Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial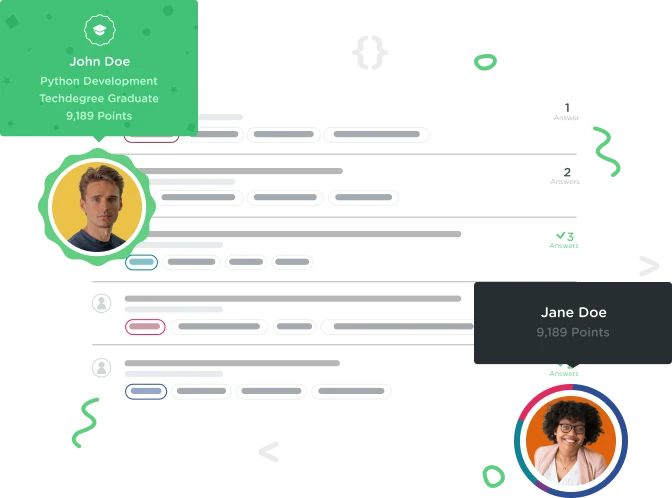
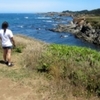
Nancy Melucci
Courses Plus Student 35,157 PointsTypeError: Cannot read property 'signIn' of undefined at UserSignUp.js:94 Not Found error on submit.
I've been working through this project and referring to the final version only as necessary. After struggling through an error with the submit function (hard to interpret) I got the signup page to work but now I am getting the error above in the console. It's as if this page thinks that I am signing a user who is already created in and not signing up a new user. After 20 minutes of trying to figure it out, I went to the final version and replaced the code. So this is the code from the Final version for UserSignUp and I don't understand why it's not working...I look at line 94 and except for the fact that name is missing (tried adding it,no change in error) I am stumped.
import React, { Component } from 'react';
import { Link } from 'react-router-dom';
import Form from './Form';
export default class UserSignUp extends Component {
state = {
name: '',
username: '',
password: '',
errors: [],
}
render() {
const {
name,
username,
password,
errors,
} = this.state;
return (
<div className="bounds">
<div className="grid-33 centered signin">
<h1>Sign Up</h1>
<Form
cancel={this.cancel}
errors={errors}
submit={this.submit}
submitButtonText="Sign Up"
elements={() => (
<React.Fragment>
<input
id="name"
name="name"
type="text"
value={name}
onChange={this.change}
placeholder="Name" />
<input
id="username"
name="username"
type="text"
value={username}
onChange={this.change}
placeholder="User Name" />
<input
id="password"
name="password"
type="password"
value={password}
onChange={this.change}
placeholder="Password" />
</React.Fragment>
)} />
<p>
Already have a user account? <Link to="/signin">Click here</Link> to sign in!
</p>
</div>
</div>
);
}
change = (event) => {
const name = event.target.name;
const value = event.target.value;
this.setState(() => {
return {
[name]: value
};
});
}
submit = () => {
const { context } = this.props;
const {
name,
username,
password,
} = this.state;
// Create user
const user = {
name,
username,
password,
};
context.data.createUser(user)
.then( errors => {
if (errors.length) {
this.setState({ errors });
} else {
context.actions.signIn(username, password)
.then(() => {
this.props.history.push('/authenticated');
});
}
})
.catch((err) => {
console.log(err);
this.props.history.push('/error');
});
}
cancel = () => {
this.props.history.push('/');
}
}
1 Answer
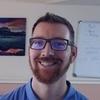
Jason Ziegler
Full Stack JavaScript Techdegree Student 36,760 PointsNancy Melucci I ran into the exact same problem and for me it was that I did not add the following to the Context.js file in the render right above the return block previ(this is instructed in the https://teamtreehouse.com/library/react-authentication/set-up-user-registration)
const value = {
data: this.data,
};