Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial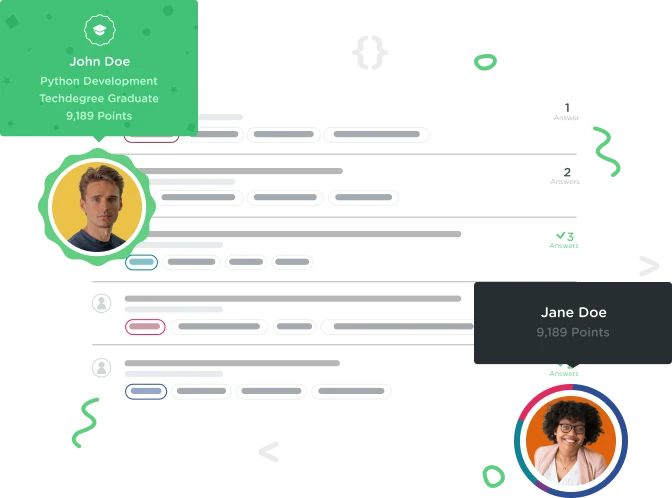

Timothy Grindall
7,843 PointsTypeError: Cannot read property 'map' of undefined
So I followed the videos and checked and rechecked my code for errors but I can find none. When you click on the checkbox I get an error saying "TypeError: Cannot read property 'map' of undefined". The error is in App.js on line 26 which says "guests: this.state.quests.map((guest, index) => {". I've included the relevant files below for examination:
App.js:
import React, { Component } from 'react';
import './App.css';
import GuestList from './GuestList';
class App extends Component {
state = {
guests: [
{
name: 'Treasure',
isConfirmed: false,
},
{
name: 'Nic',
isConfirmed: true,
},
{
name: 'Matt K',
isConfirmed: false,
}
]
}
toggleConfirmationAt = indexToChange =>
this.setState({
guests: this.state.quests.map((guest, index) => {
if (index === indexToChange) {
return {
...guest,
isConfirmed: !guest.isConfirmed
};
}
return guest;
})
});
getTotalInvited = () => this.state.guests.length;
// get AttendingGuests = () =>
// getUnconfirmedGuests = () =>
render() {
return (
<div className="App">
<header>
<h1>RSVP</h1>
<p>A Treehouse App</p>
<form>
<input type="text" value="Safia" placeholder="Invite Someone" />
<button type="submit" name="submit" value="submit">Submit</button>
</form>
</header>
<div className="main">
<div>
<h2>Invitees</h2>
<label>
<input type="checkbox" /> Hide those who haven't responded
</label>
</div>
<table className="counter">
<tbody>
<tr>
<td>Attending:</td>
<td>2</td>
</tr>
<tr>
<td>Unconfirmed:</td>
<td>1</td>
</tr>
<tr>
<td>Total:</td>
<td>3</td>
</tr>
</tbody>
</table>
<GuestList
guests={this.state.guests}
toggleConfirmationAt={this.toggleConfirmationAt}
/>
</div>
</div>
);
}
}
export default App;
GuestList.js:
import React from 'react';
import PropTypes from 'prop-types';
import Guest from './Guest';
const GuestList = props =>
<ul>
{props.guests.map((guest, index) =>
<Guest
key={index}
name={guest.name}
isConfirmed={guest.isConfirmed}
handleConfirmation={() => props.toggleConfirmationAt(index)} />
)}
</ul>;
GuestList.propTypes = {
guests: PropTypes.array.isRequired,
toggleConfirmationAt: PropTypes.func.isRequired
}
export default GuestList;
Guest.js:
import React from 'react';
import PropTypes from 'prop-types';
const Guest = props =>
<li>
<span>{props.name}</span>
<label>
<input
type="checkbox"
checked={props.isConfirmed}
onChange={props.handleConfirmation} /> Confirmed
</label>
<button>edit</button>
<button>remove</button>
</li>
Guest.propTypes = {
name: PropTypes.string.isRequired,
isConfirmed: PropTypes.bool.isRequired,
handleConfirmation: PropTypes.func.isRequired
}
export default Guest;
It renders fine before you click on the checkbox.
3 Answers

Timothy Grindall
7,843 PointsI found the answer: it's a typo. "quests" is supposed to be "guests" so when I changed that it worked.
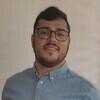
Daniel Benisti
Front End Web Development Techdegree Graduate 22,224 Pointsfirst of all you need to import App to the file with the map function.
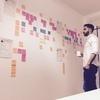
hombah
Python Web Development Techdegree Student 16,241 PointsCannot read property 'map' of undefined" generally means the javascript interpreter is trying to map over some data but the data isn't there. Try to figure out whats going on in the quests array
hombah
Python Web Development Techdegree Student 16,241 Pointshombah
Python Web Development Techdegree Student 16,241 PointsAwesome