move the push statement outside of the inner loop, so it it's only done after the calculation of sum is complete. And reset sum to 0 just before the inner loop begins.
Finally, you should use a different loop variable in each loop. Either change the name of one of them, or assign them using let.
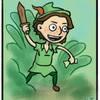
Rebecca Jensen
11,580 PointsYes! Thank you! The loop nesting was getting me.
This is the code that works now:
var ccNumbers = ['1200', '2111', '3111', '9111'];
function addDigits(number){
var sum = 0; //store the sum
var sumArray = [];//store several sums to print
for ( x = 0; x < ccNumbers.length; x++ ) { //loop through ccNumbers array
var ccNmbr = ccNumbers[x]; //pick out one array item and store it in ccNmbr
for ( y = 0; y < ccNmbr.length; y++ ) { //loop through each digit in ccNmbr
sum += parseInt(ccNmbr.charAt(y), 10); //add each digit to the sum
} //close adding digits loop
sumArray.push(sum); //add sum to an array
sum = 0; //reset sum to 0 for the next loop
} //close looping through array loop
return sumArray; //return outside of the loops
}
addDigits();
Or, interactive here: https://repl.it/I0e5/18