Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial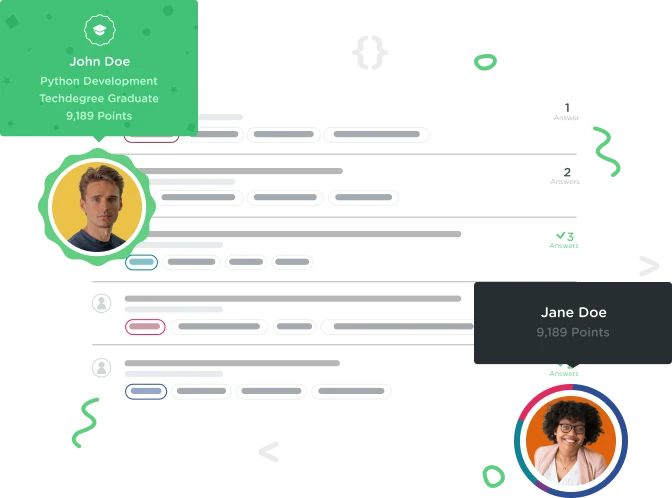

GAUTHAM RAVI PRAKASH
2,164 PointsTrying to build an app to display photos from a gallery using XML and JSON
Hi,
I am trying to develop an app that would display photos from a photo gallery with the help of XML and JSON parsing. So far, I have got the app working for display via multi-threading.
/*MainActivity-Gautham Ravi Prakash*/
package com.example.threadshw4;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
public class MainActivity extends Activity {
Button photos, slideShow;
public static final String PHOTO_ACTIVITY = "Photo Activity";
public static final int PHOTO_DISPLAY_MODE_NUMBER = 1;
public static final int SLIDE_SHOW_MODE_NUMBER = 2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
this.setTitle(R.string.MainClass_title_activity);
photos = (Button) findViewById(R.id.button1);
slideShow = (Button) findViewById(R.id.button2);
final Intent intent = new Intent(MainActivity.this, PhotoActivity.class);
photos.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View view) {
// TODO Auto-generated method stub
intent.putExtra(PHOTO_ACTIVITY, PHOTO_DISPLAY_MODE_NUMBER);
startActivity(intent);
}
});
slideShow.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
intent.putExtra(PHOTO_ACTIVITY, SLIDE_SHOW_MODE_NUMBER);
startActivity(intent);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
/*PhotoActivity-Gautham Ravi Prakash*/
package com.example.threadshw4;
import java.io.IOException;
import java.io.InputStream;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import javax.security.auth.callback.Callback;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.app.Activity;
import android.app.ProgressDialog;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.util.Log;
import android.view.Menu;
import android.view.MotionEvent;
import android.view.View;
import android.view.View.OnTouchListener;
import android.widget.ImageView;
public class PhotoActivity extends Activity {
ImageView photoImageView;
Handler hander, handlerSlideShow;
ProgressDialog progressDialog;
ExecutorService photoPool, photoPoolSlideShow;
public static final String TAG = PhotoActivity.class.getSimpleName();
public String[] urlList = null;
public int photoId = 0;
public static final int PHOTO_DISPLAY_MODE_NUMBER = 1;
public static final int SLIDE_SHOW_MODE_NUMBER = 2;
Photo photo;
PhotoSlideShow photoSlideShow;
InputStream inputStream = null;
public static final String KEY = "Image";
Message message = null;
Bitmap bitmap = null;
Bundle bundle = null;
public float x = 0;
public int a = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_photo);
this.setTitle(R.string.SecondClass_activity_photo);
photoImageView = (ImageView) findViewById(R.id.imageView1);
urlList = getResources().getStringArray(R.array.photo_urls);
photoPool = Executors.newFixedThreadPool(1);
photoPoolSlideShow = Executors.newFixedThreadPool(1);
progressDialog = new ProgressDialog(this);
progressDialog.setMessage("Loading Image...");
progressDialog.setCancelable(false);
hander = new Handler(new Handler.Callback() {
@Override
public boolean handleMessage(Message msg) {
// TODO Auto-generated method stub
bitmap = (Bitmap) msg.getData().get(KEY);
photoImageView.setImageBitmap(bitmap);
progressDialog.dismiss();
return false;
}
});
handlerSlideShow = new Handler(new Handler.Callback() {
@Override
public boolean handleMessage(Message msg) {
// TODO Auto-generated method stub
bitmap = (Bitmap) msg.getData().get(KEY);
photoImageView.setImageBitmap(bitmap);
return false;
}
});
if (getIntent().getExtras().getInt(MainActivity.PHOTO_ACTIVITY) == PHOTO_DISPLAY_MODE_NUMBER) {
getPhoto(photoId);
photoImageView.setOnTouchListener(new OnTouchListener() {
@Override
public boolean onTouch(View arg0, MotionEvent motionEvent) {
// TODO Auto-generated method stub
x = motionEvent.getX();
if (x < 0.2 * photoImageView.getWidth()) {
photoId = (photoId + urlList.length - 1)
% urlList.length;
} else if (x > 0.8 * photoImageView.getWidth()) {
photoId = (photoId + 1) % urlList.length;
}
getPhoto(photoId);
return false;
}
});
} else if (getIntent().getExtras().getInt(MainActivity.PHOTO_ACTIVITY) == SLIDE_SHOW_MODE_NUMBER) {
getPhotoSlideShow(photoId);
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.photo, menu);
return true;
}
public void getPhoto(int photoId) {
// TODO Auto-generated method stub
photo = new Photo(String.valueOf(photoId), urlList[photoId]);
photoPool.execute(photo);
progressDialog.show();
}
public void getPhotoSlideShow(int photoId) {
// TODO Auto-generated method stub
photoSlideShow = new PhotoSlideShow(photoId, urlList[photoId]);
photoPoolSlideShow.execute(photoSlideShow);
// getPhotoSlideShow(photoId);
}
public class Photo implements Runnable {
String photoId, url;
public Photo(String photoId, String url) {
super();
this.photoId = photoId;
this.url = url;
}
@Override
public void run() {
// TODO Auto-generated method stub
try {
inputStream = new java.net.URL(url).openStream();
bitmap = BitmapFactory.decodeStream(inputStream);
bundle = new Bundle();
bundle.putParcelable(KEY, bitmap);
message = new Message();
message.setData(bundle);
hander.sendMessage(message);
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
Log.e(TAG, "malformed URL exception caught : ", e);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
Log.e(TAG, "IO exception caught : ", e);
}
}
}
public class PhotoSlideShow implements Runnable {
int photoId;
String url;
public PhotoSlideShow(int photoId, String url) {
super();
this.photoId = photoId;
this.url = url;
}
@Override
public void run() {
// TODO Auto-generated method stub
try {
while(photoId != urlList.length){
inputStream = new java.net.URL(urlList[photoId++]).openStream();
bitmap = BitmapFactory.decodeStream(inputStream);
bundle = new Bundle();
bundle.putParcelable(KEY, bitmap);
message = new Message();
message.setData(bundle);
hander.sendMessage(message);
Thread.sleep(2000);
if (photoId == urlList.length) {
photoId = 0;
}
}
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
Log.e(TAG, "malformed URL exception caught : ", e);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
Log.e(TAG, "IO exception caught : ", e);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
The above is for a string-array :
<string-array name="photo_urls">
<item>http://farm8.staticflickr.com/7344/8730715795_49c6f7ca46.jpg</item>
<item>http://farm8.staticflickr.com/7429/8731821684_af03019d07.jpg</item>
<item>http://farm8.staticflickr.com/7284/8731853690_4924a98ef5.jpg</item>
<item>http://farm8.staticflickr.com/7424/8731831644_64e84a201c.jpg</item>
<item>http://farm8.staticflickr.com/7406/8731851660_483b4f6e73.jpg</item>
<item>http://farm8.staticflickr.com/7411/8731852556_5e50c98421.jpg</item>
<item>http://farm8.staticflickr.com/7295/8730733859_14dd8c7b67.jpg</item>
<item>http://farm3.staticflickr.com/2820/9494152022_b58d9ebb97.jpg</item>
<item>http://farm6.staticflickr.com/5466/9759164264_d55bdccf99.jpg</item>
<item>http://farm4.staticflickr.com/3718/9921170466_684c556137.jpg</item>
<item>http://farm4.staticflickr.com/3754/9921216314_e8231a184b.jpg</item>
<item>http://farm8.staticflickr.com/7331/9921310143_6b009e9e46.jpg</item>
<item>http://farm8.staticflickr.com/7353/9646376146_007628ede6.jpg</item>
<item>http://farm8.staticflickr.com/7436/9646411764_b7bd9f5b07.jpg</item>
<item>http://farm6.staticflickr.com/5468/9643172863_21bf5be2a0.jpg</item>
<item>http://farm3.staticflickr.com/2822/9646410328_4cb00727d4.jpg</item>
<item>http://farm8.staticflickr.com/7413/9646423470_b00281951b.jpg</item>
<item>http://farm4.staticflickr.com/3674/9646427462_c21c232de4.jpg</item>
<item>http://farm6.staticflickr.com/5538/9646428706_69230ef004.jpg</item>
<item>http://farm8.staticflickr.com/7288/9646431680_820c3f70d2.jpg</item>
<item>http://farm8.staticflickr.com/7294/9643190645_55a6c9390f.jpg</item>
<item>http://farm6.staticflickr.com/5474/9646433970_efc97efe68.jpg</item>
<item>http://farm3.staticflickr.com/2883/9643193679_019de436ac.jpg</item>
<item>http://farm4.staticflickr.com/3755/9646438074_12b9c861f1.jpg</item>
<item>http://farm4.staticflickr.com/3759/9646438386_469eed5772.jpg</item>
<item>http://farm8.staticflickr.com/7305/9646446852_6e485f90a1.jpg</item>
<item>http://farm9.staticflickr.com/8032/8043029022_06643dc31e.jpg</item>
</string-array>
Now, I am planning to implement the same using XML and JSON by retrieving images from flickr API and need a little head start on this one. Any help would be great ! Thanks...
1 Answer

Ben Jakuben
Treehouse TeacherIf you can spare the money for this book, I highly recommend it. It specifically has a section devoted to using the Flickr API and displaying images in a gallery. :) http://www.amazon.com/Android-Programming-Ranch-Guides-ebook/dp/B00C893P8U/ref=sr_1_1?ie=UTF8&qid=1382544179&sr=8-1&keywords=big+nerd+ranch+android
And here's a question on StackOverflow that links to some sample code that might help: http://stackoverflow.com/questions/4748283/flickr-api-on-android
GAUTHAM RAVI PRAKASH
2,164 PointsGAUTHAM RAVI PRAKASH
2,164 PointsThanks a lot for your help ! I was checking that code from StackOverflow and that was going way over my head !! So might have to look into that book now...is there an ebook or a pdf of it already available? couldn`t find one after searching for it..
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherYou should be able to get the Kindle version from Amazon. Not sure what other formats, though. Looks like you can download the code, at least, from their site: http://www.bignerdranch.com/book/android_the_big_nerd_ranch_guide
GAUTHAM RAVI PRAKASH
2,164 PointsGAUTHAM RAVI PRAKASH
2,164 PointsThanks for the link ! I believe those PhotoGallery codes should help me out...