Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial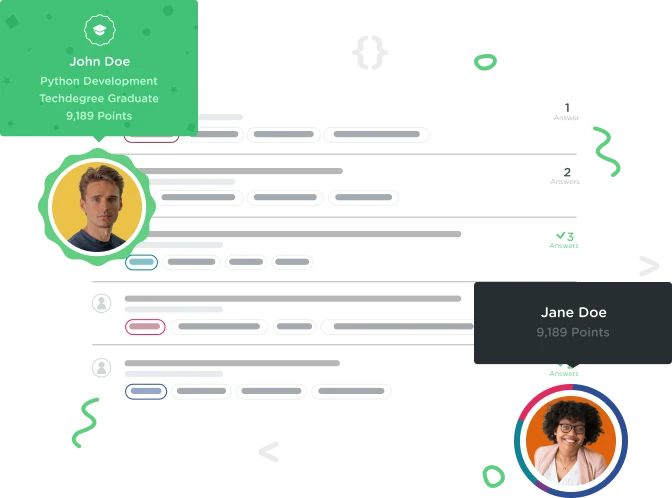
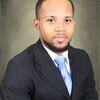
Matthew Bradshaw
7,329 PointsTypeError: openai.createChatCompletion is not a function
Getting this error whenever I try to run the code from the video.
require('dotenv').config(); const { Client, IntentsBitField } = require('discord.js'); OpenAI = require('openai');
const client = new Client({ intents: [ IntentsBitField.Flags.Guilds, IntentsBitField.Flags.GuildMessages, IntentsBitField.Flags.MessageContent, ] })
client.on('ready', () => { console.log('ChatGPT is online'); })
const openai = new OpenAI({ apiKey: process.env.API });
client.on('messageCreate', async (msg) => { if(msg.author.bot) return; if(msg.channel.id !== process.env.CHANNEL) return; if(msg.content.startsWith('!')) return;
let conversationLog = [{
role: 'system',
content: 'You are a friendly chatbot'
}]
await msg.channel.sendTyping();
let previousMessages = await msg.channel.messages.fetch({ limit: 15 });
previousMessages.reverse();
previousMessages.forEach((message) => {
if(msg.content.startsWith('!')) return;
if(message.author.id !== client.user.id && msg.author.bot) return;
if(message.author.id !== msg.author.id) return;
conversationLog.push({
role: 'user',
content: message.content,
})
})
const res = await openai.createChatCompletion({
model: 'gpt-3.5-turbo',
messages: conversationLog,
});
msg.reply(res.data.choices[0].message);
});
client.login(process.env.TOKEN);
1 Answer
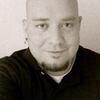
Brian Jensen
Treehouse StaffHiya Matthew Bradshaw! 👋 Our apologies for the issues that you are running into!
Like many aspects of technology, software packages are often updated frequently. Consequently, a method or feature that functions properly in one version may become obsolete or altered in subsequent releases. This appears to be the scenario with the OpenAI Node.js library, where certain functionalities have seemingly been deprecated or changed following the v4 update. Luckily, there is a handy v3 to v4 migration guide if you want to follow along with that.
Another option would be to run npm uninstall openai
in the terminal, then run npm i openai@3.3.0
to specify the final version of v3, which will match the code in the video.