Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial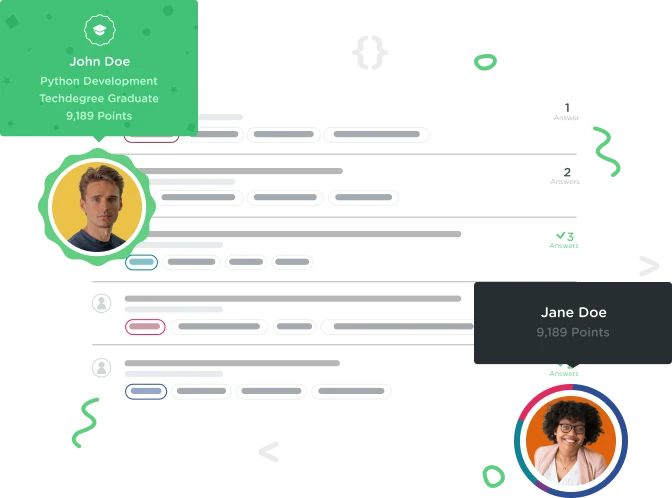

elena meneghini
iOS Development with Swift Techdegree Student 9,832 PointsTrying to get a random UIColor from an array of dictionaries
After watching FunFacts videos, I'm trying to make a similar app that picks a random color from a list of IUColors but instead of displaying a random fact, it displays the name of the specific color chosen randomly.
I started making an array of dictionaries in order to have the name associated with the UIColor, then I'm trying to add a method to get the name of the color to display in the view and I'm not sure how to get only the name(keys) from the dictionary:
import UIKit
import GameKit
struct colorProvider {
let GMDcolors = [ "Pomegranate" : UIColor(red:0.96, green:0.26, blue:0.21, alpha:1.0),
"Amaranth" : UIColor(red:0.91, green:0.12, blue:0.39, alpha:1.0),
"Seance" : UIColor(red:0.61, green:0.15, blue:0.69, alpha:1.0),
"Purple Heart" : UIColor(red:0.40, green:0.23, blue:0.72, alpha:1.0),
"San Marino" : UIColor(red:0.25, green:0.32, blue:0.71, alpha:1.0),
"Dodger Blue" : UIColor(red:0.13, green:0.59, blue:0.95, alpha:1.0)
]
func getRandomColor() -> String {
let randomNumber = GKRandomSource.sharedRandom().nextInt(upperBound: GMDcolors.count)
return GMDcolors[randomNumber]
}
}
not sure I'm doing it right, can someone help me?
2 Answers
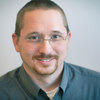
Jesse Anderson
iOS Development Techdegree Student 9,702 PointsNot sure this will help, but you can get an array of the keys by using GMDcolors.keys
and from there you should be able to get a count of all the keys using GMDcolors.keys.count
or you could even assign the array to a different variable like
keyArray = GMDcolors.keys
for key in keyArray {
do something with the individual key here
}
So you can see where you can take the dic.keys value and use that in a couple of different ways. I hope that helps out. You can read more about it in the Apple documentation

elena meneghini
iOS Development with Swift Techdegree Student 9,832 Pointsyes, I forgot the double square brackets sorry! So in this case, how could I return, in the last method, only the name of the color?
struct ColorProvider {
let GMDcolors: [[String: UIColor]] = [
[ "Pomegranate" : UIColor(red:0.96, green:0.26, blue:0.21, alpha:1.0)],
[ "Amaranth" : UIColor(red:0.91, green:0.12, blue:0.39, alpha:1.0)],
[ "Seance" : UIColor(red:0.61, green:0.15, blue:0.69, alpha:1.0)],
[ "Purple Heart" : UIColor(red:0.40, green:0.23, blue:0.72, alpha:1.0)],
[ "San Marino" : UIColor(red:0.25, green:0.32, blue:0.71, alpha:1.0)],
[ "Dodger Blue" : UIColor(red:0.13, green:0.59, blue:0.95, alpha:1.0)],
[ "Cerulean" : UIColor(red:0.01, green:0.66, blue:0.96, alpha:1.0)],
[ "Robin's Egg Blue" : UIColor(red:0.00, green:0.74, blue:0.83, alpha:1.0)],
[ "Persian Green" : UIColor(red:0.00, green:0.59, blue:0.53, alpha:1.0)]
]
func getRandomColor() -> [String: UIColor] {
let randomNumber = GKRandomSource.sharedRandom().nextInt(upperBound: GMDcolors.count)
return GMDcolors[randomNumber]
}
}
The label should display the name of the color in the view controller:
@IBOutlet weak var colorNameLabel: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
colorNameLabel.text = colorProvider.getRandomColor()
}

Magnus Hållberg
17,232 PointsWhats the reason for making it an array of dictionaries? Why not just a dictionary, do you need it ordered? If not I cant see the benefit, now you need both an array index and a dictionary key to access the value. A plain dictionary seems much easier. Then you could do something like:
let GMDcolors: [String: UIColor] = [ "Pomegranate" : UIColor(red:0.96, green:0.26, blue:0.21, alpha:1.0), "Amaranth" : UIColor(red:0.91, green:0.12, blue:0.39, alpha:1.0), "Seance" : UIColor(red:0.61, green:0.15, blue:0.69, alpha:1.0), "Purple Heart" : UIColor(red:0.40, green:0.23, blue:0.72, alpha:1.0), "San Marino" : UIColor(red:0.25, green:0.32, blue:0.71, alpha:1.0), "Dodger Blue" : UIColor(red:0.13, green:0.59, blue:0.95, alpha:1.0), "Cerulean" : UIColor(red:0.01, green:0.66, blue:0.96, alpha:1.0), "Robin's Egg Blue" : UIColor(red:0.00, green:0.74, blue:0.83, alpha:1.0), "Persian Green" : UIColor(red:0.00, green:0.59, blue:0.53, alpha:1.0) ]
func getRandomColorKey() -> String { var tempArray = String let randomNumber = Int(arc4random_uniform(GMDcolors.count - 1))
for (key, value) in GMDcolors {
tempArray.append(key)
}
return tempArray[randomNumber]
}
I'm not sure this is 100% correct since I'm not "coding" on a Mac in a playground at the moment so its not tested. I don't remember if arc4random starts at 0 or 1 so you might not need the "- 1" part.
Sorry, cant get the syntax highlighting to work so this looks really confusing. However, hope this helps.
Magnus Hållberg
17,232 PointsMagnus Hållberg
17,232 PointsThe code you have posted is no array of dictionaries, its just a dictionary. An array of dictionaries would look like this [["Pomegranate" : UIColor(red:0.96, green:0.26, blue:0.21, alpha:1.0)], ["Amaranth" : UIColor(red:0.91, green:0.12, blue:0.39, alpha:1.0)]]... Hope that helps.