Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial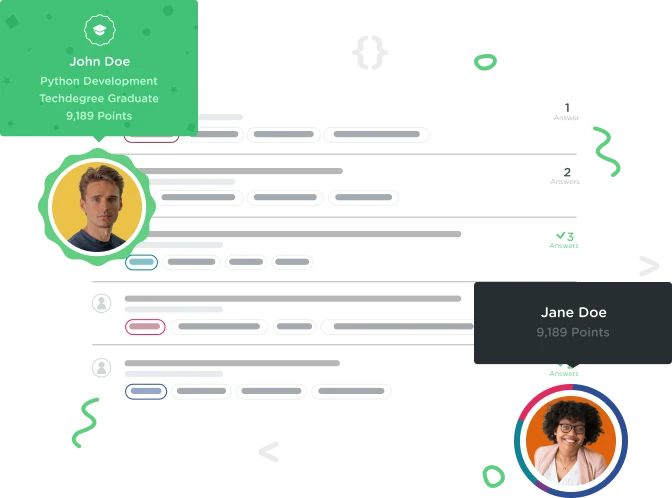
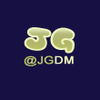
Jonathan Grieve
Treehouse Moderator 91,254 PointsTweak to the "Sup" Java FX App
Hi all,
So I made a little tweak to the JavaFX app. Just a simple if statement that attempts to force the user to add something to the text field. Only no matter what I try, i always get the same (succesful) message that would come if something was typed.
Here's my code, any ideas? Thanks :)
package sample;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Group;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) throws Exception{
//Parent root = FXMLLoader.load(getClass().getResource("sample.fxml"));
Group root = new Group();
//Set up new child nodes
Text txt = new Text("Hello I'm a JavaFX Text node");
TextField userName = new TextField();
VBox box = new VBox();
Button btn = new Button();
//run methods on nodes
btn.setText("Click Me!");
if(userName.getText() == " ") {
btn.setOnAction(evt -> System.out.printf("Error: You need to enter something in this box for this program to work"));
} else {
btn.setOnAction(evt -> System.out.printf("Good job, %s. Sup was clicked%n", userName.getText()));
}
root.getChildren().add(box);
box.getChildren().addAll(txt, userName, btn); //new layout
txt.setY(50); //place text node.
primaryStage.setTitle("Whaddup, Java");
primaryStage.setScene(new Scene(root, 300, 275));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
2 Answers
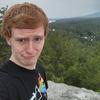
Brendon Butler
4,254 PointsFrom the looks of things, you have a space character inside this if statement
if(userName.getText() == " ");
this means that the user would have to type the space character into the TextField. If you changed that, your code might work, but it might not as well.
Whenever comparing Strings you should use ".equals()" or ".equalsIgnoreCase()" instead of "==". I don't know the exact reasoning behind it. I know that about half of the time, it could cause an issue.
Your new if statement should look like this
if (userName.getText().equals("");
I also took a look at the source code for the getText() method. And it looks like it is possible to return null.
So another possible if statement could be
if (userName.getText() == null);
EDIT: If you want to include the space character in your check, (so people can't have a space as their name) use this
if (userName.getText().trim().equals(""));
Hopefully this helps. Happy coding! :)

Boban Talevski
24,793 PointsIs it really working though? Tried to do the same thing, but it seems that if check is done only once when the application starts and at the time the text field is empty, so the button action is set according to the empty text field and there's no way to change it. Even if you write something inside the text field, the action is already set when the app was initialized, a button click isn't going to change the action, it will always print whatever you set it out to print if the name field was empty.
The if check probably needs to be done inside the button action. We need to use the longer lambda form, i.e., not a one liner. Also, this condition seems to work for both an empty field and one or more spaces, which is just a basic check for something entered in the field. This is what I'm talking about.
btn.setOnAction(event -> {
if ((nameFld.getText() + " ").trim().equals("")){
System.out.println("Error: You need to enter something in this box for this program to work");
} else {
System.out.printf("Sup %s! %n", nameFld.getText());
}
});
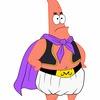
<noob />
17,063 PointsBoban Talevski your right Jonhathn's code didnt worked for the reason u mentioned. the if check needs to be inside the event handler and we have to use the longer lmabda form, which in my opnion is better!
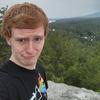
Brendon Butler
4,254 PointsWhy would you add a space to the nameFld variable, only to trim it on the same line? That makes no sense at all.

Boban Talevski
24,793 PointsBrendon Butler I really don't remember why I added the space, it was a while ago :).
I assume it was just to be safe from null pointer exceptions. Keep in mind I have no idea what this app was and I have not worked on any code for a Java desktop app since the time I completed this course.
I don't know what getText
will return if there's no text in the field. Maybe it'll return null and calling trim on a null object will probably raise a null pointer exception? That's why I'm probably adding the " "
before calling trim. I could probably achieve the same by adding ""
, but then there's a potential problem (less likely I guess) that calling trim on an empty String (in case getText returned null and I added only ""
) might give some unexpected results. And I'm sure that calling trim on " "
will return the empty string ""
which is what I'm checking equality against, and adding the " "
instead of ""
doesn't change the result of the equals check, but keeps the potential problems away.
So yeah, guess I was just trying to be on the safe side, or well... too lazy to check the docs :).
Jonathan Grieve
Treehouse Moderator 91,254 PointsJonathan Grieve
Treehouse Moderator 91,254 PointsHi Brendon.
I did try using null in my expression before but that didn't seem to work.
I've since gone ahead and used the equals method as you suggested and got it to work using this...
I like that you can include any number of spaces and still show up the error so text is required :)
Thanks