Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial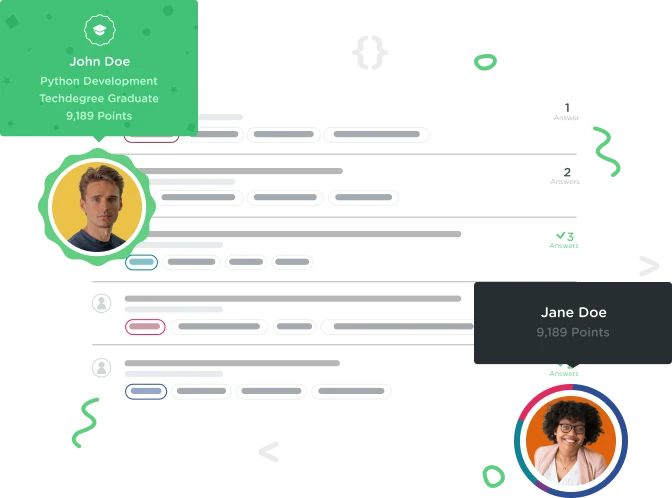

Denis Chernenko
4,077 PointsTypeError: 'list' object is not callable
# -*- coding: utf-8 -*-
"""
Created on Fri Aug 11 12:11:06 2017
@author: User
"""
import datetime
import random
from questions import Add, Multiply
class Quiz:
questions = []
answers = []
correct = []
def __init__(self):
question_types = (Add, Multiply)
#generate 10 random questions
for _ in range(5):
num1 = random.randint(1,50)
num2 = random.randint(1,50)
question = random.choice(question_types)(num1,num2)
#add this questions to self.questions
self.questions.append(question)
def take_quiz(self):
#log the start time
self.start_time = datetime.datetime.now()
#ask all the questions
for question in self.questions:
self.answers.append(self.ask(question))
else:
self.end_time = datetime.datetime.now()
#log if they got the questions right
#log the end time
#show the summary
return self.summary()
def ask(self, question):
correct = 0
question_start = datetime.datetime.now()
#log the start time
answer = input(question.text + " = ")
#capture the answer
#check the answer
if answer == str(question.answer):
correct +=1
#log the end time
question_end = datetime.datetime.now()
#if the answer's right, send back True
#otherwise False
#send back the elapsed time too
return correct, question_end - question_start
def total_correct(self):
#return total number of correct answers
total = 0
for answer in self.answers:
if answer[0]:
total+=1
return total
def summary(self):
#print how many you got right and the total# of questions. 5/10
print("You've got {} from {} right".format(self.correct(), len(self.questions)))
#print the total time for quiz: 30 seconds!
print ("It took you {} seconds total".format(self.end_time - self.start_time).seconds)
Quiz().take_quiz()
#print (smth.questions[0].text)
#print (smth.ques tions[0].answer)
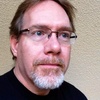
Chris Freeman
Treehouse Moderator 68,423 PointsCan provide complete details on the error message. It usually include the line number where the error occurred. Thanks!

Denis Chernenko
4,077 PointsAfter 5 tries, i've an error TypeError: 'list' object is not callable. Please see below:
47 * 2 = 94
22 + 13 = 33
44 * 21 = 4
16 * 11 = 4
43 + 10 = 4
Traceback (most recent call last):
File "<ipython-input-1-e0dc703efda7>", line 71, in <module>
Quiz().take_quiz()
File "<ipython-input-1-e0dc703efda7>", line 38, in take_quiz
return self.summary()
File "<ipython-input-1-e0dc703efda7>", line 66, in summary
print("You've got {} from {} right".format(self.correct(), len(self.questions)))
TypeError: 'list' object is not callable
1 Answer
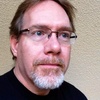
Chris Freeman
Treehouse Moderator 68,423 PointsRemove the parens after self.correct
. It is a list and with parens, the code is trying to call the list as a function. But as the error says 'list' object is not callable.
print("You've got {} from {} right".format(self.correct, len(self.questions)))
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsAdded ```python code formatting