Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial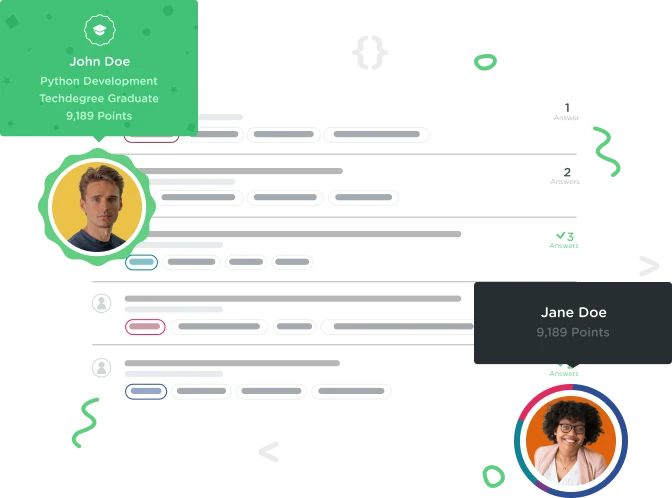

Dan B
6,155 PointsTypeError: super() takes at least 1 argument (0 given)
I'm getting this weird error even though I'm absolutely sure that the code I've written is the same as Kenneth's.
The error:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "inheritance.py", line 17, in __init__
super().__init__(name, **kwargs)
TypeError: super() takes at least 1 argument (0 given)
My code:
import random
# This is a 'parent' class to which other classes can inherit attributes
class Character:
def __init__(self, name, **kwargs):
self.name = name
for key, value in kwargs.items():
setattr(self, key, value)
# Thief is inheriting the init and dictionary (kwargs) attributes from character
class Thief(Character):
sneaky = True
def __init__(self, name, sneaky=True, **kwargs):
# When we use super() we have to include the method name and the required argument(s)
super().__init__(name, **kwargs)
self.sneaky = sneaky
def pickpocket(self):
return self.sneaky and bool(random.randint(0, 1))
def hide(self, light_level):
return self.sneaky and light_level < 10
I'm trying to run:
mark = Thief("Mark", sneaky=False, clever=True)
So the exact same code that Kenneth is running, except he doesn't get an error and I have. I've spent 20 minutes looking at my code but can't understand why it's spitting out this error. I have passed in an argument to super() as far as I can see I passed name at the very least.
P.S. If anyone could tell me how to set python syntax highlighting here in Treehouse that would be great. The Markdown Chatsheet just redirects to the forums.
1 Answer

Dan B
6,155 PointsI just realised I'm using the REPL for Python 2.7.16 to run this...
Python 2.7.16 (default, Oct 10 2019, 22:02:15)
[GCC 8.3.0] on linux2
Type "help", "copyright", "credits" or "license" for more information.
>>> from inheritance import Thief
>>> mark = Thief("Mark", sneaky=False, clever=True)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "inheritance.py", line 17, in __init__
super().__init__(name, **kwargs)
TypeError: super() takes at least 1 argument (0 given)
Running this with python3 works fine.
But I would like to know, does anyone know what changed between Python 2.7.16 and Python 3.7.3 that makes this work? (If it isn't too much to explain)
boi
14,242 Pointsboi
14,242 PointsFor python formatting,
``` python
your code here
```