Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial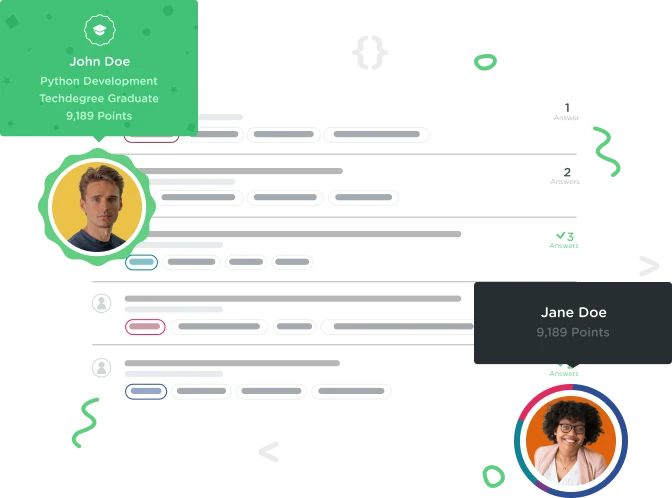

Ty Green
2,062 PointsTypeError: __init__() got an unexpected keyword argument 'sides'
I'm getting an typerror and not sure how to fix it. This is the code...
import random
class Die: def init(self, sides=2, value=0): if not sides >= 2: raise ValueError("Must have at least 2 sides") if not isinstance(sides, int): raise ValueError("Sides must be a whole number") self.value = value or random.randint(1, sides)
def init(self): return self.value
def eq(self, other): return int(self) == other
def ne(self, other): return int(self) != other
def gt(self, other): return int(self) > other
def lt(self, other): return int(self) < other
def ge(self, other): return int(self) > other or int(self) == other
def le(self, other): return int(self) < other or int(self) == other
def add(self, other): return int(self) + other
def radd(self, other): return int(self) + other
class D6(Die): def init(self, value=0): super().init(sides=6, value=value)
This is the error message...
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/home/treehouse/workspace/yatzy/dice.py", line 40, in init
super().init(sides=6, value=value)
TypeError: init() got an unexpected keyword argument 'sides'
Any suggestions? Thanks in advance.
2 Answers
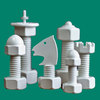
Steven Parker
231,271 PointsI can't test it without the snapshot, but looks like you might need to pass normal arguments in the "super" call:
class D6(Die):
def __init__(self, value=0):
super().__init__(6, value)

Ty Green
2,062 PointsThanks, Steven. I've updated the code but a new type error occurs...
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/home/treehouse/workspace/yatzy/dice.py", line 41, in init
super().init(6, value)
TypeError: init() takes 1 positional argument but 3 were given
import random
class Die:
def __init__(self, sides=2, value=0):
if not sides >= 2:
raise ValueError("Must have at least 2 sides")
if not isinstance(sides, int):
raise ValueError("Sides must be a whole number")
self.value = value or random.randint(1, sides)
def __init__(self):
return self.value
def __eq__(self, other):
return int(self) == other
def __ne__(self, other):
return int(self) != other
def __gt__(self, other):
return int(self) > other
def __lt__(self, other):
return int(self) < other
def __ge__(self, other):
return int(self) > other or int(self) == other
def __le__(self, other):
return int(self) < other or int(self) == other
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return int(self) + other
class D6(Die):
def __init__(self, value=0):
super().__init__(6, value)
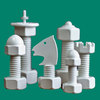
Steven Parker
231,271 PointsMuch easier to read with formatting! So there seems to be two "__init__" methods in the "Die" class, the first takes arguments but the second one does not. And since it comes later, it wipes out the first one.
I'm guessing that 2nd one was meant to be "__int__" (for integer conversion) instead of "__init__" (for instance construction).

Ty Green
2,062 PointsThanks, Steven. That works beautifully!
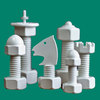
Steven Parker
231,271 PointsTy Green — Glad to help. You can mark a question solved by choosing a "best answer".
And happy coding!
Steven Parker
231,271 PointsSteven Parker
231,271 PointsWhen posting code to the forum, use Markdown formatting to preserve the appearance, or (even better) share the entire workspace by making a snapshot and posting the link to it.