Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial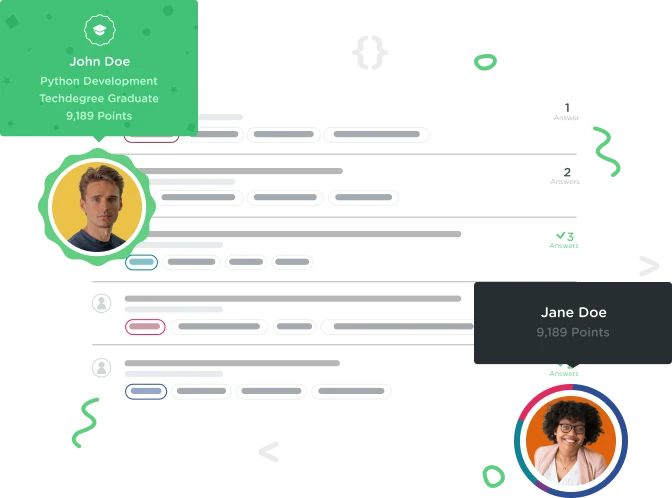

Andrew McLane
3,385 Pointstwoples.py
Am lost on this problem. I don't understand how *args works at all. I even tried just adding the arguments into a list using a blank list and it doesn't work. I guess I just have a fundamental misunderstanding of how to use this, because I thought using a for loop it would be simple to add all the arguments into a list.
1 Answer
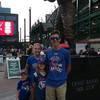
AJ Salmon
5,675 PointsHey Andrew! This can be frustrating, for sure. Here's how I did this challenge:
First, defining the function and the parameter(s). With the *args pattern, you're telling python to take any and all arguments passed to a function and put them into a tuple. The key opperand here is the star. It's convention to use the name args after the star, though. So, here's what it looks like:
def multiply(*args):
And here's what it looks like behind the scenes when you pass it some arguments.
>>> multiply(1, 3, 5, 7, 8)
#*args takes these numbers and puts them in a tuple, so
args = (1, 3, 5, 7, 8)
>>>multiply('string', ['list', 'of', 'whatever'], 7)
#again, all of the arguments, whether it's one or one thousand, is put in a tuple
args = ('string', ['list', 'of', 'whatever'], 7)
So now that the function takes any number of arguments, and puts them into a tuple, we can loop through the tuple with a for loop. First, though, we should create a number variable that we can use as a base for our final value. Since we're multiplying, I set that variable to 1.
def multiply(*args):
num = 1
for arg in args:
num *= arg
return num
This makes it so that the first arg in the for loop is multiplied by 1, and then each number after that is multiplied by the product of the previous numbers. Again, args is just the conventional name. This could be rewritten like this and be the exact same thing:
def multiply(*nums):
num = 1
for thing in nums:
num *= thing
return num
It's the asterisk that matters. Hopefully this clears things up a bit, if not, feel free to ask any questions. Happy coding! :)
Elgene Ee
3,554 PointsElgene Ee
3,554 PointsHi...I am just wondering can I call you legend? The explanation is really on point and help me clear things up lil bit. Anyway, thanks!