Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial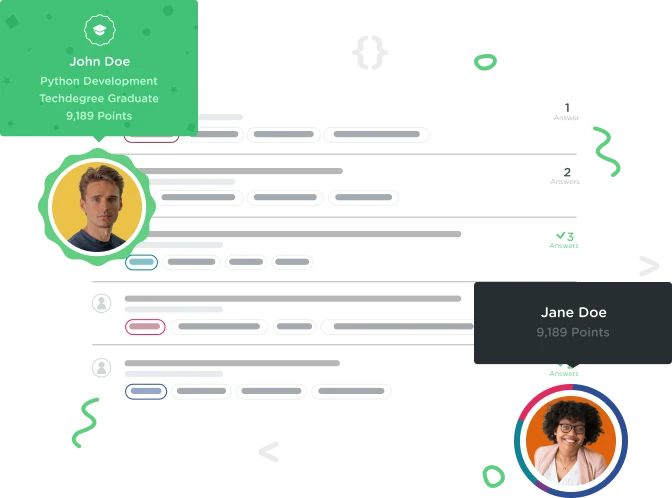
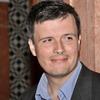
Brian Patterson
19,588 PointsTypeError: Cannot read property 'length' of undefined
I am trying to get some practice with using Node.js . The problem I am having is parsing the data as I am getting the error above. Below is my code.
const http = require('http');
const season = 1971;
function printMessage(races, season) {
const message = `There are ${season} rounds at the ${races}.`;
console.log(message);
}
//printMessage(22,2017);
//Connecting to the API url (http://ergast.com/api/f1/2012.json)
const request = http.get(`http://ergast.com/api/f1/${season}.json`,response => {
//console.dir(response.statusCode);
let body = "";
//Read the data
response.on('data', data => {
//Changing the buffer into a string.
body += data;
});
response.on('end', () => {
//Parse the data
const seasonF1 = JSON.parse(body);
// console.log(body);
// console.log(typeof body);
//console.dir(seasonF1);
printMessage(season, seasonF1.Races.length);
//Print the data
});
});
Below is the error I am getting in the console.
/Users/briankaty1/Dropbox/JavaScript/testApp/app.js:26
printMessage(season, seasonF1.Races.length);
^
TypeError: Cannot read property 'length' of undefined
at IncomingMessage.response.on (/Users/briankaty1/Dropbox/JavaScript/testApp/app.js:26:40)
at emitNone (events.js:91:20)
at IncomingMessage.emit (events.js:185:7)
at endReadableNT (_stream_readable.js:974:12)
at _combinedTickCallback (internal/process/next_tick.js:74:11)
at process._tickCallback (internal/process/next_tick.js:98:9)
Any help would be appreciated.
2 Answers

andren
28,558 PointsThe issue is that there is no property named "Races" at the root of the JSON that you get from that URL.
Here is the parsed JSON:
{
MRData: {
xmlns: "http://ergast.com/mrd/1.4",
series: "f1",
url: "http://ergast.com/api/f1/1971.json",
limit: "30",
offset: "0",
total: "11",
RaceTable: {
season: "1971",
Races: [...]
}
}
}
As you can see the Races
array is found within an object called RaceTable
, which itself is found within MRData
.
If you change your print statement to reflect that structure like this:
printMessage(season, seasonF1.MRData.RaceTable.Races.length);
Then your code should work.
EDIT: Added a view of the JSON you receive.
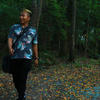
Tuan Phan
10,825 PointsI used [] instead of . to access the value in array.
Works well for me.
My case:
printMessage(profile['name'], profile['badges'].length, profile['points']['JavaScript']);
Yours maybe work with this
printMessage( season, seasonF1['Races'].length );
Brian Patterson
19,588 PointsBrian Patterson
19,588 PointsThank you andren.