Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial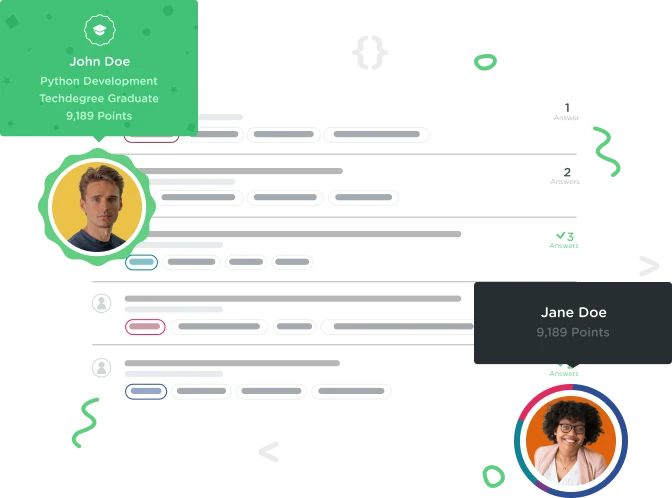

Jeffrey Kwok
3,006 PointsTrying to parse JSON file in node js but I get a "Cannot read property 'length' of undefined" error
Hi,
The title explains it all. The json file url and code is below. What I want to happen is for the "description object", "title object" and " downloadUrl object" to be printed out
Can anyone help?
CODE:
const https = require('https');
const apikey = "XXXXXXXXX";
// Connect to the API URL
const request = https.get(`https://core.ac.uk/api-v2/articles/search/cells?page=1&pageSize=20&metadata=true&fulltext=false&citations=false&similar=
false&duplicate=false&urls=true&faithfulMetadata=false&apiKey=${apikey}`, response => {
let body = "";
// Read the data
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
// Parse the data
const profile = JSON.parse(body);
// Print the data
// console.dir(profile);
});
var json = body;
for(var i = 0; i < json.line.length; i++)
{
console.dir(" Description: " + json.line[i].description + " title: " + json.line[i].title + " downloadUrl: " + json.line[i].downloadUrl);
//
}
3 Answers

Alexander La Bianca
15,959 Pointsresponse.on('end') takes a callback which you have, but you need to put your json loop inside the 'end' event listener. like so:
response.on('end', () => {
// Parse the data
const profile = JSON.parse(body);
// Print the data
// console.dir(profile);
var json = body;
for(var i = 0; i < json.line.length; i++)
{
console.dir(" Description: " + json.line[i].description + " title: " + json.line[i].title + " downloadUrl: "
+json.line[i].downloadUrl);
}
});
I have not tried running it, but the error you got means that line in json was not defined which makes sense if you have it on the outside of your 'end' listener.

Jeffrey Kwok
3,006 PointsHi Alex,
you may need to install a json viewer add on your browser so that its in a readable format
Basically, the key value pairs for "description", "title" , and "downloadUrl" are all nested in the "data" array of the json filee. Does that answer your question? Let me know if you need clarification.

Alexander La Bianca
15,959 PointsThanks. Yes so you need to loop over the json.data instead over the json.line.
const request = https.get(`https://core.ac.uk/api-v2/articles/search/cells?page=1&pageSize=20&metadata=true&fulltext=false&citations=false&similar=false&duplicate=false&urls=true&faithfulMetadata=false&apiKey=${apikey}`, (response) => {
let body = "";
response.on('data', data => {
console.log('Reading Data');
body += data.toString();
});
response.on('end', () => {
// Parse the data
const profile = JSON.parse(body);
var json = body;
console.log(json.line); //prints undefined. so you can't call json.line.length on it wihtout throwing an error
console.log(json.data); //prints the data you want
for(let i = 0; i<json.data.length;i++) {
//do stuff with json.data[i].description etc. here
}
});
});

Jeffrey Kwok
3,006 PointsThanks for the help Alex! I appreciate it :)
Jeffrey Kwok
3,006 PointsJeffrey Kwok
3,006 PointsHI Alex,
Thank you for the reply. I tried what you recommended but I still get the same error. I think it might me because some of the "description" and "title" keys are null. Would that have an effect?
Alexander La Bianca
15,959 PointsAlexander La Bianca
15,959 PointsHi Jeffrey,
No. Your problem is that the property 'line' is undefined. So json does not have a property named line. I know that because when I run the following code, it prints 'undefined'
What is the json strucutre looking like? How do you know you need to access the line property? If you can provide an example I may be able to help you out.
Jeffrey Kwok
3,006 PointsJeffrey Kwok
3,006 PointsHi Alex,
Sorry to bug you again. I did try implementing "json.data" as you suggested but I'm still getting the same error. I made a genuine attempt to solve it by looking back on previous tutorials and looking through stackoverflow to no avail. Is there any other reason why the error keeps appearing? below is the code:
Alexander La Bianca
15,959 PointsAlexander La Bianca
15,959 PointsHi Jeffrey,
Sorry I should have caught this yesterday already, but you pretty much do not need the line var json = body; As you are parsing the data with const profile = JSON.parse(body); Turning the body into json and assigning it to profile. Now you need to refer to
profile
then in your loop and not json. Like so:I actually tried it out this time lol and it works. It takes a few seconds for the response to come back though so be patient.