Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial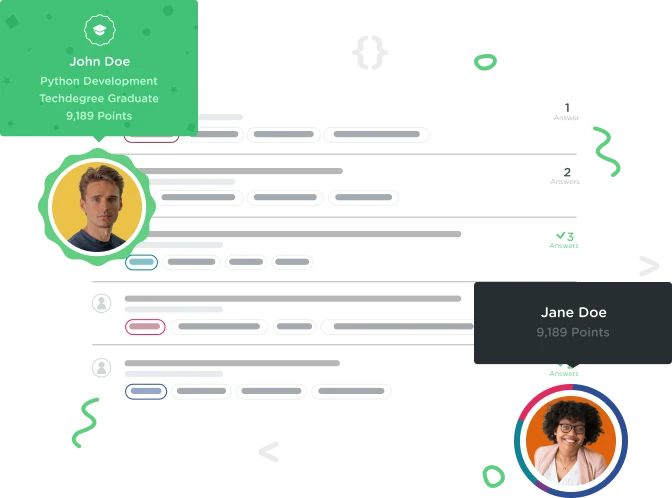
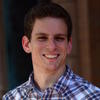
Jeff Lange
8,788 PointsTwo dimensional arrays: how do I access just one array of data at a time?
As part of an exercise, I'm creating a program that can calculate the side lengths of a triangle based on user-inputted coordinates. As I take in the measurements of each side (using a loop) I'm storing the values in an array. Thus, if the user inputs information for three different triangles, I'll end up with an array looking something like this:
[[3, 3, 3,], [4, 5, 6], [3, 4, 5]]
Hence, an array of three arrays, each array containing the three lengths of a triangle's sides. My question is, let's say I want to access just the values for the second triangle's array (i.e. [4, 5, 6]), to do something like calculate the perimeter (i.e. 4 + 5+ 6 = 15), how would I grab that array's, and only that array's, values?
Further, how would I tell the program to iterate through one array at a time, making necessary calculations (perimeter, area, etc), and then moving on to the next array, before finally stopping when it's gotten to the end?
Thanks!
2 Answers
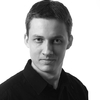
Maciej Czuchnowski
36,441 PointsOK, so let's say you are storing this whole array of arrays in variable a
:
a = [[3, 3, 3], [4, 5, 6], [3, 4, 5]]
You can access individual arrays inside this way (remember, we start counting from 0, so index 0 is the first element):
a[0]
^ this would give you [3, 3, 3]
a[1]
^ this would give you [4, 5, 6]
, etc.
You access individual values using two indexes - first one is the index of the array, and the second - the index of the value inside that array, so:
a[1][0]
^ this gives you value 4 (second array, first element, counting from 0)
If you want to iterate through all elements of each array, you just need to make two loops, one inside another, something like this:
a.each { |ary|
ary.each { |num|
// your code
}
}
ary
is each of the arrays, one after another and num
is each number inside the ary
array that you are going through at the moment.
More documentation and stuff:
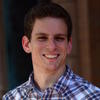
Jeff Lange
8,788 PointsAwesome! That was super helpful. Thank you!
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsSo as far as your last question goes, it would be something like this:
a.each { |ary| puts (ary[0] + ary[1] + ary[2]) }
^ this would print the perimeter of each triangle, one at a time.