Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial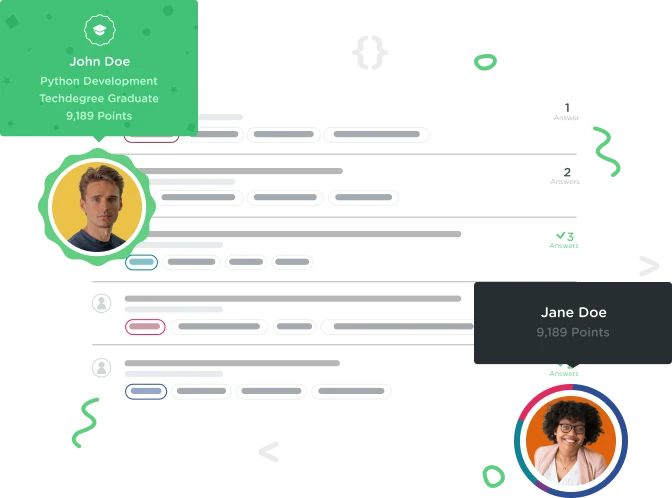

Matthew Chess
13,860 PointsUiTabBar Swipe to Delete a row
I wanted to add a feature to the Ribbit app that a user could swipe a row to delete a contact. Could someone guide me what to look for in the documentation?
2 Answers
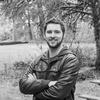
Ryan Ackermann
14,665 PointsThis is a great idea for a feature to add!
After a little work messing around, and adding some cheeky animations I came up with the following:
#pragma mark - Swipe to delete
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath {
return YES;
}
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath {
if (editingStyle == UITableViewCellEditingStyleDelete) {
PFUser *userToDelete = [self.friends objectAtIndex:indexPath.row];
NSLog(@"Deleting User: %@", userToDelete.username);
[self.friendsRelation removeObject:userToDelete];
[[PFUser currentUser] saveInBackground];
[self.friends removeObjectAtIndex:indexPath.row];
NSRange range = NSMakeRange(0, 1);
NSIndexSet *sectionToReload = [NSIndexSet indexSetWithIndexesInRange:range];
[tableView reloadSections:sectionToReload withRowAnimation:UITableViewRowAnimationFade];
}
}
In order to make this all work you need to change the friends property from an NSArray to an NSMutableArray to efficiently remove and reload the tableview.
Link to the Apple Doc for UITableViewDataSource
Hope this helps dude! :)

Matthew Chess
13,860 Points. I had thought apple would have a method for this to mimic their other apps but guess not. Thanks man that helps a lot
Imran Mouna
2,504 PointsImran Mouna
2,504 PointsAwesome thank you! If it help anyone else when I switched the friends NSArray to an NSMutable Array I had change the following in viewWillAppear:
self.friends = objects
To
self.friends = [objects mutableCopy];