Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial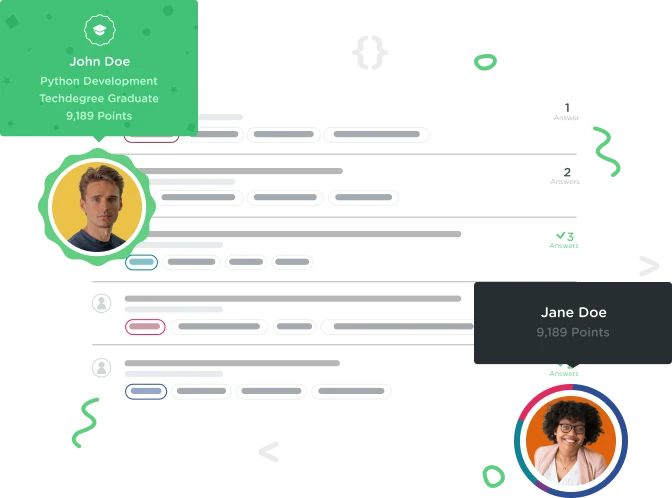
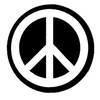
john larson
16,594 PointsTrying to get the len of a list for comparison, But I'm doing something wrong
I'll probably slap myself in the head when I find what I'm doing wrong. Nudge me in the right direction please.
teachers_dict = {
'Jason Seifer': [
'Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': [
'Python Basics', 'Python Collections']
}
jason = []
kenneth = []
def most_classes(teachers_dict):
for key in teachers_dict:
if key == "Jason Seifer":
jason.append(teachers_dict[key])
else:
kenneth.append(teachers_dict[key])
# these prints are just for testing and debugging
print(jason)
print(len(jason))
print(kenneth)
print(len(kenneth))
# I need the length of the lists for comparison
# I can see three items in the first list
# but I only get a len of 1
# two in the second list, but again a len of 1
# [['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations']]
# 1
# [['Python Basics', 'Python Collections']]
# 1
# if len(jason) > len(kenneth):
# return "Jason Seifer"
# else:
# return "Kenneth Love"
most_classes(teachers_dict)
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi John,
The reason you're having the problem with the len() function is because you have a list within a list. You can see this in your comments.
You initialize jason
to an empty list and then you append the list of courses to that empty list. So your outer list only has 1 item in it. That item happens to be a list itself, with 3 items in it.
len(jason) # 1
len(jason[0]) # 3
The overall problem though is that you're coding a solution specifically for the example dictionary given. It's only meant to be an example so that you could see the structure of it. You don't want to make any assumptions about what teachers will be in this dictionary.
You don't have to create this dictionary and you also don't have to call your function. The challenge tester will do these things for you.
The comments in the code give you some tips on how to code the solution.
You want to keep track of the current maximum and also the teacher that has that current maximum. You would update these 2 variables when you find a teacher that has more courses than the current maximum.
Let me know if you need more hints.
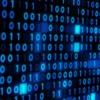
Alexander Davison
65,469 Pointsget ready to slap ur forehead..... lol
your most_classes function didn't return anything.
You forgot to return the teacher with more classes! LOL Hope it helps
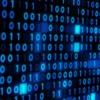
Alexander Davison
65,469 PointsAdd this code to the end of your most_classes function (and at least I think ti should work):
if len(kenneth) > len(jason):
return "Kenneth Love"
else:
return "Jason Seifer"
Lol hope it helps and get ready to slap ur forehead hard
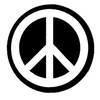
john larson
16,594 PointsAlexander, I had similar logic in my editor, but I found that for a reason I don't get, I keep returning a length of one for both lists that I am comparing. So I took out that code to focus on what I think is causing the problem. I'll post my full code if you feel like taking another look. It did not pass the challenge for me.
teachers_dict = {
'Jason Seifer': [
'Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': [
'Python Basics', 'Python Collections']
}
jason = []
kenneth = []
def most_classes(teachers_dict):
for key in teachers_dict:
if key == "Jason Seifer":
jason.append(teachers_dict[key])
else:
kenneth.append(teachers_dict[key])
print(jason)
print(len(jason))
print(kenneth)
print(len(kenneth))
# is returning this in the console
# I need the length of the lists for comparison
# I can see three items in the first list
# but I only get a len of 1???
# [['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations']]
# 1
# [['Python Basics', 'Python Collections']]
# 1
if len(jason) > len(kenneth):
return "Jason Seifer"
else:
return "Kenneth Love"
result = most_classes(teachers_dict)
print(result)
I'm not ready to slap my head yet :D
john larson
16,594 Pointsjohn larson
16,594 PointsThat's great! I'll keep those points in mind.