Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial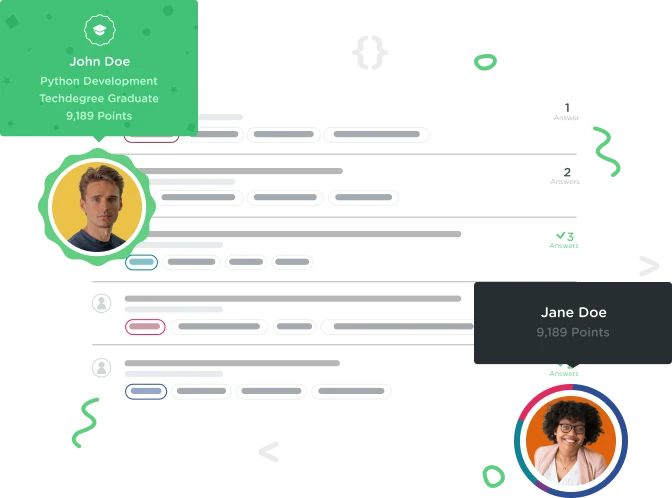

David Snow
Courses Plus Student 1,011 PointsTrying to create an array to store UIColors
I am trying to create an array of UIColors in multiple files and am having a problem implementing it. DFCrystalBall.h <p>-(IBAction)buttonpressed{//button event self.predictionLabel.text = [self.crystalBall randomPrediction];//calls method randomPrediction from CrystallBall class self.predictionLabel.textColor = [self.crystalBall randomColor];//sets text color when button is pressed} </p> <p>@interface DFCrystalBall : NSObject { NSArray _predictions; NSArray_colors; }
@property (strong, nonatomic, readonly) NSArray *predictions;//readonly is a form of encapsulation
@property (strong, nonatomic, readonly) NSArray *colors;
-(NSString*) randomPrediction;
-(UIColor*) randomColor;
//get method -(NSArray*) predictions;
//set method -(void) setPredictions:(NSArray*) newPredictions;
-(NSArray*) colors;
-(void) setColor:(NSArray*) newColor;
@end </p>
<p>-(NSArray*) colors{ if(_colors == nil) { _colors=@[[UIColor redColor],[UIColor blueColor], [UIColor greenColor], [UIColor purpleColor]]; } return _colors; }//end colors
-(UIColor*) randomColors { int random = arc4random_uniform(self.colors.count); return [self.colors objectAtIndex:random]; }//end randomColors </p>
7 Answers

Stone Preston
42,016 Points@interface DFCrystalBall : NSObject { NSArray _predictions; NSArray_colors; }
you are missing a space in between NSArray and _colors there. that could be causing some issues

David Snow
Courses Plus Student 1,011 PointsDidn't work plus objective-c isn't so much white space dependent. But the hazard I'm receiving is that I am trying to assign UIColor to NSArray

Stone Preston
42,016 Pointswhile thats true that some white space is ignored by the compiler not all of it is. in this case having NSArray_colors cause a syntax error since a white space is used to distinguish the end of the data type from the object name. Also why do you have the instance variables _colors and _predictions in addition to the properties? when using properties the _propertyName variables are generated for you, you dont have to declare them yourself. what errors/problems are you experiencing?

Stone Preston
42,016 Pointsif you want to have an array of UIColors, you could assign the UIColors to the array using initWithObjects like so:
self.colors = [[NSArray alloc] initWithObjects:[UIColor redColor], [UIColor whiteColor], nil];

David Snow
Courses Plus Student 1,011 PointsWhy would I need to do that for UIColor objects and not string objects. For instance I can allocate an array of quotes like this: _predictions=@[@"blah", @"some word"]; with no issues.

Stone Preston
42,016 Pointsyou could do that. thats using an Array literal. there are many ways of putting objects in an array. I was just showing you one of the ways

Stone Preston
42,016 Pointscan you post your implementation of this method -(void) setColor:(NSArray*) newColor;
?

David Snow
Courses Plus Student 1,011 PointsAre you speaking specifically of the setter method that you have posted? If so how would I actually implement this method?

Stone Preston
42,016 Pointsim speaking specifically of the setter method you have listed in your interface file here:
@property (strong, nonatomic, readonly) NSArray *colors;
-(NSString*) randomPrediction;
-(UIColor*) randomColor;
//get method -(NSArray*) predictions;
//set method -(void) setPredictions:(NSArray*) newPredictions;
-(NSArray*) colors;
-(void) setColor:(NSArray*) newColor;
its the last one listed Have you implemented it?

David Snow
Courses Plus Student 1,011 PointsOkay to answer one of your earlier questions the reasons why I had both the instance variable and the @property(s) was because I had not gotten that far in the tutorial/extra credit but it does make more sense to not have both of those. I believe I have also properly refactored the application or at least I hope I did it a decent way.
{
NSArray *colors = @[[UIColor greenColor],[UIColor redColor],[UIColor blueColor], [UIColor cyanColor],[UIColor purpleColor],[UIColor grayColor],[UIColor orangeColor],[UIColor magentaColor], [UIColor brownColor]];
NSInteger randomColorIndex = arc4random()%[colors count];
return [colors objectAtIndex:randomColorIndex];
}
```-(IBAction)buttonpressed{//button event { self.predictionLabel.text = [self.crystalBall randomPrediction];//calls method randomPrediction from CrystallBall class self.predictionLabel.textColor = [self.crystalBall randomColor]; }
I have one more question though how do I post my code snippets the proper way. As you can probably tell I am a total N00B. Thanks