Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial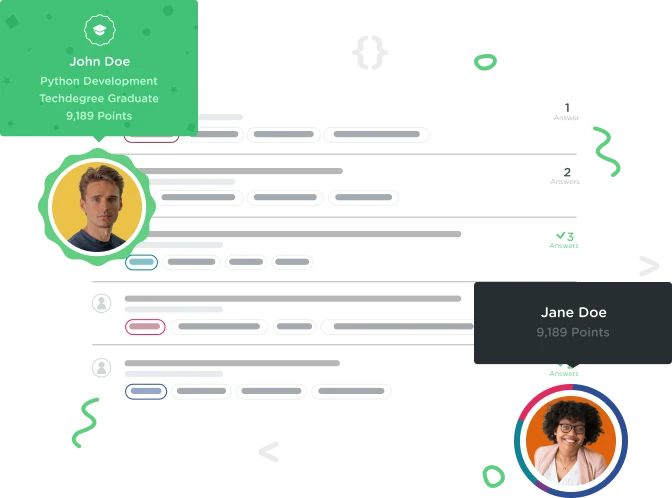

tytyty
4,974 PointsTuples Extra Credit Challenge
So I am depressed because I couldn't get this one on my own. It's not complete and here it is but I still have questions.
import UIKit
let inPlay = ["title": "Roar", "artist": "Katy Perry", "album": "Prism"]
func inspectTags(tags: [String: String]) -> (title: String,artist: String,album: String) {
return (tags["title"]!, tags["artist"]!, tags["album"]!)
}
let display = inspectTags(inPlay)
display.title
display.artist
display.album
println(display)
1.) I tried putting the let statement in the function as Amit has been doing in the videos but it wouldn't work. Why?
2.) Exclamation mark is the NOT operator so why is it here?
6 Answers
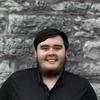
Michael Hulet
47,912 PointsThe reason you can't put the
let
line inside the function is because you're calling theinspectTags
function inside itself. This causes an infinite loop, and will crash your Playground (or whatever you're doing this in). This is called "recursion". This can be ok, but only if you provide some condition in the function where it will be run without it being called again from within itself, and this condition must be run relatively quickly. In your case, it looks like you want to call it outside the function, anyway.While the
!
is the negation operator, it is also the operator to explicitly unwrap optionals. When referencing an object in a Dictionary, it returns an optional, because the value at the index you specify could very easily benil
. Usually, the way you unwrap optionals is with an operation called "optional chaining", or "if let
syntax". An alternative and safer implementation of that function would look like this:
func inspectTags(tags: [String: String]) -> (title: String?, artist: String?, album: String?) {
var title: String?
var artist: String?
var album: String?
if let dictTitle = tags["title"]{
title = dictTitle
}
if let dictArtist = tags["artist"]{
artist = dictArtist
}
if let dictAlbum = tags["album"]{
album = dictAlbum
}
return (title: title, artist: artist, album: album)
}
In this scenario, the function returns optional strings, so you don't crash if any of the values at any of the referenced indexes of the passed-in Dictionary is nil

tytyty
4,974 PointsMan it looks great but it looks like your code also has code we didn't learn in the videos up to now or stuff we barely touched upon like "string?" and "let if". The code I copy and pasted also has code we didn't learn such as the ! to unwrap optionals.
Would it be possible for you to write the function using only what we learned up to this point? Did we use "let if"? I don't think so. My brain is wiped out.
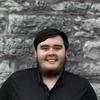
Michael Hulet
47,912 PointsAll of these things have to do with optionals, which you'll learn about in the next stage of the course you're taking. Unfortunately, you can't do much with a Dictionary
without learning about optionals, which seems like a massive oversight by the Treehouse staff, so unless you wanna totally ignore the Dictionary
that was passed in and return
arbitrary values in the named tuples, you should come back and try this after you finish the next stage

tytyty
4,974 PointsYeah Mike, it's weird. I feel like this particular Extra Credit Challenge was quite advanced compared to what we've learned up to now. The previous Extra Credit challenges I could use everything we'd been taught but it's not the case with this one. Maybe Extra Credit means we might have to research stuff we haven't been taught. I started the Optionals video and yes you're right, everything that was in the Extra Challenge is in this video lol Bizarre.
I also wish they had code for the eExtra Challenges since they can be quite involved. I woke up today thinking I'd get through many courses but instead spent the entire day on the one Extra Challenge. I won't be doing that again. After 1 hour I'll just post away in the forums instead of wasting 8 hours lol
Thanks for your help!
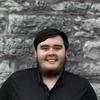
Michael Hulet
47,912 PointsI think the Extra Credit courses are often designed to push your knowledge on the subject, and oftentimes force you to research a topic before it's taught, the theory being that you'll learn it better by immediately jumping right in and doing it. Because of the time it takes to do each one, I oftentimes skipped them, but one of them from the Objective-C track ended up becoming the App I have on the App Store

tytyty
4,974 PointsAnother question re: the extra challenge. The challenge said "//Write a function that accepts a Dictionary as parameter and returns a named tuple. The dictionary should contain the following keys: title, artist and album."
Are we to assume right from the get-go that the dict would be declared outside/before the function? This is a no-brainer for experienced programmers?
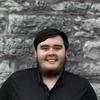
Michael Hulet
47,912 PointsYes to both. Because the function accepts the Dictionary
as a parameter, it is safe to assume it was created outside of the function, or passed in as a literal. Remember the "recursion" stuff I talked about earlier? It's possible that a Dictionary
could be created within a function, which is then passed as a parameter when the function calls itself recursively, but this is extremely dangerous, and therefore very seldom used. This kind of logic becomes second nature, when you get to know the language a little better than you do now. Give it a few hours more, and you'll be a pro in no time, I bet

tytyty
4,974 PointsThanks Mike. Nice looking app btw. Congrats! :)
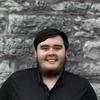
Michael Hulet
47,912 PointsIt's not problem. Thanks!

Marie Thomasson
1,103 PointsNice app Mike! Makes me excited to get to that point!!!
One helpful pointer I wish Treehouse would point out is that the extra credit from arrays and dictionaries helps alot to Michael's point above about declaring/defining the dictionary first, separate from the function. Got that working, but still confused about the rest!!
I'm going to watch the next section and then come back to this challenge. I think I may be spinning my wheels.