Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial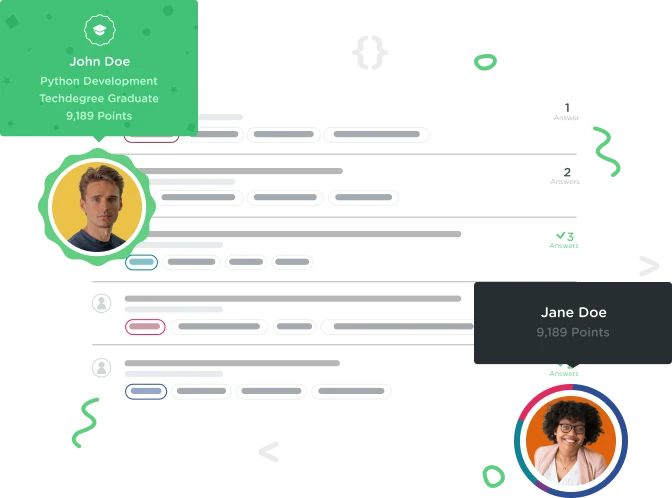

thomas Sichel
8,992 Pointstuplets
I really don't understand what this question is asking me to do... could you possibly send me a walk-throuigh on how to do task 1 to 3? thanks
func greeting(person: String) -> String {
let language = "English"
let greeting = "Hello \(person)"
return greeting
}
1 Answer

Alvin Abia
Courses Plus Student 23,034 PointsSo this is the code provided at the start of the code challenge.
func greeting(person: String) -> String {
let language = "English"
let greeting = "Hello \(person)"
return greeting
}
The first task asks you to change the return value of this function so that it returns both the greeting and language constants as a tuple, with each item in the tuple specifically named 'greeting', and 'language'. You basically need to just alter your function so that it fulfills the above rather than the single string as it originally did. Remember that tuples need to be encapsulated in parenthesis, so it would look like this:
func greeting(person: String) -> (greeting: String,language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting,language)
}
The second task asks you to create a variable named result and assign it to the tuple returned from the above function using the string "Tom" as its parameter. This would look like this:
var result = greeting("Tom")
The third task asks you to use the println statement to print out the value of the language element from the result tuple. You can access specific elements of a tuple using dot notation. This would look like this:
println(result.language)
I know that the concept of tuples is a slightly confusing one, so I hope this helps. With a little practice, you'll definitely master it and you'll really love just how much functionality tuples provide in the Swift language. Good luck!
Eric Anderson
6,608 PointsEric Anderson
6,608 PointsThank you for explaining and providing a walk through Alvin, it was very helpful!
Michael Pastran
4,727 PointsMichael Pastran
4,727 PointsThanks Alvin, i was getting really discouraged by this section. I wish there were more challenges. 3 exercises doesnt really give you an understanding of Tuples. Thank you btw i hope with a little more practice i will get it
Alvin Abia
Courses Plus Student 23,034 PointsAlvin Abia
Courses Plus Student 23,034 PointsAnytime guys, glad I could help! Feel free to contact me if there's anything else you guys come across in Swift that I could elaborate for you.
Michael Pastran
4,727 PointsMichael Pastran
4,727 PointsHey Alvin, do you know if there is a section of the site where you can do more examples. so for like Tuples, is there another set of challenges you can do? i just want to get more practice in so i can get a clearer understanding through practice. thanks
Alvin Abia
Courses Plus Student 23,034 PointsAlvin Abia
Courses Plus Student 23,034 PointsHey Michael, I am not actually sure if there is a section within the website that provides extra examples. However, what I found to help when I came across a concept particularly difficult was to: 1) Recreate it in a separate Xcode playground, while looking at the code challenge line to line and explain to myself the purpose of each piece of code (I have a load of playgrounds with each line annotated with comments explaining what everything did which really helped me in future projects where I may have forgotten something about a certain concept like tuples for example). 2) Recreate it again in another separate playground without looking at the code challenge (So try creating your own function that returned a tuple. 3) Start toying around with the concept (try adding more than 2 components to a tuple, or try adding other data types other than just Strings).
I found this process to be really helpful for myself because it allowed me to have a bunch of annotated code to refer back to whenever I needed to, and you'd be suprised at how quickly something will click for you when you explain it to yourself line by line and then experimenting with it. Good luck bud!
Michael Pastran
4,727 PointsMichael Pastran
4,727 PointsThanks Alvin, really. you're really helpful. i just started learning like a week ago and i've completely falling in love with it. im doing a study blue too to keep as much notes as i can. i will deft add more comments to my source code.