Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial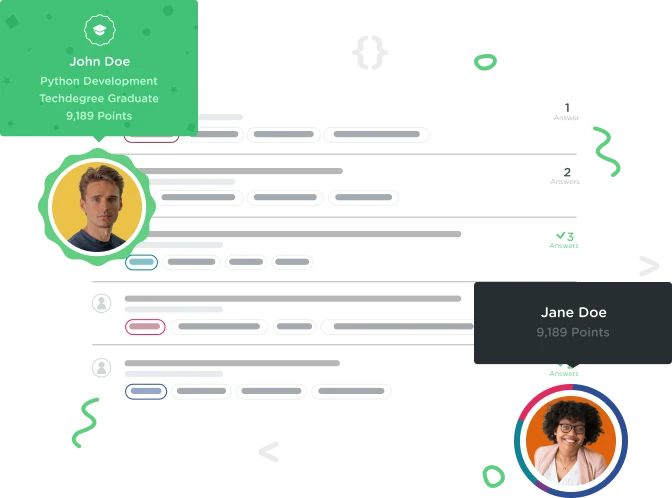

Jonathan Healy
21,601 PointsTrying to use an else statement to get the search to return a different message...
I was just messing around with doing the additional challenges on what Dave had proposed, and my first effort was to simply add to his code by returning a message stating a student records doesnt exist by using an else statement.
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentData(student) {
var data = '<h2>Student: ' + student.name + '</h2>';
data += '<p>Track: ' + student.track + '</p>';
data += '<p>Points: ' + student.points + '</p>';
data += '<p>Achievements: ' + student.achievements + '</p>';
return data;
}
while(true) {
search = prompt('Search student records: Type a student name [Big Jim] to search records (or type "quit" to end)');
if (search === null || search.toLowerCase() === 'quit'){
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( search.toLowerCase() === student.name.toLowerCase() ){
message = getStudentData( student );
print(message);
} else {
message = 'Could not find student record';
print(message);
}
}
}
If I simply remove the final else statement, the student's records display properly if their name is correctly searched. However with the addition of the else statement, even searched students who have records returns the 'could not find student' message. Can someone please explain the reasoning for this.
Side note, I also noticed that with the or operator for the break statement, if I switch the null and 'quit' conditions, and then hit the cancel button on the the search prompt, an error occurs on the java script console... why is this? Are the conditions checked from left to right with 'or' operators?
3 Answers
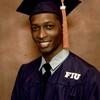
Dane Parchment
Treehouse Moderator 11,075 PointsHello Jonathan!
So the problem that you are having is mainly due to the fact that you are looping through every student in the array, so when you the input does not equal the currently iterated student's name, the if statement ends and the else statement runs, displaying the message that the student does not exist, it will do this several times until the code hits a name that is equal to the input.
Let's walk through what your compiler is doing to help illustrate the problem!
- Prompt the user for a name.
- The user prompted for the name 'Jody' -> Set to lowercase -> 'jody'
- Is this equal to the value null, or 'quit'
- No, it must be name then continue to if for loop
- The current variable i value is 0 -----> Compare to student array index value 0
- The student array value at index 0 is ---> "Dave" -> Set to lowercase -> 'dave'
- This value does not equal 'jody' --> evaluate the else statement instead
- Set the message variable equal the the string "Could not find student record"
- Run the function print() with a parameter of the variable message
After step 9 you will see the message Could Not Find Student Record displayed to the webpage. However, the compiler is not done. It will run through steps 5 - 9 again this time increment 0 to 1, and checking for the student array index 1, it will do this until it reaches the end of the array, or hits an exception. Hopefully you understand the mistake you made, and can think through a way to fix it. I will provide a solution below but I advise you not to look at it, unless you cannot figure out a solution yourself!
SOLUTION (well at least mine anyway...)
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentData(student) {
var data = '<h2>Student: ' + student.name + '</h2>';
data += '<p>Track: ' + student.track + '</p>';
data += '<p>Points: ' + student.points + '</p>';
data += '<p>Achievements: ' + student.achievements + '</p>';
return data;
}
/************************************************************
* Searches the an array of students for the provided name
* if the name exists then the index of the array at where
* the name was matched will be returned, otherwise it will
* return negative one.
*----------------------------------------------------------
* @param name A string containing a name
* @return An integer value pertaining the the index of the
* array at which the students name was matched, if no match
* is found then -1 is returned instead
************************************************************/
function search(name) {
//loop through the students array, when a name is matched
//return the value at which the name is found
for(var i = 0, j = students.length; i < j; i++) {
//We set the student name to lowercase for easier string comparing
if(students[i].name.toLowerCase() === name.toLowerCase()) {
alert("Found a name at index " + i);
return i;
}
}
//If no name is matched however, return -1
alert("The name specified was not found!");
return -1;
}
/************************************************************
* This function will prompt the user for a name, if the
* user enters nothing, quit, or a null, then the program
* will quit. Otherwise it will then proceed to run a search
* based on the name, if the search yields a value greater
* or equal to 0, then the program will display the
* contents of the student found at the student array index
* matching the value. If the search yields negative 1 then
* it will let the user know that the student does not exist.
************************************************************/
function runStudentReport() {
while(true) {
var studentName = prompt("Enter the name of the student you want to search for, if you want to quit the program at any time, please enter the value quit.");
//Make a check and see if the name is valid
if(studentName === null || studentName.toLowerCase() === 'quit' || studentName.toLowerCase() === "") {
//its invalid or the user quit, let then know then exit
alert("Looks like you either want to leave or entered nothing! Bye!");
break;
}
else {
var studentIndex = search(studentName);
if(studentIndex === -1) {
print("A student with that name does not exist!");
}
else {
print(getStudentData(students[studentIndex]));
}
}
}
}
runStudentReport()
The program does have some faults though...like if the user's name appears more than once, or if the user's name is a blank string.
For added challenge why don't you try to implement this in a way that it accepts multiple names, a hint is to use an array.
Also you can try to implement this where instead of replacing the html, it adds appends more html to the page, so that when you are done, you are left with a long page of search results!
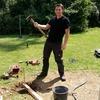
Nejc Vukovic
Full Stack JavaScript Techdegree Graduate 51,574 PointsHi.
About the "or" operator: it doesn't matter the way you write the conditions. They are both checked. So when either of them is TRUE than the condition is evaluated to true.
I looked the code you provided and it all normally runs in my WS.
What kind of error does the console throw when you run this?
And just for the reading check this LINK it describes what value you get when you cancel the prompt in the browser.
The value you get back depends on the browser.
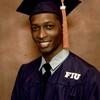
Dane Parchment
Treehouse Moderator 11,075 PointsI figured out his problem, it's a logic error, give me a moment to answer the prompt.

Jonathan Healy
21,601 PointsThank you both for your input. Dane, I had a feeling it was something along these lines but you breaking it down step by step is a great help to understanding my mistake. Also Nejc, I will try to reproduce the console error and report my findings soon. Thanks again
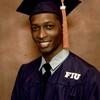
Dane Parchment
Treehouse Moderator 11,075 PointsGlad to have been of some help, and it's great that you understand the mistake you made!
Your original code is not faulty so no errors should actually occur in the console, though I could be wrong, didn't check it after all.
The type of error you ran into is what we call a logic error in computer science. These are the worst errors because they do not throw an exception or log any errors that make it easy to pinpoint and fix mistakes. These errors allow your program to compile correctly, but you do not get the desired outcome.
If you need anymore assistance I will be glad to assist you.