Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial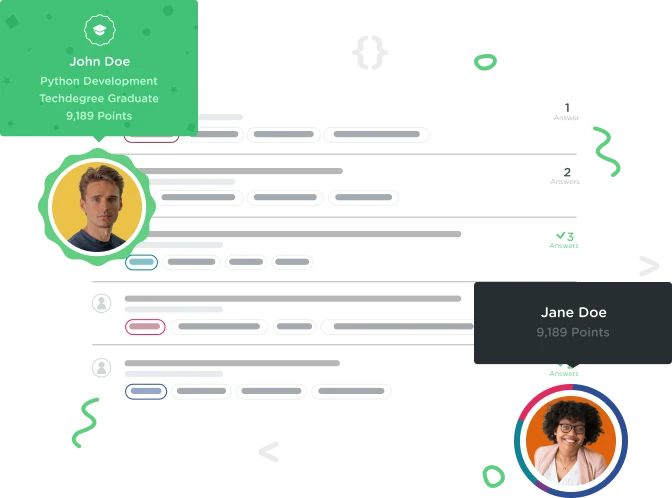

Andrew Gordon
10,178 Pointsturning a string in a dictionary
I'm not real sure what the issue is with my code. I understand that these exercises look for something specific to be returned from the function, but I don't get why this isn't working.
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
def word_count(x):
x = "I am that I am".lower()
count = 0
word_dict = {}
for y in x:
count += 1
word_dict[y] = count
if y in word_dict:
break
return word_dict
2 Answers
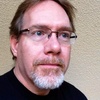
Chris Freeman
Treehouse Moderator 68,423 PointsAs Jeffrey mentioned, the function needs to accept any input string. The other missing part is using split()
to break the string into a list of words. Indentation increased to 4 spaces
def word_count(x):
xlower = x.lower() #<-- lower case input string
wordlist = xlower.split() #<-- split string into list of words
count = 0
word_dict = {}
for y in wordlist:
# Check if word already seen
if y in word_dict:
# Add to word count
count += 1
word_dict[y] += 1
else:
# Add new word to dict
word_dict[y] = 1
return word_dict
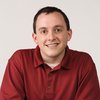
Jeffrey Ruder
6,408 PointsA few things about your code look off to me.
First, I would suggest changing the first part of the function so that you're not using a specific string. I'm pretty sure "I am that I am" is just an example. You might get test cases that use other strings.
Change it to something like:
def word_count(thestring):
word_dict = {}
lowercasestring = thestring.lower()
for word in lowercasestring:
#and then do stuff
Second, I'm not sure that your count variable is going to do what you want. It looks like it increases with every pass through the for loop regardless of whether the word is already in the dictionary.
Third, it looks to me like you're using break in the for loop incorrectly. I think the way you're using it means that the for loop will stop the first time it encounters a duplicate word. I don't think that's what you want. Maybe you meant to use continue instead.