Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial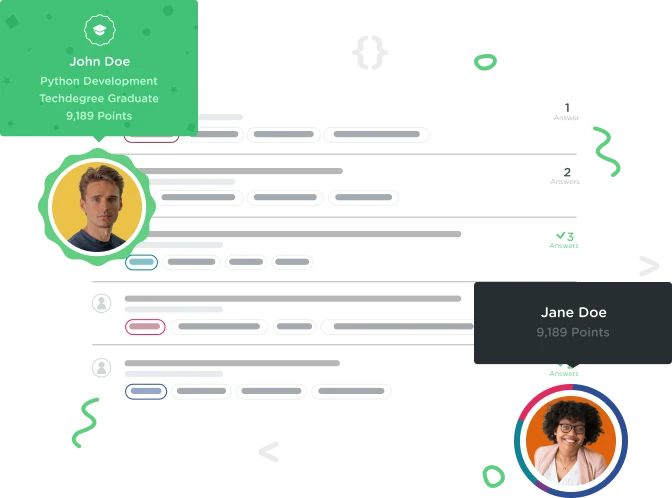

zhengyutang
9,548 PointsTrying to pass the Django TDD. manage.py test all passed, but the tests on treehouse are not passing.
Is there anything I missed in my code? Thank you.
from django.db import models
class Song(models.Model):
title = models.CharField(max_length=255)
artist = models.CharField(max_length=255)
performer = models.ForeignKey('Performer')
length = models.IntegerField()
def __str__(self):
return '{} by {}'.format(self.title, self.artist)
class Performer(models.Model):
name = models.CharField(max_length=255)
def __str__(self):
return self.name
from django.shortcuts import render
from django.views import generic
from .models import Song, Performer
class SongListView(generic.ListView):
model = Song
context_object_name = 'songs'
template_name = 'songs/song_list.html'
class PerformerDetailView(generic.DetailView):
model = Performer
context_object_name = 'performer'
template_name = 'songs/performer_detail.html'
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['performer'] = Performer.objects.get(pk=self.kwargs['pk'])
return context
class SongDetailView(generic.DetailView):
model = Song
context_object_name = 'song'
template_name = 'songs/song_detail.html'
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['song'] = Song.objects.get(pk=self.kwargs['pk'])
return context
{% extends 'base.html' %}
{% block title %}{{ performer }}{% endblock %}
{% block content %}
<h2>{{ performer }}</h2>
<ul>
{% for song in performer.song_set.all %}
<li>{{ song }}</li>
{% endfor %}
</ul>
{% endblock %}
3 Answers
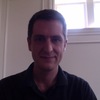
Max Hirsh
16,773 PointsI can't spot anything wrong with your code. I ran into this issue as well but was able to submit the code successfully the third time I tried it. If it's passing the TDD.manage.py tests, I recommend you try reloading the submit page or using a different browser to see if that helps.
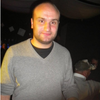
Vittorio Somaschini
33,371 PointsHello Zhengyu.
Are you sure it is passing all the tests in the workspaces? Because I have tested it in workspaces and it does not pass (I simply copied and pasted your code in the right files).
To be precise:
I have run the tests one by one (out commenting the other tests) and: The models look ok, they both pass the test.
The views return errors, so out of 5 tests I got 3 errors...
Vittorio

zhengyutang
9,548 PointsReally! Would you be able to post the Trace back here?
I did not work in the workspaces. I downloaded the project files and worked on locally. In the meantime, I will to work put it up to the workspaces and try again. Thanks a lot.
Ryan

zhengyutang
9,548 Pointstreehouse:~/workspace/karaoke$ python manage.py test
Creating test database for alias 'default'...
.....
Ran 5 tests in 0.090s
OK
Destroying test database for alias 'default'...

zhengyutang
9,548 PointsNever mind, I probably know what is going on here now. The error might do to the urls.py file. Class views are not supported, because the urls.py is waiting for functional views.
Ryan
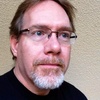
Chris Freeman
Treehouse Moderator 68,423 PointsAre all the tests passing now?