Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial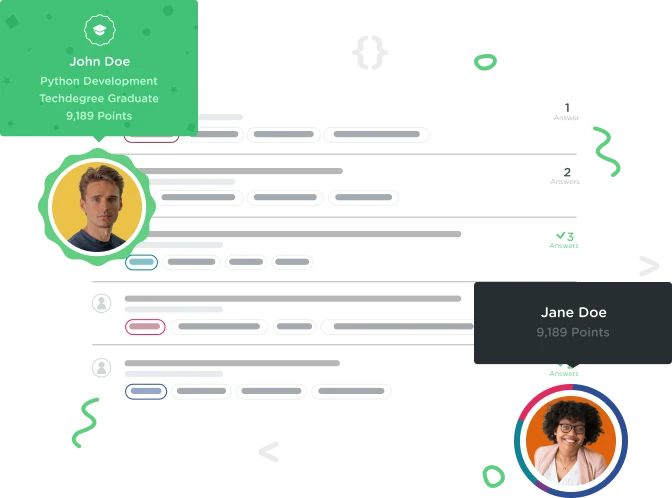

Connor Palindat
1,735 PointsUIColor Array?
Hi,
I'm trying to do the extra credit for the data collection track, but when I run this code, I get an error talking about threads? I already deleted the breakpoints and I am still getting the error.
- (void)viewDidLoad
{
[super viewDidLoad];
self.predictions = [[NSArray alloc] initWithObjects:
@"It is Certain",@"It is decidely so",
@"All signs say YES",@"The stars are not aligned",
@"My reply is no",@"It is doubtful",
@"Better not tell you now",
@"Concentrate and ask again",
@"Unable to answer now",
nil];
self.textColor = [[NSArray alloc] initWithObjects: [UIColor blueColor], [UIColor redColor], nil];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (IBAction)buttonPressed {
int randomColor = arc4random_uniform(self.textColor.count);
int random = arc4random_uniform(self.predictions.count);
self.predictionLabel.text = [self.predictions objectAtIndex:random];
self.predictionLabel.textColor = [self.predictions objectAtIndex:1];
}
@end
3 Answers

kerdeseverin
9,688 PointsIf you look at your fix, you're now assigning self.predictionLabel.textColor to a UIColor. Previously you were assigning it to a NSString.
self.predictionLabel.textColor = [self.predictions objectAtIndex:1];
where self.predictions was:
self.predictions = [[NSArray alloc] initWithObjects:
@"It is Certain",@"It is decidely so",
@"All signs say YES",@"The stars are not aligned",
@"My reply is no",@"It is doubtful",
@"Better not tell you now",
@"Concentrate and ask again",
@"Unable to answer now",
nil];
Your predictions array only had strings. This is why it was not working. So your current fix will work, or you can use your previous code by changing to
[self. textColor objectAtIndex:random];

Connor Palindat
1,735 PointsNope :( It still doesn't work even with that change.

kerdeseverin
9,688 PointsTake a look at it again. Where are you using the self.textColor property?
self.predictionLabel.text = [self.predictions objectAtIndex:random];
self.predictionLabel.textColor = [self.predictions objectAtIndex:1]; // <--- here
You're setting the textColor of the preditionlabel to a NString.

Connor Palindat
1,735 PointsI...kind of get what you mean but still not 100%. I did figure out a fix where I did this:
- (IBAction)buttonPressed {
int random = arc4random_uniform(self.predictions.count);
int randomColorIdx = arc4random_uniform(colors.count);
NSArray *colors = @[[UIColor redColor], [UIColor blueColor]];
UIColor *randomColor = [colors objectAtIndex:randomColorIdx];
self.predictionLabel.textColor = randomColor;
self.predictionLabel.text = [self.predictions objectAtIndex:random];
}
I just think this isn't as efficient as the memory for the colors array would be created and destroyed everytime the button is pressed right? Im just having a hard time using self and making this into a property.