Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial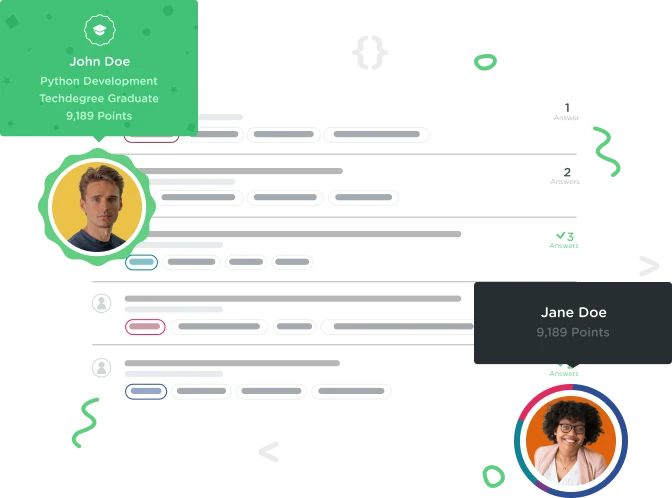

kellycurtis
3,716 PointsUnable to figure out what I am missing for this challenge after various attempts.
Define a constructor on the Fish class with 3 parameters, in order, named $name, $flavor, and $record.
<?php
class Fish {
public $common_name; public $flavor; public $record_weight;
function_construct($name, $flavor, $record) {
} } ?>
<?php
class Fish {
public $common_name;
public $flavor;
public $record_weight;
function_construct($name, $flavor, $record) {
}
}
?>
4 Answers
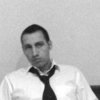
Alex Heil
53,547 Pointshey Kelly Curtis , from the logic / syntax your code and the one provided by @jasonanders is absolutely fine. having that said the reason why your code currently doesn't pass is that the properties defined in the class don't match inside the constructor function. instead of common_name you currently use name and instead of record_weight you use record - these technically don't exist ;)
the function instead should read like this:
<?php
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
?>
hope that helps and have a nice day ;)
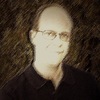
Jason Anders
Treehouse Moderator 145,860 PointsHi Kelly, You are close. First off, you are missing one under-score before "construct." Also you need to assign the seudo variable and the property name inside the function part of the class.
<?php
class Fish
{
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record){
$this->name = $name;
$this->flavor = $flavor;
$this->record = $record;
}
}
?>
I hope this makes sense and helps you. Keep Coding! :)

kellycurtis
3,716 PointsYes! Thank you! I did do the pseudo, but it still errored out so I thought it was wrong. It was the 2nd underscore I was missing. I made it harder than it needed to be. Thanks for the help!!!
Kelly

kellycurtis
3,716 PointsActually that didn't work though it makes the most sense. These challenges are sometimes too strict since it is automated checking. Any other ideas??
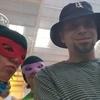
Robbie Thomas
31,093 PointsAlex Heli's code works in this one.
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsThanks Alex.
It's weird though, I don't copy/paste code into the forum until I make sure it passes the challenge question. But, you're right, it doesn't pass, because the properties don't match. Not sure how I let that slip by? :)
kellycurtis
3,716 Pointskellycurtis
3,716 PointsI tried it that way too and it didn't work. Tried it again just in case. I can usually figure these out, but this one is giving me a run for my money. This is the last one I tried after trying yours above.
<?php
class Fish {
public $common_name; public $flavor; public $record_weight;
function __construct($name, $flavor, $record){ $this->common_name = $name; $this->flavor = $flavor; $this->record_weight = $record; } ?>
Alex Heil
53,547 PointsAlex Heil
53,547 Pointshey Kelly Curtis , the code you just pasted is missing the very last closing curly bracket. I copied your code, added the curly bracket and the challenge passes just fine.
here's the whole correct code in a block:
hope that helps ;)
kellycurtis
3,716 Pointskellycurtis
3,716 PointsThanks for all your help! And I couldn't comment on your last answer, but this will count. It would figure I would miss a curly brace. I think I was up way too long and this is a new language for me as I learned RoR first. I'm cramming as I was offered a position with this as the language.
Thanks for all your help!!!