Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial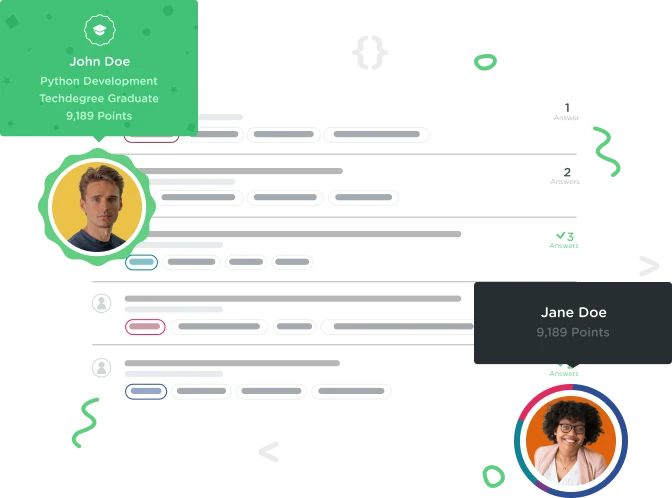

Taylor Facen
Full Stack JavaScript Techdegree Student 15,441 PointsTypeError: app.use() requires middleware functions
For some reason, I'm getting this error even though it looks like my code matches the instructor's.
app.js
const express = require('express');
const bodyParser = require('body-parser');
const cookieParser = require('cookie-parser');
const app = express();
app.use(bodyParser.urlencoded({extended: false}));
app.use(cookieParser());
// tells express which template engine to use
app.set('view engine', 'pug');
const routes = require('./routes');
app.use(routes);
app.use((req, res, next) => {
console.log('Hello');
next();
});
app.use((req, res, next) => {
console.log("World");
next();
});
app.use((req, res, next) => {
const err = new Error('Not Found');
err.status = 404;
next(err);
});
app.use((err, req, res, next) => {
res.locals.error = err;
res.status(err.status);
res.render('error');
});
app.listen(3000, () => {
console.log('The application is running on localhost 3000');
});
index.js
const express = require('express');
const router = express.Router();
router.get('/', (req, res) => {
const name = req.cookies.username;
if (name) {
res.render('index', {name});
} else {
res.redirect('/hello');
}
});
router.get('/cards', (req, res) => {
// another way to do it
//res.locals.prompt = "Who is buried in Grant's tomb?"
res.render('card', {prompt: "Who is buried in Grant's tomb?"});
});
router.get('/hello', (req, res) => {
const name = req.cookies.username;
if (name) {
res.redirect('/');
} else {
res.render('hello');
}
});
router.post('/hello', (req, res) => {
res.cookie('username', req.body.username);
res.redirect('/');
});
router.post('/goodbye', (req, res) => {
res.clearCookie('username');
res.redirect('/hello')
});
module.experts = router;
Please help.
2 Answers
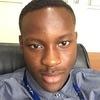
Osaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsIn the last line of your index.js file. You typed 'module.experts' instead of 'module.exports'.

Taylor Facen
Full Stack JavaScript Techdegree Student 15,441 PointsAhhh thank you Osaro Igbinovia ! I'm always messing up on typos. Do you have any advice on how to overcome this?
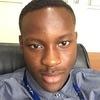
Osaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsTake your time when typing lines of code and quickly review whatever you type before going on.
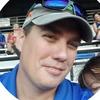
Ryan Emslie
Full Stack JavaScript Techdegree Student 14,453 PointsDon't feel bad. I had the exact same error.