Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial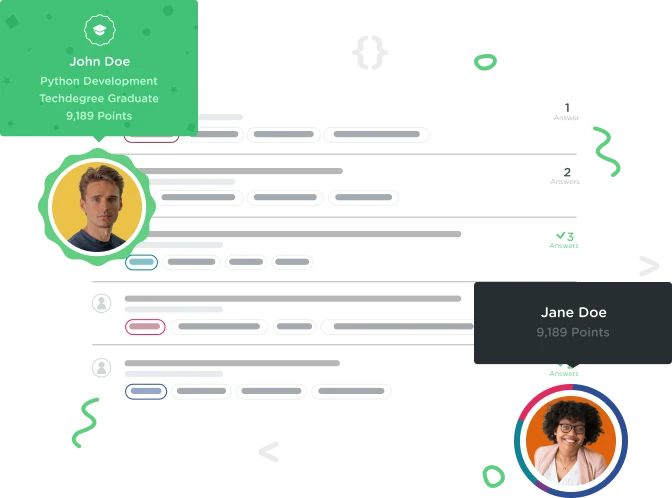

Georg Ekeberg
5,702 PointsUnable to find the right solution by testing for an error thrown
Here is my code. It says that you tried with something that should pass, but it failed. I have tried a lot of different things.
I get this message: Bummer: We tried your spec with a version of subtraction.js that DOESN'T work correctly, expecting the test to fail. But it passed! Better try again!
var expect = require('chai').expect
describe('subtraction', function () {
var subtraction = require('../WHEREVER')
it('only works with numbers', function () {
const errorMsg = "subtraction only works with numbers!"
const handler0 = () => subtraction("one",2);
const handler1 = () => subtraction(1, "two");
const handler2 = () => subtraction("one", "two");
const handlerB0 = () => subtraction(true,2);
const handlerB1 = () => subtraction(1, true);
const handlerB2 = () => subtraction(true, true);
const handlerA0 = () => subtraction([1],2);
const handlerA1 = () => subtraction(1, [2]);
const handlerA2 = () => subtraction([1], [2]);
const handlerO0 = () => subtraction({b:1},2);
const handlerO1 = () => subtraction(1, {b:2});
const handlerO2 = () => subtraction({b:1}, {b:2});
const handlerx = () => subtraction(1,2);
expect(handler0).to.throw(errorMsg);
expect(handler1).to.throw(errorMsg);
expect(handler2).to.throw(errorMsg);
expect(handlerB0).to.throw(errorMsg);
expect(handlerB1).to.throw(errorMsg);
expect(handlerB2).to.throw(errorMsg);
expect(handlerA0).to.throw(errorMsg);
expect(handlerA1).to.throw(errorMsg);
expect(handlerA2).to.throw(errorMsg);
expect(handlerO0).to.throw(errorMsg);
expect(handlerO1).to.throw(errorMsg);
expect(handlerO2).to.throw(errorMsg);
expect(handlerx).to.not.throw(errorMsg);
})
})
function subtraction (number1, number2) {
if (typeof number1 !== 'number' || typeof number2 !== 'number') {
throw Error('subtraction only works with numbers!')
}
return number1 - number2
}
1 Answer
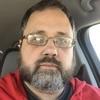
Mark Sebeck
Treehouse Moderator 38,304 PointsI did get it to pass if I write the function like Guil did
const handler0 = function() { subtraction("number",2); };
Georg Ekeberg
5,702 PointsGeorg Ekeberg
5,702 PointsThank you! I guess it could not handle arrow functions. Was no problem locally.
Mark Sebeck
Treehouse Moderator 38,304 PointsMark Sebeck
Treehouse Moderator 38,304 PointsYou're welcome. Glad you did it locally with arrow functions. I admire you trying! Keep at it.