Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial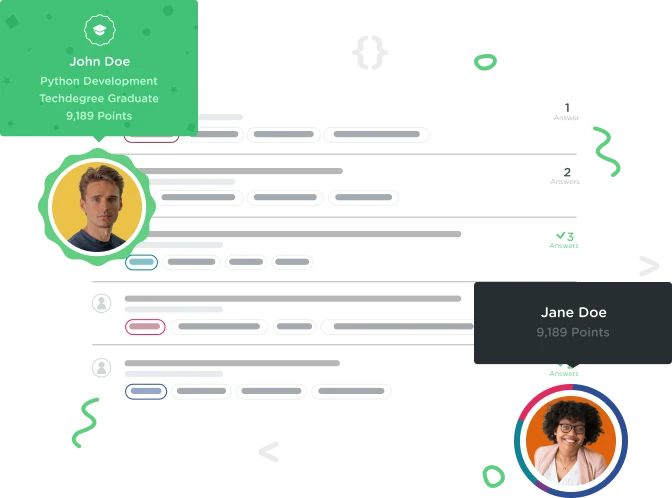

Jonah Kesoyan
2,055 PointsUICollectionView and Parse
If you have photos stored in Parse, how can you place them in a UICollectionView?
2 Answers

muqbilhashi
1,498 PointsUICollectionView is similar to UITableView. If you are not familiar with UICollectionViewController, I suggest you spend sometime reading and understanding UICollectionViewController. If you are familiar with UICollectionViewController, share what you have done so far so we can better help you.

muqbilhashi
1,498 Points- (void)viewDidLoad {
[super viewDidLoad];
[self getPhotosFromParse];
}
- (void)getPhotosFromParse {
PFQuery *query = [PFQuery queryWithClassName:@"Photos"];
[query findObjectsInBackgroundWithBlock:^(NSArray *objects, NSError *error) {
if (error) {
NSLog(@"Error %@ %@", error, [error userInfo]);
}
else
{
self.photos = objects;
}
}];
}
-(UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath {
PhotoCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:@"pic" forIndexPath:indexPath];
UIImage *myImage = [UIImage imageNamed:@"PlaceholderPhoto.png"];
PFImageView *imageView = [[PFImageView alloc] init];
imageView.image = myImage;
cell.myImageView.image = imageView.image;
PFFile *pImage = [self.photos objectAtIndex:indexPath.row] ;
imageView.file = pImage;
[imageView loadInBackground:^(UIImage *image, NSError *error) {
if (!error) {
cell.myImageView.image = image;
}
}];
return cell;
}
This is rough draft.. I have not tested. This is just for retrieving images from parse and displaying placeholder image briefly while the image is loading in the background. hope this helps
Jonah Kesoyan
2,055 PointsJonah Kesoyan
2,055 PointsTHIS IS THE CLASS ASSOCIATED WITH UICOLLECTIONVIEWCONTROLLER, HOW WOULD I GET THE PHOTOS FROM PARSE IN THE COLLECTION VIEW?