Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial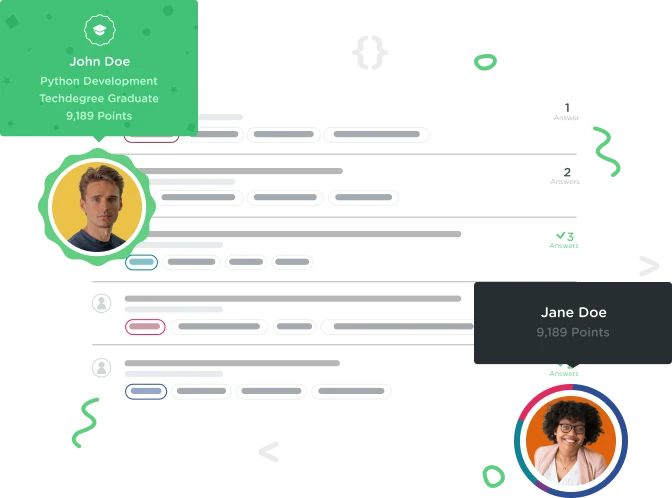

Prateek Sharan Lall
1,778 PointsTypeError: '<' not supported between instances of 'D6' and 'D6'
While running, I am getting this error. Please help, I cannot find what did I do wrong. Thanks.
from dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
class YatzyHand(Hand):
def __init__(self, *args, **kwargs):
super().__init__(size=5, die_class=D6, *args, **kwargs)
2 Answers
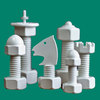
Steven Parker
241,807 PointsChris's suggestion will work, but in the video, a "__lt__
" method is implemented in the "Die" class, which is the base (parent) class of D6. So it's not necessary to re-implement it in D6.
But check your "Die" implementation (in "dice.py", not shown here) in case that method was overlooked or has an error.
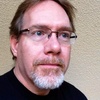
Chris Freeman
Treehouse Moderator 68,460 PointsThe TypeError
issue is being raised by the line self.sort()
in Hand.__init__
. The list.sort
method needs a way to compare two items. The default method is to use the less than <
operator. This requires the __lt__
method to be present in each object contained in the list
. There are two choices:
Add comparisons __lt__
, __gt__
method to the D6 class
def __lt__(self, other):
return int(self) < other
def __gt__(self, other):
return int(self) > other
def __repr__(self):
"""Allow seeing value instead of object description"""
return str(int(self))
Use key=
parameter to the sort
method:
self.sort(key=lambda x: x.value)
Using the key argument says "Use this function, which returns the attribute item.value
(an int
), when comparing items ".
Post back if you need more help. Good luck!!
Chris Freeman
Treehouse Moderator 68,460 PointsChris Freeman
Treehouse Moderator 68,460 PointsGood points. The OP error message implied that
__lt__
wasn't found in the local code, so I assumed it needed to be added in some class. I agree thatDie
is a better location to add this instead ofD6
.Additionally,
__lt__
may not be sufficient unless theother
argument is handled directly.If you get the message TypeError: '<' not supported between instances of 'int' and 'D6' (notice this is between
int
andD6
and not twoD6
instances) then the__gt__
method is needed when theD6
is on the right-hand side of the less than comparison.