Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial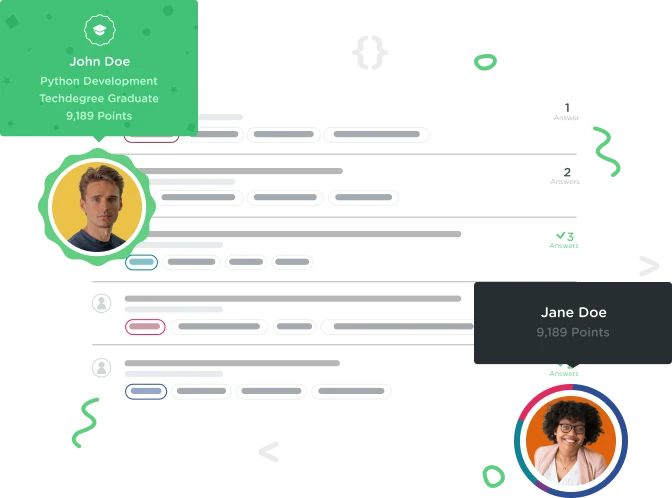

habtezion dawit
1,918 Pointsunable to complete challenge, i keep getting the same error message
why does it keep showing me the same compiler error saying that " Bummer! Oops, look like there is a compiler error! It could be that you forgot to include the proper import statements. Click Preview to see the exact compiler message." my code looks correct
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> allAuthors = new TreeSet<String>
for(BlogPost post : mPost) {
allAuthors.add(post.getAuthors());
}
return allAuthors;
}
}
3 Answers

andren
28,558 PointsIt keeps giving you an error because there are errors, mostly typos which can be incredibly hard to spot while just skimming over the code, that is why you are being recommended to look at the preview page that displays errors, those point out the places where Java is encountering an error, which make it far easier to notice where a typo has been made.
Rather than just providing the answer, I'll first give you some troubleshooting tips that will hopefully help you solve issues like this by yourself in the future, being able to understand compiler error messages and using them as a guide to fix your code is somewhat of an essential skill for a programmer, especially in the beginning where you'll likely be making a lot of typos that can be hard to catch in any other way.
I'll go through the method I used to troubleshoot your code, which is also how I tend to troubleshoot my own code.
First try to run the code and then press the "Preview" button to look at the compiler errors that have been produced, there are a lot of errors that pop up when your code is run but often times a single mistake can lead to a lot of errors popping up as a result. So let's concentrate on the first error for now, as the first error is often the thing causing a lot of the other errors:
./com/example/Blog.java:20: error: '(' or '[' expected
for(BlogPost post : mPost) {
^
That tells us that it was expecting but did not find a parentheses or a bracket somewhere around line 20 of the Blog.java file, looking at that area we can see this code:
Set<String> allAuthors = new TreeSet<String>
for(BlogPost post : mPost) {
allAuthors.add(post.getAuthors());
}
While the for loop (which Java was pointing at) looks correct, the line directly above it does not, it is missing a set of parentheses and a semicolon.
Fixing that one error and trying to run the code now only returns two errors, those being:
./com/example/Blog.java:20: error: cannot find symbol
for(BlogPost post : mPost) {
^
./com/example/Blog.java:21: error: cannot find symbol
allAuthors.add(post.getAuthors());
^
"cannot find symbol" is Java's way of saying that one of the names referenced is unrecognized, which often means that there is a typo in the name.
Looking closely at those lines and comparing the names to the names found elsewhere in the code it becomes clear that the words with errors are: "mPost" which should be "mPosts", and "getAuthors()" which should be "getAuthor()"
Fixing those two typos and running the code now result in the code running properly and the challenge succeeds.
Here is the fixed code for reference:
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> allAuthors = new TreeSet<String>();
for(BlogPost post : mPosts) {
allAuthors.add(post.getAuthor());
}
return allAuthors;
}
}

Ken Alger
Treehouse TeacherHabtezion;
You are close. There are some syntax errors in your code, but you have the basic idea I think. Double check a few things like spelling (mPosts
instead of mPost
for example), semi-colons, and correct function names and assignments.
Post back if you are still stuck.
Happy coding,
Ken

habtezion dawit
1,918 Pointsthanks andren, understanding the nuances of my mistakes will help me in the long run!