Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial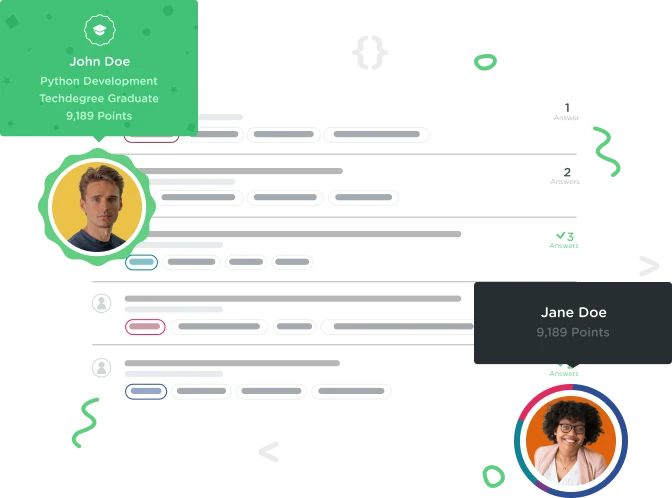
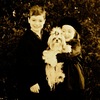
Bradley Maravalli
8,927 PointsTyping out this code has given me and others difficulties w/ parentheses, brackets, etc. So here is the completed code.
I and others have had difficulties in typing out this code for the React - Decomposing our Application lesson. Too many parentheses, brackets, and more that, if typed out incorrectly, leads us to have to deal with a bunch of errors.
Here is the full code that I recommend you USE THIS AS A COMPARISON rather than code to copy & paste.
function Header(props) {
return (
<div className="header">
<h1>{props.title}</h1>
</div>
);
}
Header.propTypes = {
title: React.PropTypes.string.isRequired,
};
function Counter(props) {
return (
<div className="counter">
<button className="counter-action decrement"> - </button>
<div className="counter-score"> {props.score} </div>
<button className="counter-action increment"> + </button>
</div>
);
}
Counter.propTypes = {
score: React.PropTypes.number.isRequired,
};
function Player(props) {
return (
<div className="player">
<div className="player-name">
{props.name}
</div>
<div className="player-score">
<Counter score={props.score} />
</div>
</div>
);
}
Player.propTypes = {
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired,
};
function Application(props) {
return (
<div className="scoreboard">
<Header title={props.title} />
<div className="players">
<Player name="Jim Hoskins" score={31} />
<Player name="Andrew Chalkley" score={33} />
</div>
</div>
);
}
Application.propTypes = {
title: React.PropTypes.string,
};
Application.defaultProps = {
title: "Scoreboard",
}
ReactDOM.render(<Application />, document.getElementById('container'));
Steven Parker
231,269 PointsSteven Parker
231,269 PointsRegardless of the intent, I don't think pasteable solutions are a good idea. What might make more sense would be a few example lines from areas that typically cause trouble (assuming you've identified them).
Also, how might someone associate this post with something from one of the courses?