Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial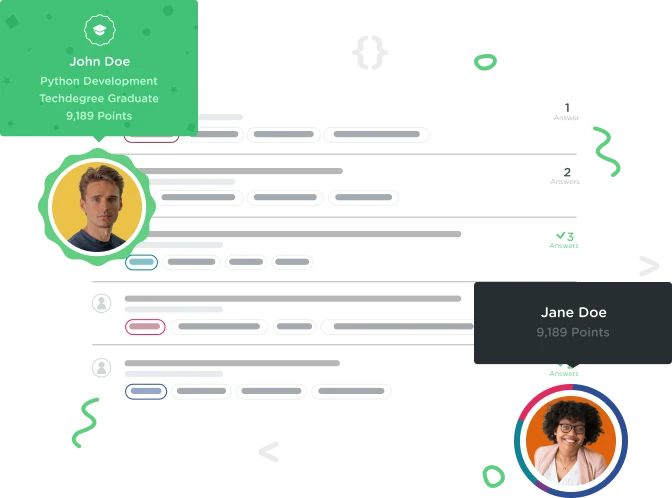
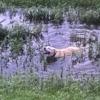
Kyle Vassella
9,033 PointsTweak .indexOf() to make 'Grocery List' program more user friendly?
Dave had us construct a program using Arrays, Conditional Statements and Array Methods to have a prompt come up that allows users to check the stock of a grocery store. Code looks like this:
var inStock = [ 'apples', 'eggs', 'milk', 'cookies', 'cheese', 'bread', 'lettuce', 'carrot', 'broccoli', 'pizza', 'potato', 'crackers', 'onion', 'tofu', 'frozen dinner', 'cucumber'];
var search;
function print(message) {
document.write( '<p>' + message + '</p>');
}
while ( true ) {
search = prompt("Search for a product in our store. Type 'list' to show all of the produce and 'quit' to exit");
search = search.toLowerCase();
if (search === 'quit' ) {
break;
} else if (search === 'list' ) {
print(inStock.join(', '));
} else {
if ( inStock.indexOf( search ) > -1 ) {
print( 'Yes, we have ' + search + ' in stock.');
} else {
print ("No, we don't have " + search + " in stock.");
}
}
}
At the end of this video he inserts the search = search.toLowerCase(); statement to make the program more user friendly: in case the user typed their responses in all or mixed caps, the program would still list those items as 'in stock' as long as the spelling is the same as something in the array.
For fun I'd like to further this - so that in case a user types a plural form of an item which is listed as singular in the array - or vice versa - the program would still give the 'correct' response. So if the user typed carrots, the program will still respond that the item (listed as 'carrot' in the array) is in stock. Besides adding 'carrots' (plural) to the array, is there better way to do this?
1 Answer
John Coolidge
12,614 PointsThe easiest method that comes to my mind is to ask users to search for either the singular or plural form. This way you don't have to alter your array (except to make sure that all items are singular or plural) and you don't have to add tedious amounts of code that check the user input for plurals (of which in English, there are many forms) and snip off the end of that plural or some such similar bit of code.
I don't want to be the guy that has to put that code together. You can't just ask to take the 's's off the end. What about words like "peaches" with requires the removal of an 'es'. But wait, if you remove the 'es' what about words like "cheeses"? Take off the 'es' and you now have 'chees'. That might be overly complicated for what you want to do.
john larson
16,594 Pointsjohn larson
16,594 PointsI agree for this lesson that would be overly complicated. However, I did see someone else address this type of thing. They had a clever response using indexOf() targeting the last letter doing something I didn't quite get. It seemed like they understood what they were doing but I didn't have the emotional or mental energy at the time to absorb it. Maybe you know?