Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial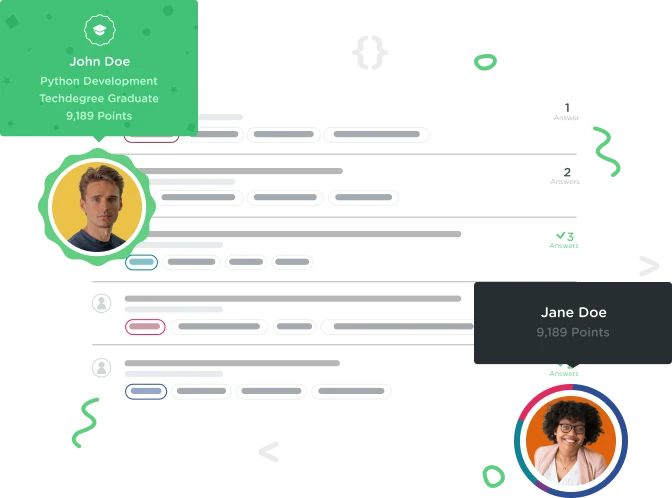
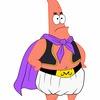
<noob />
17,062 PointsTrying to make an Hangman game and stuck.
Hi, Im trying to make an Hangman game in python.
However I have 2 problems rn.
- I tried to make a function which let to see the progress of the game, what does it mean? if the word is "python", then if the user enters "p" i want to see the progress like so : p---- and if he guessed "n" then i want to see the progress again like so: p----n.
this is the fucntion that i wrote:
def get_progress(user_guess,word):
#for debuing purposes
progress = ""
for letter in word:
display = "-"
if user_guess == letter and user_guess in word:
display = user_guess
progress += display
return progress
It's actually working, but when i guess another letter the progress is reseted, I know why, it's because the user_guess is changing evreytime, However i couldn't figure a way to modify the function..
2.the second problem that im facing is with restarting the game. it works great if the player press "y" and if the player press "n" but if the player for example press quit and then he decides to stay it dosent break out of the program, This is a demonstration of the output: https://gyazo.com/c27b3449178a731039d3d334d142a613
this is the current snapshot, The file is called hangman.py, Check it see what i already did : https://w.trhou.se/2ivnf1lxqi
I will appreciate your help
5 Answers
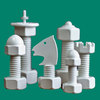
Steven Parker
229,732 PointsAt first glance, it looks like you need a "break" after the call to game_loop on line 45. Otherwise, when you call game_loop() to start a new game, the current one is just put on hold. Then when the new game ends, the current one resumes. Having a "break" as the next step will cause the current one to end also.
The 2015 version of Python Basics with Kenneth Love had a hangman game (called "letter game") as the "code along" project. It has since been refreshed, and the new version has a different project. But if you look through the forum for questions asked about that project you might find some still-active snapshots of mostly complete programs.
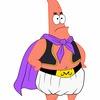
<noob />
17,062 PointsHey steven thanks for the reply. Your suggestion worked!, How so though?, as far as i know, when we use break we break out of the loop so how exactly adding the "break" after the call to game_loop() doesn't break out of the program when pressed "n"?
I did the hangman game in java but it's quite different here.
What i added today:
1.a feature which allow the user to guess a wrong letter 7 times, otherwise he's losing.
- A "cheat" mode which allow the user to get the word if he wants to cheat XD
However I still couldn't figure out a way to make the progress function() to behave like i mentioned above, It get reset because user_choice is changing each time like i said but idk how to fix it.
This is my latest tries:
def get_progress(user_guess,word):
#for debuing purposes
progress = ""
for letter in word:
is_hit = word.find(letter)
display = "-"
if is_hit != -1:
if user_guess == letter:
display = user_guess
progress += display
return progress
def get_progress(user_guess,word,guesses):
progress = ""
converted = "".join(guesses)
for letter in word:
display = "-"
if converted.find(letter):
if user_guess == letter:
display = user_guess
progress += display
return progress
I just can't figure out how to keep the letters that has been guessed and that are in the word when i guess another letter
And i couldn't figure out how to get a winner, this function is depends on the get_progress() function, in java course they did a check to check if get_progress contain "-" if it isn't the user won.
this is the current snapshot with improvement >> THE FILE IS CALLED hangman.py
I will appreciate you're help i just can't figure this out
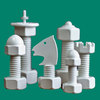
Steven Parker
229,732 PointsI updated my answer
This might be a great time for you to work on your debugging skills – which are very important for a developer. Spend a bit more time with it and draw from other available resources. Those old Python "letter game" forum questions for example, they might give you an idea for an alternative strategy for retaining and displaying guesses.
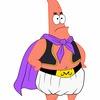
<noob />
17,062 PointsHey steven thanks for the helpful link!, it helped me to figure out a way to display the guesses like i wanted to. I did a function instead of while loop because it's unnecessary.
This is the current snapshot:
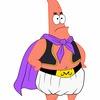
<noob />
17,062 PointsHey steven I didn't had time to update my comment, I figure out how to check for a WIN situation as well during exploring the discussions, I didn't know how I didn't thought about this way like Kenneth did, it's so simple but genious! :]
However I added some of my own features as well, like a show command which automatically shows the good guesses and the bad guesses if the user forgot for some reason.
I also refactored the code as well.
This project was abit hard but I completed it :], thanks to ur guide and I will really appreciate if u can check the final version:
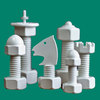
Steven Parker
229,732 PointsWhen you have a series of exclusive choices you should use "elif" after the first one. Because of the separate "if"s, the "cheat" function doesn't work because it is also treated as an invalid input.
But otherwise, good job!
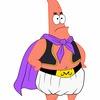
<noob />
17,062 PointsThanks!, It’s weird it worked when i checked it , i will check it thanks!, any other suggestions?