Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial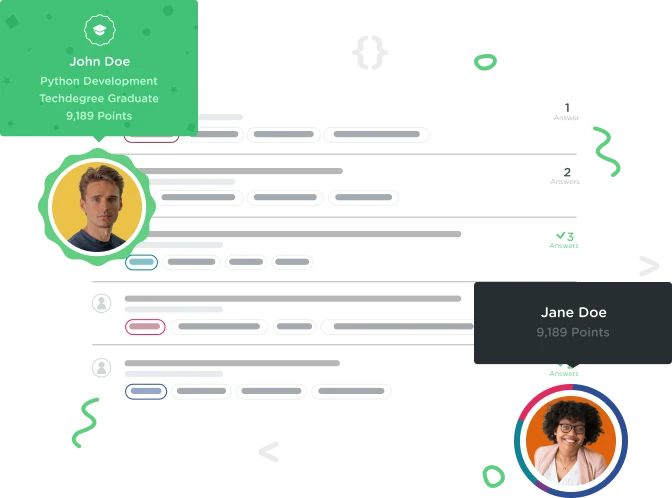

Daniel McCann
Courses Plus Student 5,308 PointsTurning students into their own div
Instead of Just outputting the students as heading and paragraphs I have been trying to change the for loop to first create a new div then add each piece of information to it's appropriate new div. I get output but it is tripling my results and they are not in the proper order. I tried stepping through each line with the debugger but all I have been able to tell is that my loop creates the divs properly, but adds all of the previous info in the message variable into the current div. So the first div is correct, but the second div has info for students 1 & 2, thirs div has info for students 1, 2 & 3 etc.
var message = '';
var student;
var newDiv;
for ( i = 0; i < students.length; i++ ) {
student = students[i];
newDiv = document.createElement('div');
newDiv.className = student.name;
newDiv.innerHTML = message;
message += '<h2><strong>Name: ' + student.name + '</strong></h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
document.getElementById('output').appendChild(newDiv);
}
and I just used the students array that was provided in the separate javascript file
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
2 Answers
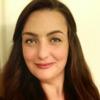
Jennifer Nordell
Treehouse TeacherHi there, Daniel McCann ! You're doing great and I applaud your willingness to experiment and you're much closer than you think (I'd be willing to bet). A lot of this comes down to placement. You start the message
variable as an empty string, which is smart otherwise there can be issues with concatenation down the line. You do this outside the loop. So the first time through the loop, it takes the first student and adds that to the message
variable. And the second time through the loop, it adds the second student to that message
. But remember, you never cleared out the message variable! It still has the first student and now it had the first plus the second student. The third time through the loop it will add the third student onto the first and second (and so on).
I believe that this can all be fixed by moving this line to be the first line inside your for
loop:
var message = '';
Hope this helps!

Daniel McCann
Courses Plus Student 5,308 PointsThank you! That makes a lot more sense.
Daniel McCann
Courses Plus Student 5,308 PointsDaniel McCann
Courses Plus Student 5,308 PointsBecause of Scope!!! of course! Thanks so much! That makes sense. When I swap the positioning of that and move the var declaration into the first line of the code block, however, the page doesn't seem to run automatically anymore. Do I need to change this to a do while loop or am I just missing something in my for loop?
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherDaniel McCann Woops! I forgot to add another hint about placement in my original answer. Assuming you moved the
message
declaration to the first line of that loop, you will also need to move the part where you set theinnerHTML
property until after you've done your concatenation. Otherwise, you're setting theinnerHTML
to an empty string. Take a look at the endingfor
loop.