Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial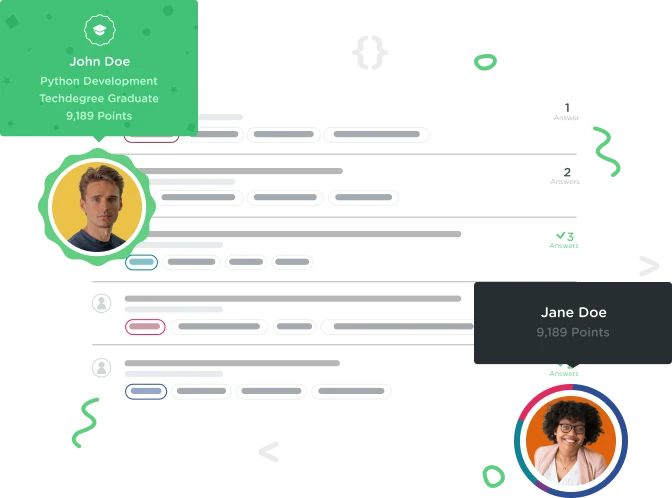

Stewart Mandla Kohlisa
11,737 Pointstyd this code so many times but um getting nothing,whats wrong with my code or there are bugs in this code challenge?
using Treehouse.Models;
namespace Treehouse.Data { public class VideoGamesRepository { // TODO Add GetVideoGames method public VideoGame[] GetVideoGames(int id) { VideoGame[] videoToReturn = null;
foreach (var _videoGame in _videoGames)
{
if(_videoGame.Id==id)
{
videoToReturn = _videoGame;
break;
}
}
return _videoGames;
}
// TODO Add GetVideoGame method
private static VideoGame[] _videoGames = new VideoGame[]
{
new VideoGame()
{
Id = 1,
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = true
},
new VideoGame()
{
Id = 2,
Title = "Mario Kart 64",
Description = "Mario Kart 64 is a 1996 go-kart racing game developed and published by Nintendo for the Nintendo 64 video game console.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = false
}
};
}
}
using Treehouse.Models;
namespace Treehouse.Data
{
public class VideoGamesRepository
{
// TODO Add GetVideoGames method
public VideoGame GetVideoGames(int id)
{
VideoGame videoToReturn = null;
foreach (var _videoGame in _videoGames)
{
if(_videoGame.Id==id)
{
videoToReturn = _videoGame;
break;
}
}
return _videoToReturn;
}
// TODO Add GetVideoGame method
private static VideoGame[] _videoGames = new VideoGame[]
{
new VideoGame()
{
Id = 1,
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = true
},
new VideoGame()
{
Id = 2,
Title = "Mario Kart 64",
Description = "Mario Kart 64 is a 1996 go-kart racing game developed and published by Nintendo for the Nintendo 64 video game console.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = false
}
};
}
}
namespace Treehouse.Models
{
// Don't make any changes to this class!
public class VideoGame
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public string[] Characters { get; set; }
public string Publisher { get; set; }
public bool Favorite { get; set; }
public string DisplayText
{
get
{
return Title + " (" + Publisher + ")";
}
}
}
}
5 Answers

Marius Tørseth
2,623 PointsIt passes for me, I'm seeing that the website didn't pickup all the code i wrote and placed it in the "box" (Fixed it now)
However, Here is the entire script:
using Treehouse.Models;
namespace Treehouse.Data
{
public class VideoGamesRepository
{
// TODO Add GetVideoGames method
public static VideoGame[] GetVideoGames()
{
return _videoGames;
}
// TODO Add GetVideoGame method
public static VideoGame GetVideoGame(int id)
{
VideoGame videoToReturn = null;
foreach (var _videoGame in _videoGames)
{
if (_videoGame.Id == id)
{
videoToReturn = _videoGame;
break;
}
}
return videoToReturn;
}
private static VideoGame[] _videoGames = new VideoGame[]
{
new VideoGame()
{
Id = 1,
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = true
},
new VideoGame()
{
Id = 2,
Title = "Mario Kart 64",
Description = "Mario Kart 64 is a 1996 go-kart racing game developed and published by Nintendo for the Nintendo 64 video game console.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = false
}
};
}
}

Marius Tørseth
2,623 PointsHello Stewart,
It seems like you are missing a Method.
In the challenge it states that you need to create a "GetVideoGames" method and a "GetVideoGame" method
You've almost got it right,
The GetVideoGames method should look like:
public static VideoGame[] GetVideoGames()
{
return _videoGames;
}
Notice the square brackets, we are returning an array
There is one issue with your "GetVideoGame" method, at the end of it, you are returning "_videoToReturn" but it doesn't exist in the current context, but "videoToReturn" does, since its created at the start of the method and then later set in the foreach.
It should look like this:
public static VideoGame GetVideoGame(int id)
{
VideoGame videoToReturn = null;
foreach (var _videoGame in _videoGames)
{
if (_videoGame.Id == id)
{
videoToReturn = _videoGame;
break;
}
}
return videoToReturn;
}
No need for square brackets on the return type on this method because we are just comparing which ID has been input and returning the instance matching that ID..
Hope this helps, please tell me if something is wrong or not working like it should

Stewart Mandla Kohlisa
11,737 Pointsits not passing Marius

Stewart Mandla Kohlisa
11,737 Pointsthanks Marius Tørseth it has passed found where i was making the mistake

Marius Tørseth
2,623 PointsHappy to help :)