Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial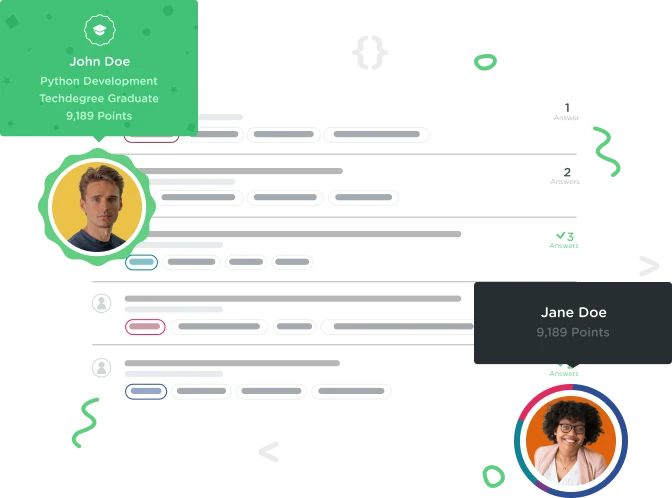

Yue Yue
543 PointsTrying to get past the Try/Except question
Here's my code but it says It looks like Task 1 is not passing
def add(a,b): try: return float(a) + float(b) except: ValueError return None else: return a + b
def add(a,b):
try:
return float(a) + float(b)
except: ValueError
return None
else:
return a + b
4 Answers
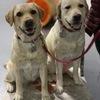
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Yue Yue,
Glad to hear that the challenge passed for you but I should warn you that the code you just described wouldn't work the way you would expect and I think you might still be confused about how the except
works.
The reason I said that your colon was in the wrong place and not the ValueError is that you are only trying to catch exceptions that are of the type ValueError. To do this you write the following:
except ValueError:
When you use this code, any bad value that causes the code in the try
statement to fail will trigger a ValueError and be handled by the except clause. Importantly, any value that fails for a reason other than a ValueError (e.g., a TypeError) will not be caught by the except clause and will just crash with a stack trace.
The reason that this is important is so that when you write error handling code, you are actually handling the type of error you think you are: when you write a try statement you are doing so because you have some specific failure case in mind, which is what your except is catching. You still want the program to crash if an error that you didn't expect goes through the try statement because the code you wrote to handle the error you do expect probably isn't the right code to handle the error type you didn't expect.
In addition to this code which catches specific types of exception, there is a 'catch all' clause which will handle every type of error that wasn't specifically handled somewhere else. This is written as follows:
except:
Although this is valid Python it has narrow usage, it's generally used to pass the error off to some other part of code that will handle (or at least alert) that there is an error happening that the code can't resolve. In the real world, it's very unlikely that error handling code that can handle every possible error type can be written in that except block.
Moving the ValueError onto the next line doesn't do anything. It's like writing just 'hello world' on a line in the program, it doesn't get rendered to the screen, it doesn't alter the return value, it doesn't change any state. There is no situation where you'd just write an error name as a line of code.
I strongly recommend that you rewatch the 'Exception to the Rule' video to be sure you understand this concept as you are going to be seeing it a lot in future code you read and write. And if your error handling code doesn't behave the way you expect you are going to have a very frustrating experience (well, even more frustrating!) chasing down stubborn bugs.
Cheers
Alex
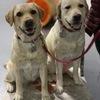
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Yue,
Take a look at the position of the colon in your except
line.
Cheers
Alex

Yue Yue
543 PointsCheers Alex - grateful for your help - worked once I moved the ValueError down to the next line :-)

Yue Yue
543 PointsAlex,
Thanks for taking the time to put me right - much appreciated.
Yue
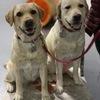
Alex Koumparos
Python Development Techdegree Student 36,887 PointsYou're welcome, happy coding!