Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial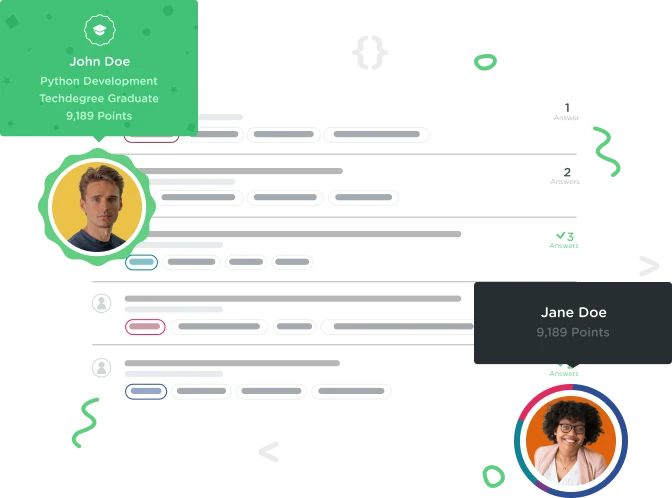
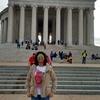
Purvi Agrawal
7,960 PointsUnable to get it through !
Around line 17, create a function named 'arrayCounter' that takes in a parameter which is an array. The function must return the length of an array passed in or 0 if a 'string', 'number' or 'undefined' value is passed in. Important: The code you write in each task should be added to the code written in the previous task.
1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <title>JavaScript Foundations: Functions</title> 5 <style> 6 html { 7 background: #FAFAFA; 8 font-family: sans-serif; 9 } 10 </style> 11 </head> 12 <body> 13 <h1>JavaScript Foundations</h1> 14 <h2>Functions: Return Values</h2> 15 <script> 16
17
18 ? 19 </script> 20 </body> 21 </html>
6 Answers
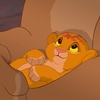
Laura Cressman
12,548 PointsThe idea here is to define a function with one parameter, then check to see if the "type of" the parameter is a string, number, or undefined. If it is, the function should return 0. If it isn't ("else"), the function should return the parameter's length by calling the length method. I am not sure what your code looks like, but this should work:
function arrayCounter (list) {
if (typeof list == "string" || typeof list == "number" || typeof list == "undefined") {
return 0;
} else {
return list.length;
}
}
Let me know if that helps :)
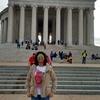
Purvi Agrawal
7,960 PointsThank You so much .... :) It helped a lot !!
I was just not able to understand the question. Your description was so perfect .
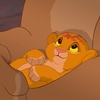
Laura Cressman
12,548 PointsYou're welcome!
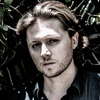
Richard Nash
24,862 PointsHi Laura, what are the tall vertical lines for? They have not been introduced in the course up to this point. Also, why can i not put "string" "number" and "undefined" together? Also, why would I want to return a 0 for a 'string' or a 'number'? That would seem to be what I would be looking for inside of most arrays...
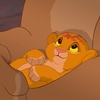
Laura Cressman
12,548 PointsHi Richard, The vertical lines are Javascript for "or", so just like && means and, || means or. As far as I know, the syntax doesn't let you just type commas between them, so we need three separate statements to check. Lastly, the parameter of our function is an array, since our function involves counting how many items are in an array. For example, if we have an array called animals (["dog", "cat", "fish"]), our function should return 3 since there are three things in it. If we call arrayCounter(animals), since animals is an array, we should get three. We want to make sure that we return 0 if someone gives the function a parameter other than an array, like arrayCounter("a string"), or arrayCounter(32), since these are not arrays and therefore don't have a number of items. Strings and numbers can certainly be IN the arrays we pass the function, but we must pass the function an array, not just an individual string or number, which is why we have our long if statement at the beginning. Does that help? :)
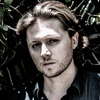
Richard Nash
24,862 PointsThank you for the reply Laura. It's all starting to come together slowly and make more and more sense as i do more and more of it. I must say, though, that programming is not as hard as i thought it would be at all... at least not at this stage :-)
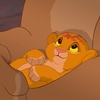
Laura Cressman
12,548 PointsGlad to hear it's making sense for you :)
Errin E. Johnson
22,458 PointsI now get the || = or, in regards to understanding the problem now,(thank YOU) also, what im am hearing from Laura is that the problem is asking to pass an array(as in, definition), more than 1 item, where as ||or|| can result in only 1 item therefore the name array vs. the function of an array is what confused me, in regards to the question. the "If" statement calls to pass the" || or" and doesn't not call to pass an "array" of [item1,item,2item3]. The "if" statement needs to specify not an array but either || or then return 0; or you will get 3 as in the length of array.length or 1 for arrayCounter.length. I think. :)