Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial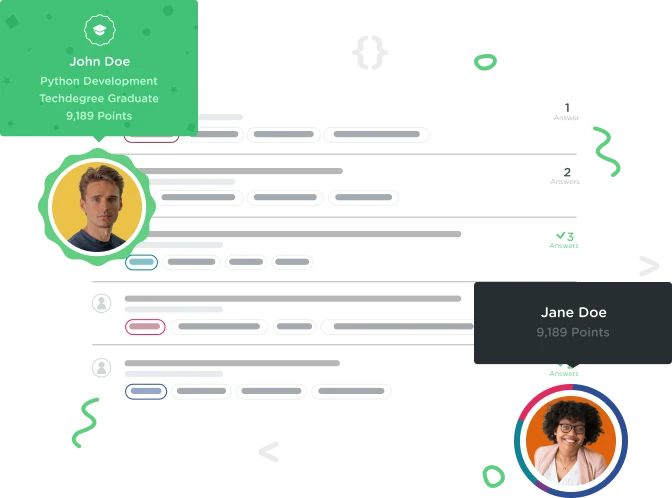

jamesflint00
42,676 PointsTypeError: __str__() missing 1 required positional argument: 'pattern'
No sure what is going on here but when I run this in an IDE I get the following Traceback TypeError: str() missing 1 required positional argument: 'pattern'
But when I put the print in the parent class init it prints the pattern, not sure why it isn't going in the parent class init?
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self, pattern):
for index, value in enumerate(pattern):
if value == ".":
pattern[index] = "dot"
elif value == "_":
pattern[index] = "dash"
else:
continue
return "-".join(pattern)
class S(Letter):
def __init__(self):
pattern = ['.', '_', '.']
super().__init__(pattern)
1 Answer
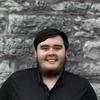
Michael Hulet
47,913 Pointsprint
takes any kind of object as a parameter, but you can only print strings to the console, so that means the print
function has to convert whatever it takes to a string. It does this by calling __str__
on the object it's passed, which it expects to return the object in string form. The __str__
method on all objects should generally shouldn't take any required arguments at the call site, but in your implementation of it, you're requiring that whoever calls __str__
on your Letter
class (or any of its subclasses) pass it a parameter pattern
. The people who wrote Python's print
function don't know about your class, so they just call __str__
without parameters. In other words, print
is calling __str__
on your object like this:
stringified = your_object.__str__()
But you're requiring it to call it by doing something like this:
stringified = your_object.__str__([".", "_", "."])
You're not gonna be able to talk to the developers of Python and get them to change the language to call __str__
like you want it to, but you can tell Python to not worry about that one extra parameter you're asking for. You do this by setting it to have a default value, like you did in Letter
's __init__
, where you set the pattern
object to have a default value of None
. If you do something like that on the __str__
method, print
will be able to convert your object to a string without your program crashing
jamesflint00
42,676 Pointsjamesflint00
42,676 PointsThanks Michael