Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial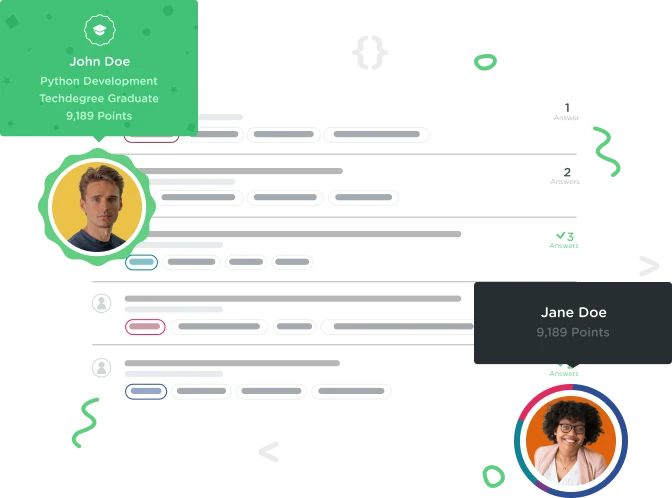

Luke Haddad
6,082 PointsUgh, I've tried to figure this out, and I'm not sure what this challenge exactly wants me to do....so frustrating...
//This initiates the program, prompting a message to the user.
console.log("Begin program");
alert("Welcome, welcome! Are you lucky enough?");
//This prompts the user with questions indicating they should fill in numbers and await their random number response.
var questionOne = prompt("Please enter your first number below.");
var integerOne = parseInt(questionOne);
var questionTwo = prompt("Please enter your second number below.");
var integerTwo = parseInt(questionTwo);
/*This is where I've expanded the original number generator, and allowed it to give the user a random number between designated numbers I've put in the 'document.write'.
I've also made sure that only the values are numbers, and will spit out an error message if it isn't.*/
function findErrors(two, nine) {
if (isNaN(nine) === true || true === isNaN(one))
{throw new Error('Wrong! Make sure it\'s a number.');}
else
}
function random(integerTwo, integerOne)
{if (integerOne > integerTwo)
{return (Math.floor(Math.random() * (integerTwo - integerOne + 1)) + integerOne);
document.write(random);}
else if (integerOne < integerTwo)
{return (Math.floor(Math.random() * (integerOne - integerTwo + 1)) + integerTwo);
document.write(random);}
else
{alert("Nope, sorry. Try again.");}}
document.write("<p>Here's your first lucky number:</p>");
document.write(random(10, 2));
document.write("<p>Here's your second lucky number:</p>");
document.write(random(100, 20));
document.write("<p>Here's your third lucky number:</p>");
document.write(random('nine hundred', 'two hundred'));
document.write("<p>Play again?</p>");
//This is the end of program.
console.log("End program");
1 Answer
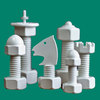
Steven Parker
229,783 PointsIf you watch the next video, the instructor shows you a solution that you can compare to.
But for starters, here's a few observations I made with your code:
- when testing, you don't need to check values against "true", just naming the value does that
- giving variables number names is not illegal, but it can be very confusing
- in your findErrors function, be sure that you are working with the same variables that are passed in
- you have to call your findErrors function at some point (like inside "random") for it to work
- you don't need an else if you don't do anything different when the test does not pass
Luke Haddad
6,082 PointsLuke Haddad
6,082 PointsThis challenge is a bit confusing to understand (I attempted to it in "function findErrors"). I don't know....I would appreciate a little help in the right direction?