Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial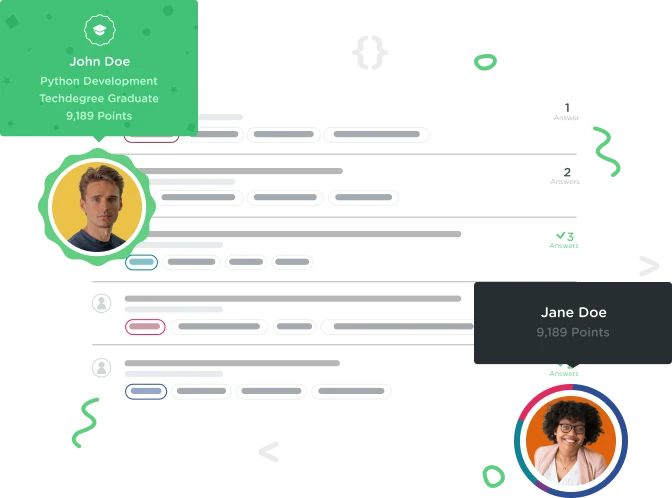

David Orlow
Courses Plus Student 1,333 PointsType casting, what am I doing wrong?
So, the project is asking me to take a string of whatever and feed it into an integer for the answer to life. I cannot get it to compile within TreeHouse. But, I downloaded Net Beans and got it to compile. It worked just fine. What am I doing wrong on the website?
public class Main {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
String answerToLife = "42";
Integer answer;
answer = Integer.parseInt(answerToLife);
System.out.print(answer);
}
}
Output
JAVA_HOME="C:\Program Files\Java\jdk1.8.0_301" cd G:\My Drive\Documents\NetBeansProjects\gradleproject1; .\gradlew.bat --configure-on-demand -x check run Configuration on demand is an incubating feature.
Task :compileJava UP-TO-DATE Task :processResources NO-SOURCE Task :classes UP-TO-DATE
Task :run 42 BUILD SUCCESSFUL in 741ms 2 actionable tasks: 1 executed, 1 up-to-date
But Tree House gives me an error.
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package gradleproject1;
/**
*
* @author dmorl
*/
public class Main {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
String answerToLife = "42";
Integer answer;
answer = Integer.parseInt(answerToLife);
System.out.print(answer);
}
}
2 Answers

jb30
44,806 PointsThe challenge is not expecting you to create a class. You only need to declare the variable answer
and set its value.
In your code, answer
is an Integer
rather than an int
. To make it an int
variable, you could change Integer answer;
to int answer;
.

Sharina Jones
Courses Plus Student 2,502 PointsTo create a variable with the integer data type, you use a lowercase int. If you use Integer, you are creating an Integer object, rather than a primitive variable.
public static void main(String[] args) {
String answerToLife = "42";
int answer;
answer = Integer.parseInt(answerToLife);
System.out.print(answer);
}