Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial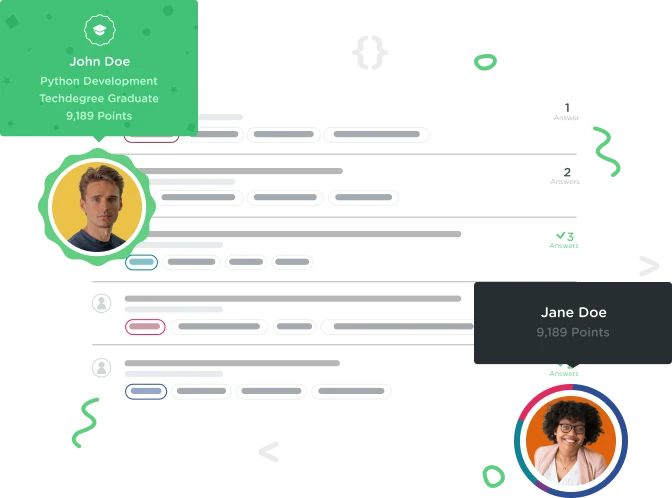
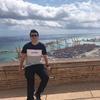
Tadjiev Codes
9,626 PointsTrying to understand setTimeOut
I'm trying to further practice the setTimeOut but can't seem to understand how to do a simple thing like displaying a string character by character.
</head>
<body>
<input type="button" id="btnPrint" value="Print" onclick="printString();" />
<div id="outDiv"></div>
</body>
</html>
function printString() {
// "flag" variable is used to determine when loop will stop
// "dummy" and "count" are used to simulate processing work
var done = false;
//var count = 0;
// the inner function is used to simulate the "while" loop
// found in UIDemo1
function innerLoop() {
var myNextChar = "The nefarious thing about performance bugs is that the user may never know they are there - the program appears to work correctly, carrying out the correct operations, showing the right thing on the screen or printing the right text. It just does it a bit more slowly than it should have. It takes an experienced programmer, with a reasonably accurate mental model of the problem and the correct solution, to know how fast the operation should have been performed, and hence if the program is running slower than it should be";
document.getElementById("outDiv").innerHTML += myNextChar;
var wordIndex = 0;
var letterIndex = 0;
var word = myNextChar[wordIndex];
var letter = word.charAt(letterIndex);
// why does this work?
// we're able to use the "setTimeout" to recursively call
// innerLoop every 5 milliseconds
// while the JavaScript system is waiting the 5 ms, it will
// process all UI events
if (!done)
setTimeout(innerLoop, 10);
}
// this starts the "loop" running by
// calling innerLoop the first time
innerLoop();
}
It would be great if somebody could explain how to do it so it displays each character of the string with 10 milliseconds delay.
Thanks in advance
3 Answers
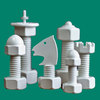
Steven Parker
231,275 PointsThe logic to display the characters isn't quite right, this code will repeat the entire string.
But if you defined and index variable in the outer function named "lx" for example, then in the inner function you could do something like this:
document.getElementById("outDiv").innerHTML += myNextChar[lx++];
if (lx >= myNextChar.length) done = true;
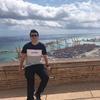
Tadjiev Codes
9,626 PointsThanks a lot, Mr.Steven)
// event handler will be called when the user clicks the button
function printString() {
// "flag" variable is used to determine when loop will stop
// "dummy" and "count" are used to simulate processing work
var done = false;
//var count = 0;
var lx = 0;
// the inner function is used to simulate the "while" loop
function innerLoop() {
var myNextChar = "The nefarious thing about performance bugs is that the user may never know they are there - the program appears to work correctly, carrying out the correct operations, showing the right thing on the screen or printing the right text. It just does it a bit more slowly than it should have. It takes an experienced programmer, with a reasonably accurate mental model of the problem and the correct solution, to know how fast the operation should have been performed, and hence if the program is running slower than it should be";
document.getElementById("outDiv").innerHTML += myNextChar[lx++];
if (lx >= myNextChar.length) done = true;
setTimeout(innerLoop, 10);
if (myNextChar.length >= 100) {
'\n'
}
/*
if (!done)
setTimeout(innerLoop, 10);
*/
}
// this starts the "loop" running by
// calling innerLoop the first time
//setTimeout(innerLoop, 10);
innerLoop();
}
It's displaying the characters of the string one by one but after there's one line of words undefined. Where's that coming from? And If I wanted to limit the display let's say having only 100 characters on one line and then start a new line with '\n' keyword doesn't seem to work as well
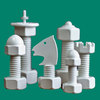
Steven Parker
231,275 PointsYou still need to reset the timeout only "if (!done)
".
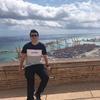
Tadjiev Codes
9,626 PointsAlright Thank you again