Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial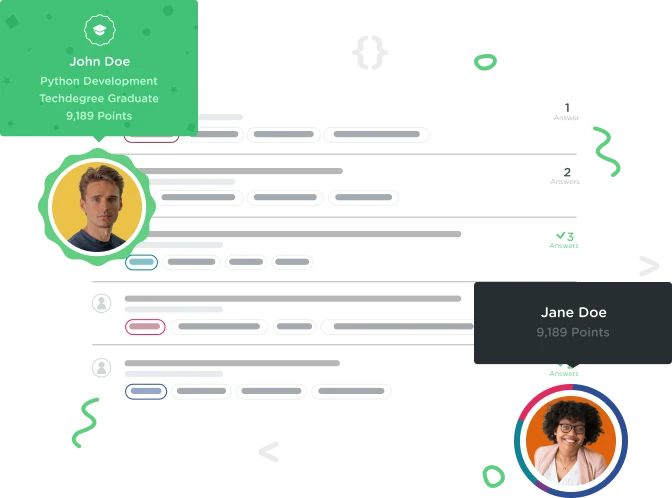

Youssef Subehi
Courses Plus Student 290 PointsTutorial: Adding Search to Ribbit App
hello From Jordan Country, My Name is Youssef and im Really happy to be a member at Tree House it's really amazing Place to lean in easy and helpful way.
im Here in first place to learn Android and i just reached step 4 Relation Users in Parse and look how i did it
i learned how to user Parse query's and Also how i make it work inside android app.
ok since @Ben Jakuben forget to show us how to implement search inside Ribbit app i'll show you how easy it is after watching the first 4 parts only
ok First we need to put some text box and button inside the edit_friends.xml
and the image will look like this:
Don't Forget to give it a Hint and Id for both of them,
IF YOU WANT TO FOLLOW ALONG WITH ME HERE IS WHAT I USE
<EditText
android:id="@+id/searchFriend"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:ems="10"
android:hint="@string/search_friend_hint" >
<requestFocus />
</EditText>
<Button
android:id="@+id/searchButton"
style="?android:attr/buttonStyleSmall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_toRightOf="@+id/searchFriend"
android:text="@string/search_button" />
you will notice that i use searchField as id for the text entry and the searchButton as id for the button
now we are done with the layout lets go back to the EditFriendsActivity.java
it's very easy there is 3 steps you need to do
*the first one is *
add Protected values to the top of the file these values to define them inside the EditFriendsActivity
protected EditText sUsername;
protected Button mSearchButton;
The Second One
exactly after protected void onResume() {
add these values also
sUsername = (EditText)findViewById(R.id.searchFriend);
mSearchButton = (Button)findViewById(R.id.searchButton);
Now you are done, you just need one more step to go, i highly Recommended you to view this documentation in parse Parse.Com Documentation
what i did is i created listener when the user click on the search button it will take he value from the search box and pass it to the Parse query and the parse will return the value
also i make sure it will return it if it's a friend or not
here is the Full Code of the page EditFriendsActivity.java
the names may not be the same as your project is but i think it's very easy to change them with your data names
in the code you will see some comments and you will see a BIG comment for the new method and the old method
package com.twaa9l.isnap;
import java.util.List;
import android.app.AlertDialog;
import android.app.ListActivity;
import android.os.Bundle;
import android.support.v4.app.NavUtils;
import android.util.Log;
import android.view.MenuItem;
import android.view.View;
import android.view.Window;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseQuery;
import com.parse.ParseRelation;
import com.parse.ParseUser;
import com.parse.SaveCallback;
public class EditFriendsActivity extends ListActivity {
public static final String TAG = EditFriendsActivity.class.getSimpleName();
protected List<ParseUser> mUsers;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected EditText sUsername;
protected Button mSearchButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_edit_friends);
// Show the Up button in the action bar.
setupActionBar();
getListView().setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
}
@Override
protected void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
sUsername = (EditText)findViewById(R.id.searchFriend);
mSearchButton = (Button)findViewById(R.id.searchButton);
///////////////////////////////////////////////////////////////////////////////////////////////////////////
//////////////The New Code for Search Users by Username///////////////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
mSearchButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//Get text from each field in register
String username = sUsername.getText().toString();
//String password = mPassword.getText().toString();
///Remove white spaces from any field
/// and make sure they are not empty
username = username.trim();
//password = password.trim();
//Check if fields not empty
if(username.isEmpty()){
AlertDialog.Builder builder = new AlertDialog.Builder(EditFriendsActivity.this);
builder.setMessage(R.string.login_error_message)
.setTitle(R.string.login_error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
else{
//Login User
setProgressBarIndeterminateVisibility(true);
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.whereEqualTo("username", username);
query.orderByAscending(ParseConstants.KEY_USERNAME);
query.setLimit(200);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> users, ParseException e) {
setProgressBarIndeterminateVisibility(false);
if(e == null){
//Success we have Users to display
//Get users match us
mUsers = users;
//store users in array
String[] usernames = new String[mUsers.size()];
//Loop Users
int i = 0;
for(ParseUser user : mUsers){
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
EditFriendsActivity.this,
android.R.layout.simple_list_item_checked,
usernames
);
setListAdapter(adapter);
addFriendCheckmarks();
}
else{
//No Users to Display
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(EditFriendsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
});
}
///////////////////////////////////////////////////////////////////////////////////////////////////////////
//////////////The Old Code for will Get all Users From The Parse//////////////////////////////////////////
/////////////////////////////////////////////////////////////////////////////////////////////////////////
/*
//Search for users query in Parse.com
setProgressBarIndeterminateVisibility(true);
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.orderByAscending(ParseConstants.KEY_USERNAME);
query.setLimit(200);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> users, ParseException e) {
setProgressBarIndeterminateVisibility(false);
if(e == null){
//Success we have Users to display
//Get users match us
mUsers = users;
//store users in array
String[] usernames = new String[mUsers.size()];
//Loop Users
int i = 0;
for(ParseUser user : mUsers){
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
EditFriendsActivity.this,
android.R.layout.simple_list_item_checked,
usernames
);
setListAdapter(adapter);
addFriendCheckmarks();
}
else{
//No Users to Display
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(EditFriendsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}*/
/**
* Set up the {@link android.app.ActionBar}.
*/
private void setupActionBar() {
getActionBar().setDisplayHomeAsUpEnabled(true);
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case android.R.id.home:
// This ID represents the Home or Up button. In the case of this
// activity, the Up button is shown. Use NavUtils to allow users
// to navigate up one level in the application structure. For
// more details, see the Navigation pattern on Android Design:
//
// http://developer.android.com/design/patterns/navigation.html#up-vs-back
//
NavUtils.navigateUpFromSameTask(this);
return true;
}
return super.onOptionsItemSelected(item);
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
if(getListView().isItemChecked(position)){
//Add Friends
mFriendsRelation.add(mUsers.get(position));
}
else{
//Remove Friend
mFriendsRelation.remove(mUsers.get(position));
}
mCurrentUser.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if(e != null){
Log.e(TAG, e.getMessage());
}
}
});
}
private void addFriendCheckmarks(){
mFriendsRelation.getQuery().findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
if(e == null){
//List Returned - look for a match
for(int i = 0; i < mUsers.size(); i++){
ParseUser user = mUsers.get(i);
for(ParseUser friend : friends){
if(friend.getObjectId().equals(user.getObjectId())){
//we have a match
getListView().setItemChecked(i, true);
}
}
}
}
else{
Log.e(TAG, e.getMessage());
}
}
});
}
}
6 Answers

Martin Oliva
Courses Plus Student 593 PointsGreat work! Thank you for sharing.

Youssef Subehi
Courses Plus Student 290 Pointsyou are welcome

Youssef Subehi
Courses Plus Student 290 Pointsyou are welcome,
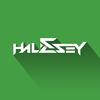
Liam Hales
356 Pointshey dude, thanks for posting this, i have done everything in your tutorial but when click on "edit friends" when running the app, the app quits and says "unfortunately (my app name) has stopped".
Can you explain why this would occur?
Many thanks for your help!

Sam smith
Courses Plus Student 59 PointsMy Alert box does not pop up telling them that there were no results..
mSearchFriendsButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
mSeachProgressBar.setVisibility(View.VISIBLE);
mUsernames = mSearchFriendsBar.getText().toString();
mUsernames.trim();
if(mUsernames.isEmpty()){
//Display an error
AlertDialog.Builder builder = new AlertDialog.Builder(EditFriendsActivity.this);
builder.setTitle(R.string.signup_error_title)
.setMessage(R.string.signup_alert_message)
.setPositiveButton(R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
else {
//success! find the users
ParseQuery<ParseUser> query = ParseUser.getQuery();
query.whereEqualTo(ParseConstants.KEY_USERNAME, mUsernames);
query.orderByAscending(ParseConstants.KEY_USERNAME);
query.setLimit(100);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> users, ParseException e) {
if(e == null){
mSeachProgressBar.setVisibility(View.GONE);
//Success
mUsers = users;
String[] usernames = new String[mUsers.size()];
int i = 0;
for(ParseUser user : mUsers){
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(EditFriendsActivity.this, android.R.layout.simple_list_item_checked, usernames);
setListAdapter(adapter);
}
else{
// Alert box
AlertDialog.Builder builder = new AlertDialog.Builder(EditFriendsActivity.this);
builder.setTitle(R.string.signup_error_title)
.setMessage(R.string.no_searches_found)
.setPositiveButton(R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}

vinay mishra
Courses Plus Student 264 PointsYeah g8t work broda :)

vinay mishra
Courses Plus Student 264 PointsInstead of self destruction, I want to store the data like whatsapp so how can I achieve this? :)
dev Manzo
38 Pointsdev Manzo
38 PointsThank you so much!