Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial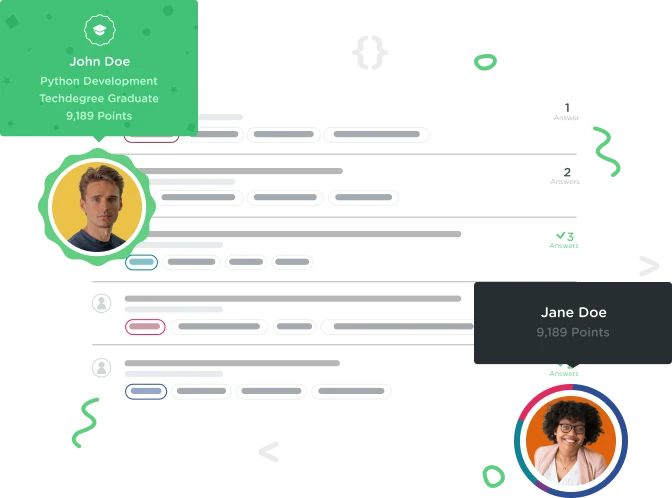

miia
13,198 PointsUnable to create requested service, Unable to load class
I got this error:
kesäk. 04, 2019 8:29:26 AP. org.hibernate.Version logVersion
INFO: HHH000412: Hibernate Core {6.0.0.Alpha2}
kesäk. 04, 2019 8:29:26 AP. org.hibernate.cfg.Environment <clinit>
INFO: HHH000206: hibernate.properties not found
kesäk. 04, 2019 8:29:27 AP. org.hibernate.annotations.common.reflection.java.JavaReflectionManager <clinit>
INFO: HCANN000001: Hibernate Commons Annotations {5.1.0.Final}
kesäk. 04, 2019 8:29:27 AP. org.hibernate.engine.jdbc.connections.internal.DriverManagerConnectionProviderImpl configure
WARN: HHH10001002: Using Hibernate built-in connection pool (not for production use!)
Exception in thread "main" java.lang.ExceptionInInitializerError
Caused by: org.hibernate.service.spi.ServiceException: Unable to create requested service [org.hibernate.engine.jdbc.env.spi.JdbcEnvironment]
at org.hibernate.service.internal.AbstractServiceRegistryImpl.createService(AbstractServiceRegistryImpl.java:275)
at org.hibernate.service.internal.AbstractServiceRegistryImpl.initializeService(AbstractServiceRegistryImpl.java:237)
at org.hibernate.service.internal.AbstractServiceRegistryImpl.getService(AbstractServiceRegistryImpl.java:214)
at org.hibernate.boot.model.relational.Database.<init>(Database.java:41)
at org.hibernate.boot.internal.InFlightMetadataCollectorImpl.getDatabase(InFlightMetadataCollectorImpl.java:216)
at org.hibernate.boot.internal.InFlightMetadataCollectorImpl.<init>(InFlightMetadataCollectorImpl.java:187)
at org.hibernate.boot.model.process.spi.MetadataBuildingProcess.complete(MetadataBuildingProcess.java:123)
at org.hibernate.boot.model.process.spi.MetadataBuildingProcess.build(MetadataBuildingProcess.java:88)
at org.hibernate.boot.internal.MetadataBuilderImpl.build(MetadataBuilderImpl.java:401)
at org.hibernate.boot.internal.MetadataBuilderImpl.build(MetadataBuilderImpl.java:79)
at org.hibernate.boot.MetadataSources.buildMetadata(MetadataSources.java:190)
at contactmgr.Application.buildSessionFactory(Application.java:33)
at contactmgr.Application.<clinit>(Application.java:19)
Caused by: org.hibernate.boot.registry.classloading.spi.ClassLoadingException: Unable to load class [org.h2.Driver]
at org.hibernate.boot.registry.classloading.internal.ClassLoaderServiceImpl.classForName(ClassLoaderServiceImpl.java:136)
at org.hibernate.engine.jdbc.connections.internal.DriverManagerConnectionProviderImpl.loadDriverIfPossible(DriverManagerConnectionProviderImpl.java:149)
at org.hibernate.engine.jdbc.connections.internal.DriverManagerConnectionProviderImpl.buildCreator(DriverManagerConnectionProviderImpl.java:105)
at org.hibernate.engine.jdbc.connections.internal.DriverManagerConnectionProviderImpl.buildPool(DriverManagerConnectionProviderImpl.java:89)
at org.hibernate.engine.jdbc.connections.internal.DriverManagerConnectionProviderImpl.configure(DriverManagerConnectionProviderImpl.java:73)
at org.hibernate.boot.registry.internal.StandardServiceRegistryImpl.configureService(StandardServiceRegistryImpl.java:100)
at org.hibernate.service.internal.AbstractServiceRegistryImpl.initializeService(AbstractServiceRegistryImpl.java:246)
at org.hibernate.service.internal.AbstractServiceRegistryImpl.getService(AbstractServiceRegistryImpl.java:214)
at org.hibernate.engine.jdbc.env.internal.JdbcEnvironmentInitiator.buildJdbcConnectionAccess(JdbcEnvironmentInitiator.java:178)
at org.hibernate.engine.jdbc.env.internal.JdbcEnvironmentInitiator.initiateService(JdbcEnvironmentInitiator.java:102)
at org.hibernate.engine.jdbc.env.internal.JdbcEnvironmentInitiator.initiateService(JdbcEnvironmentInitiator.java:35)
at org.hibernate.boot.registry.internal.StandardServiceRegistryImpl.initiateService(StandardServiceRegistryImpl.java:94)
at org.hibernate.service.internal.AbstractServiceRegistryImpl.createService(AbstractServiceRegistryImpl.java:263)
... 12 more
Caused by: java.lang.ClassNotFoundException: Could not load requested class : org.h2.Driver
at org.hibernate.boot.registry.classloading.internal.AggregatedClassLoader.findClass(AggregatedClassLoader.java:210)
at java.base/java.lang.ClassLoader.loadClass(ClassLoader.java:588)
at java.base/java.lang.ClassLoader.loadClass(ClassLoader.java:521)
at java.base/java.lang.Class.forName0(Native Method)
at java.base/java.lang.Class.forName(Class.java:415)
at org.hibernate.boot.registry.classloading.internal.ClassLoaderServiceImpl.classForName(ClassLoaderServiceImpl.java:133)
... 24 more
Here is my hibernate.cfg.xml file:
<?xml version='1.0' encoding='utf-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- Database connection setting -->
<property name="connection.driver_class">org.h2.Driver</property>
<property name="connection.url">jdbc:h2:./data/contactmgr</property>
<!-- SQL dialect -->
<property name="dialect">org.hibernate.dialect.H2Dialect"</property>
<!-- Create the database schema on startup -->
<property name="hbm2ddl.auto">create</property>
<!-- Show the queries prepared by Hibernate -->
<property name="show_sql">true</property>
<!-- Names the annotated entity classes -->
<mapping class="contactmgr.model.Contact" />
</session-factory>
</hibernate-configuration>
And here is my Application.java:
package contactmgr;
import contactmgr.model.Contact;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.boot.MetadataSources;
import org.hibernate.boot.registry.StandardServiceRegistryBuilder;
import org.hibernate.service.ServiceRegistry;
public class Application {
// Hold a reusable reference to a SessionFactory (since we need only one)
private static final SessionFactory sessionFactory = buildSessionFactory();
private static SessionFactory buildSessionFactory() {
// Create a StandardServiceRegistry
final ServiceRegistry registry = new StandardServiceRegistryBuilder().configure().build();
return new MetadataSources(registry).buildMetadata().buildSessionFactory();
}
public static void main(String[] args) {
Contact contact = new Contact.ContactBuilder("x", "x")
.withEmail("x@x.x")
.withPhone(1234567L)
.build();
// Open a session
Session session = sessionFactory.openSession();
// Begin a transaction
session.beginTransaction();
// Use the session to save the contact
session.save(contact);
// Commit the transaction
session.getTransaction().commit();
// Close the session
session.close();
}
}
And Contact.java:
package contactmgr.model;
import javax.persistence.*;
@Entity
public class Contact {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
@Column
private String firstName;
@Column
private String lastName;
@Column
private String email;
@Column
private Long phone;
// Default constructor for JPA
public Contact() {}
public Contact(ContactBuilder builder) {
this.firstName = builder.firstName;
this.lastName = builder.lastName;
this.email = builder.email;
this.phone = builder.phone;
}
@Override
public String toString() {
return "Contact{" +
"id=" + id +
", firstName='" + firstName + '\'' +
", lastName='" + lastName + '\'' +
", email='" + email + '\'' +
", phone=" + phone +
'}';
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Long getPhone() {
return phone;
}
public void setPhone(Long phone) {
this.phone = phone;
}
public static class ContactBuilder {
private String firstName;
private String lastName;
private String email;
private Long phone;
public ContactBuilder(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public ContactBuilder withEmail(String email) {
this.email = email;
return this;
}
public ContactBuilder withPhone(Long phone) {
this.phone = phone;
return this;
}
public Contact build(){
return new Contact(this);
}
}
}
And dependencies file:
plugins {
id 'java'
}
version '1.0-SNAPSHOT'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
dependencies {
compile group: 'org.hibernate.orm', name: 'hibernate-core', version: '6.0.0.Alpha2'
testCompile group: 'com.h2database', name: 'h2', version: '1.4.199'
compile group: 'javax.transaction', name: 'jta', version: '1.1'
compile group: 'javax.xml.bind', name: 'jaxb-api', version: '2.4.0-b180830.0359'
compile group: 'com.sun.xml.bind', name: 'jaxb-impl', version: '2.4.0-b180830.0438'
compile group: 'com.sun.xml.bind', name: 'jaxb-core', version: '2.3.0.1'
compile group: 'javax.activation', name: 'activation', version: '1.1.1'
compile group: 'org.olap4j', name: 'olap4j', version: '1.2.0'
}
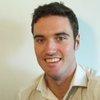
Cameron Stewart
18,041 PointsMiia, did you find a solution?
1 Answer

Pablo Wedemann Colchiesqui
9,187 Pointsfound the error, change "testCompile" in gradle to "compile". Worked for me.
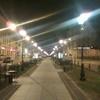
Alexander Zola
9,027 Pointsor change H2 testimplementation to implementation in build gradle
Pablo Wedemann Colchiesqui
9,187 PointsPablo Wedemann Colchiesqui
9,187 PointsI'm having the exact same problem, did you find a solution?