Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial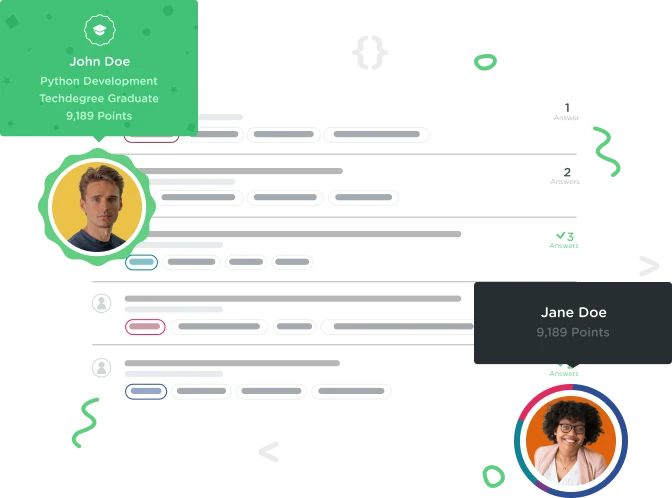

James Kim
8,475 PointsTrying to run setInterval() on Refactor challenge on Javascript Loops, Arrays, and Object course.
Hello fellow tree house students.
For those who don't know the program in the course, this is a simple web app that generates circles with random rgb values. I decided to add a setInterval() to make it change colors every "x" seconds, but i can not seem to get it down. I have pasted two codes on the bottom which seems logical to me but, it doesnt run. I appreciate all the help, hints, advices, and insights from you.
Thank you.
/***************
FIRST CODE
***************/
var html = '';
var red;
var blue;
var green;
var rgbColor;
function colorChanger(){
var color = Math.floor(Math.random()*256);
return color;
}
for (var i = 0; i < 10; i += 1) {
red = colorChanger();
green = colorChanger();
blue = colorChanger();
rgbColor = rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);
setInterval(colorChanger, 2000);
/******************
SECOND CODE
******************/
var html = '';
var red;
var blue;
var green;
var rgbColor;
function change () {
for (var i = 0; i < 10; i += 1) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);
}
change(); //for some reason when I remove the change(); the color circles do not show up.
setInterval(change, 5000);
/*****
END
*****/
1 Answer
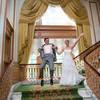
Colin Bell
29,679 PointsIn your first example, the color changer functions just returns a random number between 0-255 every 2 seconds.
In your second example, the change function creates 10 new divs with a random background color. If you run that in the setInterval then it's going to create 10 new divs every interval, but once created their color will remain stagnant.
To create divs and set the colors followed by changing the newly created divs' background at an interval you'd have to do something like this:
var html = '';
var allDiv = document.getElementsByTagName('div');
var red, blue, green, rgbColor, color;
// A modification of your original change() function that returns the
// variable instead of setting it as the background color right away
function set() {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
return rgbColor;
}
// Creates divs and sets background colors
for (var i = 0; i < 10; i += 1) {
html += '<div style="background-color:' + set() + '"></div>';
}
// Writes the divs to the document
document.write(html);
// A function that will go through all divs
// and change their background colors randomly
function change() {
for (i = 0, length = allDiv.length; i < length; i++) {
allDiv[i].style.backgroundColor = set();
}
}
// Changes each divs color every 2 seconds
setInterval(change, 2000);
James Kim
8,475 PointsJames Kim
8,475 Pointsahh... i had a feeling i needed another function with a loop, but i couldnt piece it together.
Thanks alot Colin!!