Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial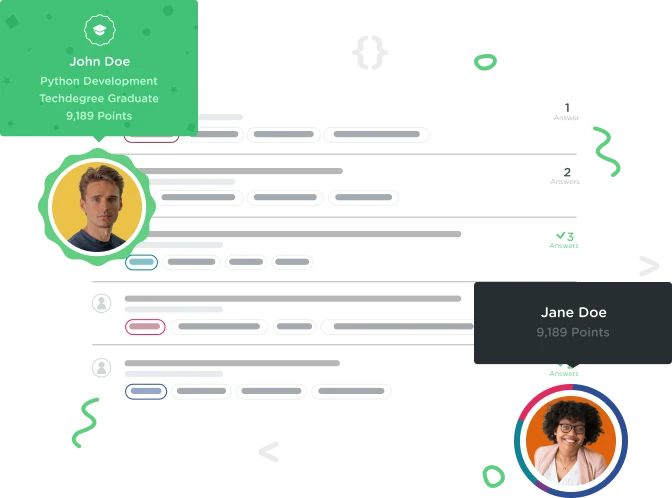
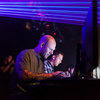
Adam Duffield
30,494 PointsTrying to get a nice animation on a scrollToTop?
Hi,
The code below makes my header above my navigation bar dissapear after it goes 100px down the page which is exactly what I am trying to do. The only issue I am having is that it makes the change instantly. Where can I place .animate() within this code to make the transition of display block to none a little smoother?
var $logo = $('.header_above_nav');
$(document).scroll(function() {
$logo.css({display: $(this).scrollTop() < 100 ? "block":"none"});
})
I suppose I could try to animate it's height from 100% slowly down to 0% also but thats more of a wildcard idea at the moment.
Many thanks,
Adam
2 Answers

Dan Hedgecock
13,807 PointsI like to use opacity in transitions like the first answer, but if you're just looking to hide the element without changing opacity you could use jQuery's slideToggle method, it's made for almost this exact situation. It hides an element by using a slide animation.
Something like:
var $logo = $('.header_above_nav');
$(document).scroll(function() {
if ($(this).scrollTop() > 100) {
$logo.slideToggle()
}
});
jquery API: http://api.jquery.com/slidetoggle/

Marco Slooten
7,899 PointsThe simplest way too do this, would be to use jQuery's .hide(), but that would also decrease the width during the animation. I would opt for animating the 'opacity' property, and perhaps after that animation running .hide() to completely remove the element. Something like this:
var $logo = $('.header_above_nav');
$(document).scroll(function() {
if ($(this).scrollTop() >100) {
$($logo).animate({
opacity: 0
}, 1000, function() {
$(this).hide();
});
}
});
So you have this condition that if the scroll exceeds 100, the variable $logo animates its opacity until it's 0 (rendering it invisible). Then in the completion function, you can hide the element to set the display to none. I hope it's clear like this! Let me know if this is what you meant.
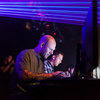
Adam Duffield
30,494 PointsNot quite what I'm after but the code does work, thanks for this but I'm after a smooth transition as I scroll down the page like the on Dan has suggested.
Adam Duffield
30,494 PointsAdam Duffield
30,494 PointsHey Dan cheers for this, it almost does exactly what I'm after apart from it constantly slides up and down once I go past 100px. The header is relative at the top of the page but after 100px it becomes fixed to the top and this might effect your code. I've tried playing around with it and adding a semi-colon to slideToggle but it still repeatedly slides up and down.
Marco Slooten
7,899 PointsMarco Slooten
7,899 PointsCheck out .slideUp(), this is the same as slideToggle(), but it only slides up. jQ API: http://api.jquery.com/slideUp/ (you can find this under Effects > Sliding). This will only run once, and then the element is hidden.
This is basically the same as animating the CSS height property to 0 and then in the completion function hiding it altogether. But way easier :)
So try this:
Adam Duffield
30,494 PointsAdam Duffield
30,494 PointsMarco, that is almost exactly what I am after! The only issue I have left is after scrolling down the page and back up 2 or 3 times it then refuses to slidebackup and leaves a blank space where I want it to consistently slide up or down following the rules I set in the code below...
Thanks again,
Adam
Marco Slooten
7,899 PointsMarco Slooten
7,899 PointsHi Adam,
It's hard to say without having the rest of the code. Might have something to do with the fixed positioning.
Also; are you aware that your conditions are overlapping? For instance: everything above 224px scroll will trigger the first condition. So the second condition (<500) will ONLY run if scroll is lower than 224px. It works, but it's harder to predict the behavior of the code by just looking at it.
Adam Duffield
30,494 PointsAdam Duffield
30,494 PointsSorry to be a pain Marco, I can't reveal the website I'm working on but there really isn't much code to this issue either. Basically the navigation bar is always fixed at the top and after 225px down the webpage the tagline above my navigation bar slidesUp and dissapears. I need it so that when I get back to the top of the page the tagline will slide back so that I won't have a gap between my content and my navigation. I've changed the code and tried this below, but when I'm so far down the page or try so many times it stops sliding back down.
Marco Slooten
7,899 PointsMarco Slooten
7,899 PointsYou say your nav is fixed, and when the tagline disappears there's a gap between the content and the nav? So it's: tagline, nav, content, and when you scroll the tagline disappears, the nav slides up to fill the space and leaves a space between the content?
You probably have some margin or padding on top of the content to prevent the navbar from overlapping content? In the same condition that slides up the tagline, you could modify that margin.
That's the best I can do without a working example, I'm afraid. Good luck!