Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial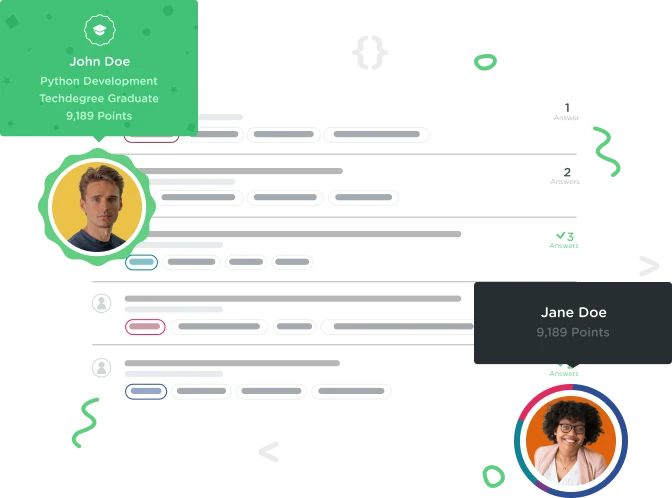

Nathan Stec
Courses Plus Student 1,201 PointsUnable to get the result variable to hold the right value
I cannot get the result variable to hold the correct value of the greeting function. I was supposed to pass the string "Tom" to the greeting function and assign it to the variable result
func greeting(person: String) -> (language:String, greeting:String) {
let language = "English"
let greeting = "Hello \(person)"
var info = (language, greeting)
return info
}
var result = greeting("Tom")
6 Answers

Greg Kaleka
39,021 PointsHi Nathan,
You're very close! All you need to change is the tuple your function is returning; it needs to be a named tuple. It's a bit confusing since the names are the same as the variables, but it should look like this:
func greeting(person: String) -> (language:String, greeting:String) {
let language = "English"
let greeting = "Hello \(person)"
var info = (language: language, greeting: greeting) // I changed this line
return info
}
var result = greeting("Tom")
However, there is a bug in this challenge (cc: Pasan Premaratne). The first challenge asks you to return a tuple with "both the greeting and the language." You're supposed to do this in this order: greeting, language. Importantly (and why I see it as a bug) the first challenge does not enforce this order, but the second challenge does.
So, in order to pass the second challenge, we need to flip the order:
func greeting(person: String) -> (greeting: String, language: String) { //flipped here
let language = "English"
let greeting = "Hello \(person)"
var info = (greeting: greeting, language: language) //and here
return info
}
var result = greeting("Tom")

Brian Holland
3,508 PointsI may be wrong, but should "greeting" not refer to two different things?

Greg Kaleka
39,021 PointsHey Brian - as I said to Anthony, it's probably not a best practice to throw the same word around with different meanings, but in this case they don't actually interfere with each other.

Anthony Sego
7,565 PointsI have not tried iOS coding yet, but I wanted to give answering a question a try. It looks like the issue is your variable is the same as your function. The word "greeting" is the function you're trying to use and the variable as well, which is probably confusing the program. Try using another variable other than "greeting."

Greg Kaleka
39,021 PointsIf I were writing this code from scratch, I would probably avoid this as well. I would also avoid naming my tuple with greeting and language. Now there are three instances of "greeting", each with different meaning... not great. However, there is nothing technically wrong with doing this. The code will work.

Nathan Stec
Courses Plus Student 1,201 PointsI thought that but I cannot change those names because those where the names I was given. I only made up the info variable. I still tried to change the names but it came up with another error saying I needed to change them back

Nathan Stec
Courses Plus Student 1,201 PointsI also plugged the code into swift and it came back with the correct output.

Anthony Sego
7,565 PointsDoes the last line need to be var result = info("Tom") ? I looks like you wrote a function to have a greeting in english, but then after that on the next line did not use the new variable that stated it was English. Just seems like you would want to use your new variable that includes both language and greeting. Again, I have no idea, I'm just throwing ideas out in hopes that it helps because I know it can be frustrating when you get stuck on something.

Nathan Stec
Courses Plus Student 1,201 PointsI tried that as well and that didn't seem to work either. It's really odd, because when I plug it into Xcode it works perfectly and gives the right output so I'm not sure why it is saying its wrong.

Greg Kaleka
39,021 PointsHey Anthony. Since Nathan needs to call the function, he's definitely right to call greeting("Tom")
. There isn't a function in his code, so info("Tom")
would cause a compiler error.
Nathan Stec
Courses Plus Student 1,201 PointsNathan Stec
Courses Plus Student 1,201 PointsThank you!